I have the following MIPS assembly code: .data prompt1: .asciiz "Enter integer A: " prompt2: .asciiz "Enter integer B: " result_add: .asciiz "A + B = " result_sub: .asciiz "A - B = " result_and: .asciiz "A & B = " result_or: .asciiz "A | B = " result_xor: .asciiz "A ^ B = " .text # Print prompt and read integer A li $v0, 4 # syscall code for printing string la $a0, prompt1 # load address of prompt1 into $a0 syscall li $v0, 5 # syscall code for reading integer syscall move $t0, $v0 # save integer A in $t0 # Print prompt and read integer B li $v0, 4 # syscall code for printing string la $a0, prompt2 # load address of prompt2 into $a0 syscall li $v0, 5 # syscall code for reading integer syscall move $t1, $v0 # save integer B in $t1 # Calculate and print A + B add $t2, $t0, $t1 li $v0, 1 # syscall code for printing integer move $a0, $t2 # load $t2 into $a0 syscall li $v0, 4 # print newline la $a0, "\n" syscall # Calculate and print A - B sub $t2, $t0, $t1 li $v0, 1 # syscall code for printing integer move $a0, $t2 # load $t2 into $a0 syscall li $v0, 4 # print newline la $a0, "\n" syscall # Calculate and print A & B and $t2, $t0, $t1 li $v0, 1 # syscall code for printing integer move $a0, $t2 # load $t2 into $a0 syscall li $v0, 4 # print newline la $a0, "\n" syscall # Calculate and print A | B or $t2, $t0, $t1 li $v0, 1 # syscall code for printing integer move $a0, $t2 # load $t2 into $a0 syscall li $v0, 4 # print newline la $a0, "\n" syscall # Calculate and print A ^ B xor $t2, $t0, $t1 li $v0, 1 # syscall code for printing integer move $a0, $t2 # load $t2 into $a0 syscall li $v0, 4 # print newline la $a0, "\n" syscall # Exit program li $v0, 10 # syscall code for program exit syscall but it is giving me the following errors: (attached is also the initial instructions)
I have the following MIPS assembly code:
.data
prompt1: .asciiz "Enter integer A: "
prompt2: .asciiz "Enter integer B: "
result_add: .asciiz "A + B = "
result_sub: .asciiz "A - B = "
result_and: .asciiz "A & B = "
result_or: .asciiz "A | B = "
result_xor: .asciiz "A ^ B = "
.text
# Print prompt and read integer A
li $v0, 4 # syscall code for printing string
la $a0, prompt1 # load address of prompt1 into $a0
syscall
li $v0, 5 # syscall code for reading integer
syscall
move $t0, $v0 # save integer A in $t0
# Print prompt and read integer B
li $v0, 4 # syscall code for printing string
la $a0, prompt2 # load address of prompt2 into $a0
syscall
li $v0, 5 # syscall code for reading integer
syscall
move $t1, $v0 # save integer B in $t1
# Calculate and print A + B
add $t2, $t0, $t1
li $v0, 1 # syscall code for printing integer
move $a0, $t2 # load $t2 into $a0
syscall
li $v0, 4 # print newline
la $a0, "\n"
syscall
# Calculate and print A - B
sub $t2, $t0, $t1
li $v0, 1 # syscall code for printing integer
move $a0, $t2 # load $t2 into $a0
syscall
li $v0, 4 # print newline
la $a0, "\n"
syscall
# Calculate and print A & B
and $t2, $t0, $t1
li $v0, 1 # syscall code for printing integer
move $a0, $t2 # load $t2 into $a0
syscall
li $v0, 4 # print newline
la $a0, "\n"
syscall
# Calculate and print A | B
or $t2, $t0, $t1
li $v0, 1 # syscall code for printing integer
move $a0, $t2 # load $t2 into $a0
syscall
li $v0, 4 # print newline
la $a0, "\n"
syscall
# Calculate and print A ^ B
xor $t2, $t0, $t1
li $v0, 1 # syscall code for printing integer
move $a0, $t2 # load $t2 into $a0
syscall
li $v0, 4 # print newline
la $a0, "\n"
syscall
# Exit
li $v0, 10 # syscall code for program exit
syscall
but it is giving me the following errors:
(attached is also the initial instructions)

![spim: (parser) syntax error on line 30 of file /Users/ralucaostoia/Desktop/1/binops.asm
la $a0, "\n"
Instruction references undefined symbol at 0x00400014
[0x00400014] 0x0c000000 jal 0x00000000 [main]
; 188: jal main](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F5ccb13ef-bf62-4bab-95f5-f0fc9e7adadc%2F8c26bd4e-ab60-4b8e-91fd-51ad5783d5be%2Fjxxfrqc_processed.png&w=3840&q=75)

Step by step
Solved in 5 steps with 1 images

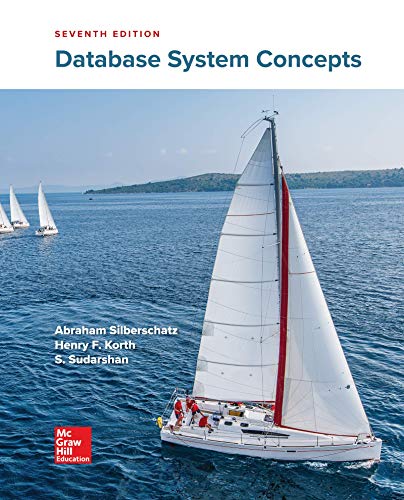
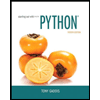
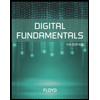
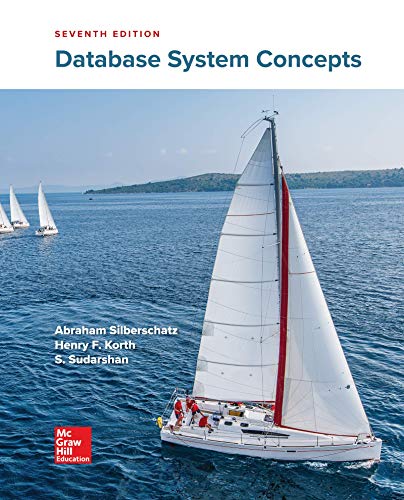
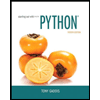
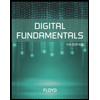
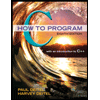
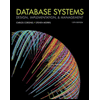
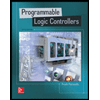