I have 2 java files. Car.java and CarValue.Java. Car.java looks like " public class Car { private int modelYear; // TODO: Declare purchasePrice field (int) private int currentValue; public void setModelYear(int userYear){ modelYear = userYear; } public int getModelYear() { return modelYear; } // TODO: Define setPurchasePrice() method // TODO: Define getPurchasePrice() method public void calcCurrentValue(int currentYear) { double depreciationRate = 0.15; int carAge = currentYear - modelYear; // Car depreciation formula currentValue = (int) Math.round(purchasePrice * Math.pow((1 - depreciationRate), carAge)); } // TODO: Define printInfo() method to output modelYear, purchasePrice, and currentValue }". While CarValue.java looks like " import java.util.Scanner; import java.lang.Math; public class CarValue { public static void main(String[] args) { Scanner scnr = new Scanner(System.in); Car myCar = new Car(); int userYear = scnr.nextInt(); int userPrice = scnr.nextInt(); int userCurrentYear = scnr.nextInt(); myCar.setModelYear(userYear); myCar.setPurchasePrice(userPrice); myCar.calcCurrentValue(userCurrentYear); myCar.printInfo(); } }". I need to insert the right code into specifically Car.java so when I input "2011 18000 2018" the output is "Car's information: Model year: 2011 Purchase price: 18000 Current value: 5770". My current code is: public class Car { private int modelYear; private int purchasePrice; private int currentValue; public int getModelYear() { return modelYear; } public void setModelYear(int userYear) { this.modelYear = userYear; } public int getPurchasePrice() { return purchasePrice; } public void setPurchasePrice(int userPrice) { this.purchasePrice = userPrice; } public int getCurrentValue() { return currentValue; } public void calcCurrenValue(int userCurrentYear){ double depreciationRate = 0.15; int carAge = userCurrentYear-modelYear; currentValue = (int)Math.round(purchasePrice * Math.pow((1-depreciationRate),carAge)); } public void printInfo(){ System.out.println("Car's Information:"); System.out.println(" Model year: "+getModelYear()); System.out.println(" Purchase price: "+getPurchasePrice()); System.out.println(" Current valud: "+getCurrentValue()); } }. But when I run it it keeps saying "CarValue.java:16: error: cannot find symbol myCar.calcCurrentValue(userCurrentYear); ^ symbol: method calcCurrentValue(int) location: variable myCar of type Car 1 error"
I have 2 java files. Car.java and CarValue.Java. Car.java looks like "
public class Car {
private int modelYear;
// TODO: Declare purchasePrice field (int)
private int currentValue;
public void setModelYear(int userYear){
modelYear = userYear;
}
public int getModelYear() {
return modelYear;
}
// TODO: Define setPurchasePrice() method
// TODO: Define getPurchasePrice() method
public void calcCurrentValue(int currentYear) {
double depreciationRate = 0.15;
int carAge = currentYear - modelYear;
// Car depreciation formula
currentValue = (int)
Math.round(purchasePrice * Math.pow((1 - depreciationRate), carAge));
}
// TODO: Define printInfo() method to output modelYear, purchasePrice, and currentValue
}". While CarValue.java looks like "
import java.util.Scanner;
import java.lang.Math;
public class CarValue {
public static void main(String[] args) {
Scanner scnr = new Scanner(System.in);
Car myCar = new Car();
int userYear = scnr.nextInt();
int userPrice = scnr.nextInt();
int userCurrentYear = scnr.nextInt();
myCar.setModelYear(userYear);
myCar.setPurchasePrice(userPrice);
myCar.calcCurrentValue(userCurrentYear);
myCar.printInfo();
}
}".
I need to insert the right code into specifically Car.java so when I input "2011 18000 2018" the output is "Car's information: Model year: 2011 Purchase price: 18000 Current value: 5770".
My current code is: public class Car { private int modelYear; private int purchasePrice; private int currentValue; public int getModelYear() { return modelYear; } public void setModelYear(int userYear) { this.modelYear = userYear; } public int getPurchasePrice() { return purchasePrice; } public void setPurchasePrice(int userPrice) { this.purchasePrice = userPrice; } public int getCurrentValue() { return currentValue; } public void calcCurrenValue(int userCurrentYear){ double depreciationRate = 0.15; int carAge = userCurrentYear-modelYear; currentValue = (int)Math.round(purchasePrice * Math.pow((1-depreciationRate),carAge)); } public void printInfo(){ System.out.println("Car's Information:"); System.out.println(" Model year: "+getModelYear()); System.out.println(" Purchase price: "+getPurchasePrice()); System.out.println(" Current valud: "+getCurrentValue()); } }.
But when I run it it keeps saying "CarValue.java:16: error: cannot find symbol myCar.calcCurrentValue(userCurrentYear); ^ symbol: method calcCurrentValue(int) location: variable myCar of type Car 1 error"


Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

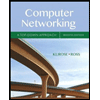
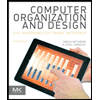
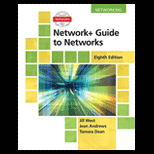
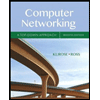
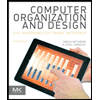
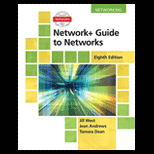
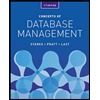
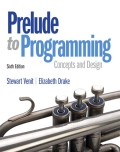
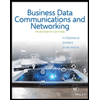