I have a quick question about an assignment with python, I am using data validation to validate that the user input does not go above a set amount, but I am not sure how to pass the variable by reference. Here is the code # Theater Seating Lab # Abisai Saavedra # the main function def main(): # constants COST_A = 20 COST_B = 15 COST_C = 10 A = 300 B = 500 C = 200 programIntro(COST_A,COST_B,COST_C,A,B,C) keepGoing = False while (keepGoing == False): totalA,totalB,totalC = get_section () display_output(totalA,totalB,totalC) keepGoing = get_yes_or_no("Are you finished inputting ticket sales? Type yes or no:") print("Thank you for using the program, program terminating") # brief explanation of program def programIntro(COST_A,COST_B,COST_C,A,B,C): print("Hello, welcome to Seatings Calculator") print("Please follow the system prompts to place number of tickets sold per section") print("After giving the program the proper information, you will get a total of revenue earned.") print("----------------------------") print("Prices:") print(" o Section A Seatings = $", COST_A,"-" ,A, "MAX seats") print(" o Section B Seatings = $", COST_B,"-" ,B, "MAX seats") print(" o Section C Seatings = $", COST_C,"-" ,C, "MAX seats") print("----------------------------") # gathers information submitted by user def get_section (): soldA = get_valid_nbr("How many seats were sold in section A") soldB = get_valid_nbr("How many seats were sold in section B") soldC = get_valid_nbr("How many seats were sold in section C") return soldA,soldB,soldC def display_output(totalA,totalB,totalC): if totalA > 0: print("you have sold", totalA * 20, "worth of seats in section A by selling", totalA) if totalB > 0: print("you have sold", totalB * 15, "worth of seats in section B by selling", totalB) if totalC > 0: print("you have sold", totalC * 10, "worth of seats in section C by selling", totalC) # function that validates user input def get_yes_or_no(message): while True: new_value = get_string(message).lower() if new_value == "y" or new_value == "yes": return True if new_value == "n" or new_value == "no": return False print('Error: invalid value entered') # function that validates user int input def get_valid_nbr(message): new_value = get_integer(message) while is_invalid(new_value): new_value = get_integer("Please enter a number greater than 0") return new_value # function that returns True or False def is_invalid(new_value): if new_value > 300: print("Theater seatings sold cannot be {0}, it must be lesser than or equal to 300".format(new_value)) return True if new_value > 500: print("Theater seatings sold cannot be {0}, it must be lesser than or equal to 500".format(new_value)) return True if new_value > 200: print("Theater seatings sold cannot be {0}, it must be lesser than or equal to 200".format(new_value)) return True if new_value > 0: return False # validation for input def get_integer(message): while True: try: new_value = int(input(message)) return new_value except ValueError: print(' Error: non-integer value entered') def get_string(message): while True: new_value = input(message) if new_value and new_value.strip(): return new_value else: print(' Error: no data entered') # calls main if __name__ == '__main__': main()
I have a quick question about an assignment with python, I am using data validation to validate that the user input does not go above a set amount, but I am not sure how to pass the variable by reference. Here is the code
# Theater Seating Lab
# Abisai Saavedra
# the main function
def main():
# constants
COST_A = 20
COST_B = 15
COST_C = 10
A = 300
B = 500
C = 200
programIntro(COST_A,COST_B,COST_C,A,B,C)
keepGoing = False
while (keepGoing == False):
totalA,totalB,totalC = get_section ()
display_output(totalA,totalB,totalC)
keepGoing = get_yes_or_no("Are you finished inputting ticket sales? Type yes or no:")
print("Thank you for using the
# brief explanation of program
def programIntro(COST_A,COST_B,COST_C,A,B,C):
print("Hello, welcome to Seatings Calculator")
print("Please follow the system prompts to place number of tickets sold per section")
print("After giving the program the proper information, you will get a total of revenue earned.")
print("----------------------------")
print("Prices:")
print(" o Section A Seatings = $", COST_A,"-" ,A, "MAX seats")
print(" o Section B Seatings = $", COST_B,"-" ,B, "MAX seats")
print(" o Section C Seatings = $", COST_C,"-" ,C, "MAX seats")
print("----------------------------")
# gathers information submitted by user
def get_section ():
soldA = get_valid_nbr("How many seats were sold in section A")
soldB = get_valid_nbr("How many seats were sold in section B")
soldC = get_valid_nbr("How many seats were sold in section C")
return soldA,soldB,soldC
def display_output(totalA,totalB,totalC):
if totalA > 0:
print("you have sold", totalA * 20, "worth of seats in section A by selling", totalA)
if totalB > 0:
print("you have sold", totalB * 15, "worth of seats in section B by selling", totalB)
if totalC > 0:
print("you have sold", totalC * 10, "worth of seats in section C by selling", totalC)
# function that validates user input
def get_yes_or_no(message):
while True:
new_value = get_string(message).lower()
if new_value == "y" or new_value == "yes":
return True
if new_value == "n" or new_value == "no":
return False
print('Error: invalid value entered')
# function that validates user int input
def get_valid_nbr(message):
new_value = get_integer(message)
while is_invalid(new_value):
new_value = get_integer("Please enter a number greater than 0")
return new_value
# function that returns True or False
def is_invalid(new_value):
if new_value > 300:
print("Theater seatings sold cannot be {0}, it must be lesser than or equal to 300".format(new_value))
return True
if new_value > 500:
print("Theater seatings sold cannot be {0}, it must be lesser than or equal to 500".format(new_value))
return True
if new_value > 200:
print("Theater seatings sold cannot be {0}, it must be lesser than or equal to 200".format(new_value))
return True
if new_value > 0:
return False
# validation for input
def get_integer(message):
while True:
try:
new_value = int(input(message))
return new_value
except ValueError:
print(' Error: non-integer value entered')
def get_string(message):
while True:
new_value = input(message)
if new_value and new_value.strip():
return new_value
else:
print(' Error: no data entered')
# calls main
if __name__ == '__main__':
main()

Step by step
Solved in 3 steps

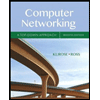
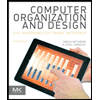
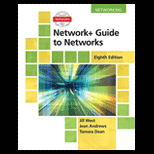
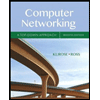
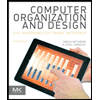
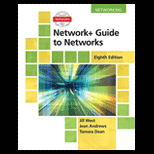
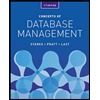
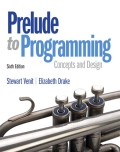
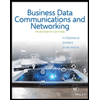