i have this c code its a bouncing ball game and it has a paddle to make the ball bounce back but it is not working. how to fix it? #include #include #include // Define constants for the screen size and ball velocity. #define SCREEN_WIDTH 60 #define SCREEN_HEIGHT 20 #define BALL_VELOCITY 1 #define CENTER // Variables to keep track of position and speed of ball int x = 0; int y = 100; int x_speed = 5; int y_speed = 5; // Variables to keep track of paddle int x_paddle = 250, y_paddle = 370; int paddle_width_half = 40; // score keeping int score = 0; int width; int key; int LEFT; int RIGHT; int keyPressed; int keyCode; // Called every re-draw, defaul 30 times per second void draw() { // Update position by adding speed x = x + x_speed; y = y + y_speed; if (y < 0) y_speed = -y_speed; if (x > width || x < 0) x_speed = -x_speed; // Check if keys are pressed if (keyPressed) { if (keyCode == RIGHT || key == 'd') { // Move paddle right x_paddle = x_paddle + 8; } else if (keyCode == LEFT || key == 'a') { // Move paddle left x_paddle = x_paddle - 8; } else if (key == ' ') { // Restart x = 0; y = 100; x_paddle = 250; score = 0; } } } int main() { // Initialize the random number generator. srand(time(NULL)); // Initialize the ball position and velocity. int ball_x = SCREEN_WIDTH / 2; int ball_y = 1; int ball_velocity_x = BALL_VELOCITY; int ball_velocity_y = BALL_VELOCITY; // Game loop. while (1) { // Clear the screen. system("cls"); // Draw the ceiling. for (int i = 0; i < SCREEN_WIDTH + 2; i++) { printf("-"); } printf("\n"); // Draw the ball. for (int i = 0; i < ball_y; i++) { printf("\n"); } for (int i = 0; i < ball_x; i++) { if (i == SCREEN_WIDTH / 2 - 3 || i == SCREEN_WIDTH / 2 + 2) { printf(" "); } else { printf(" "); } } printf("O\n"); for (int i = ball_y + 1; i < SCREEN_HEIGHT; i++) { printf("\n"); } for (int i = 0; i < SCREEN_WIDTH + 2; i++) { printf("_"); } printf("\n"); // Update the ball position and velocity. ball_x += ball_velocity_x; ball_y += ball_velocity_y; if (ball_x == 0 || ball_x == SCREEN_WIDTH - 1) { ball_velocity_x = -ball_velocity_x; } if (ball_y == 0 || ball_y == SCREEN_HEIGHT - 1) { ball_velocity_y = -ball_velocity_y; } // Check if the ball goes into the hole on top or bottom and make it come out as two balls. if (ball_x == SCREEN_WIDTH / 2 - 2 && ball_y == 0) { ball_velocity_y = -ball_velocity_y; ball_y += ball_velocity_y; printf("OO\n"); } else if (ball_x == SCREEN_WIDTH / 2 - 2 && ball_y == SCREEN_HEIGHT - 1) { ball_velocity_y = -ball_velocity_y; ball_y += ball_velocity_y; printf("OO\n"); } // Wait for a short time to control the game speed. for (int i = 0; i < 100000000; i++) { // Do nothing. } } return 0; }
Addition of Two Numbers
Adding two numbers in programming is essentially the same as adding two numbers in general arithmetic. A significant difference is that in programming, you need to pay attention to the data type of the variable that will hold the sum of two numbers.
C++
C++ is a general-purpose hybrid language, which supports both OOPs and procedural language designed and developed by Bjarne Stroustrup. It began in 1979 as “C with Classes” at Bell Labs and first appeared in the year 1985 as C++. It is the superset of C programming language, because it uses most of the C code syntax. Due to its hybrid functionality, it used to develop embedded systems, operating systems, web browser, GUI and video games.
i have this c code its a bouncing ball game and it has a paddle to make the ball bounce back but it is not working. how to fix it?
#include <stdio.h>
#include <stdlib.h>
#include <time.h>
// Define constants for the screen size and ball velocity.
#define SCREEN_WIDTH 60
#define SCREEN_HEIGHT 20
#define BALL_VELOCITY 1
#define CENTER
// Variables to keep track of position and speed of ball
int x = 0;
int y = 100;
int x_speed = 5;
int y_speed = 5;
// Variables to keep track of paddle
int x_paddle = 250, y_paddle = 370;
int paddle_width_half = 40;
// score keeping
int score = 0;
int width;
int key;
int LEFT;
int RIGHT;
int keyPressed;
int keyCode;
// Called every re-draw, defaul 30 times per second
void draw() {
// Update position by adding speed
x = x + x_speed;
y = y + y_speed;
if (y < 0)
y_speed = -y_speed;
if (x > width || x < 0)
x_speed = -x_speed;
// Check if keys are pressed
if (keyPressed) {
if (keyCode == RIGHT || key == 'd') {
// Move paddle right
x_paddle = x_paddle + 8;
}
else if (keyCode == LEFT || key == 'a') {
// Move paddle left
x_paddle = x_paddle - 8;
}
else if (key == ' ') {
// Restart
x = 0;
y = 100;
x_paddle = 250;
score = 0;
}
}
}
int main()
{
// Initialize the random number generator.
srand(time(NULL));
// Initialize the ball position and velocity.
int ball_x = SCREEN_WIDTH / 2;
int ball_y = 1;
int ball_velocity_x = BALL_VELOCITY;
int ball_velocity_y = BALL_VELOCITY;
// Game loop.
while (1)
{
// Clear the screen.
system("cls");
// Draw the ceiling.
for (int i = 0; i < SCREEN_WIDTH + 2; i++)
{
printf("-");
}
printf("\n");
// Draw the ball.
for (int i = 0; i < ball_y; i++)
{
printf("\n");
}
for (int i = 0; i < ball_x; i++)
{
if (i == SCREEN_WIDTH / 2 - 3 || i == SCREEN_WIDTH / 2 + 2) {
printf(" ");
}
else {
printf(" ");
}
}
printf("O\n");
for (int i = ball_y + 1; i < SCREEN_HEIGHT; i++)
{
printf("\n");
}
for (int i = 0; i < SCREEN_WIDTH + 2; i++)
{
printf("_");
}
printf("\n");
// Update the ball position and velocity.
ball_x += ball_velocity_x;
ball_y += ball_velocity_y;
if (ball_x == 0 || ball_x == SCREEN_WIDTH - 1)
{
ball_velocity_x = -ball_velocity_x;
}
if (ball_y == 0 || ball_y == SCREEN_HEIGHT - 1)
{
ball_velocity_y = -ball_velocity_y;
}
// Check if the ball goes into the hole on top or bottom and make it come out as two balls.
if (ball_x == SCREEN_WIDTH / 2 - 2 && ball_y == 0) {
ball_velocity_y = -ball_velocity_y;
ball_y += ball_velocity_y;
printf("OO\n");
}
else if (ball_x == SCREEN_WIDTH / 2 - 2 && ball_y == SCREEN_HEIGHT - 1) {
ball_velocity_y = -ball_velocity_y;
ball_y += ball_velocity_y;
printf("OO\n");
}
// Wait for a short time to control the game speed.
for (int i = 0; i < 100000000; i++)
{
// Do nothing.
}
}
return 0;
}

Trending now
This is a popular solution!
Step by step
Solved in 3 steps

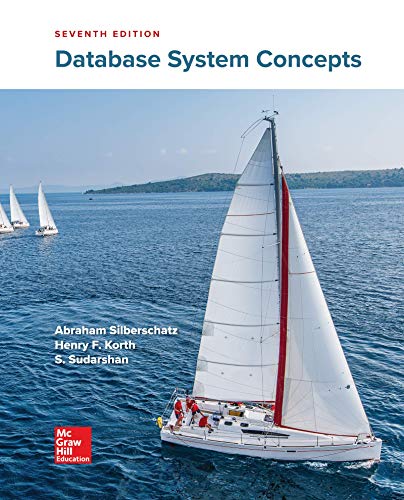
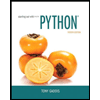
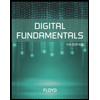
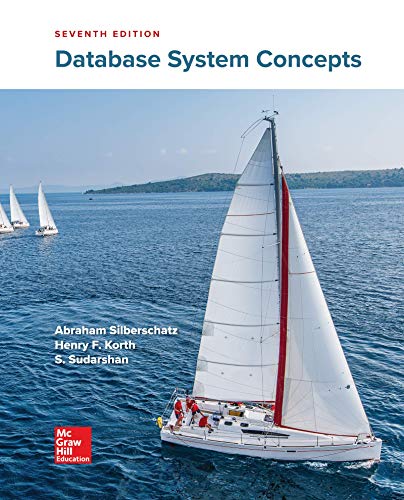
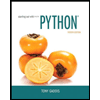
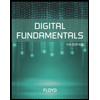
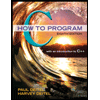
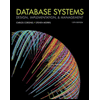
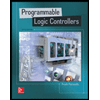