I got this error message for the following program: "Error! opening file" QUESTION: There are two files, f1 and f2, each containing a single integer. Write the statements needed to assign the larger of these two values to an int variable fmax. Declare any other variables you need. Test your code with some external files, f1 and f2. PROGRAM: #include #include int main() { int firstNum,secondNum; FILE *file1,*file2; int fmax; if ((file1 = fopen("input.txt","r")) == NULL) { printf("Error! opening file"); exit(1); } if ((file2 = fopen("input2.txt","r")) == NULL) { printf("Error! opening file"); exit(1); } fscanf(file1,"%d", &firstNum); fscanf(file2,"%d", &secondNum); if(firstNum>secondNum) { fmax=firstNum; printf("Maximum value is =%d from file input1.txt", fmax); } else { fmax=secondNum; printf("Maximum value is =%d from file input2.txt", fmax); } fclose(file1); fclose(file2); return 0; }
Hello,
I got this error message for the following
QUESTION:
There are two files, f1 and f2, each containing a single integer. Write the statements needed to assign the larger of these two values to an int variable fmax. Declare any other variables you need. Test your code with some external files, f1 and f2.
PROGRAM:
#include <stdio.h>
#include <stdlib.h>
int main()
{
int firstNum,secondNum;
FILE *file1,*file2;
int fmax;
if ((file1 = fopen("input.txt","r")) == NULL)
{
printf("Error! opening file");
exit(1);
}
if ((file2 = fopen("input2.txt","r")) == NULL)
{
printf("Error! opening file");
exit(1);
}
fscanf(file1,"%d", &firstNum);
fscanf(file2,"%d", &secondNum);
if(firstNum>secondNum)
{
fmax=firstNum;
printf("Maximum value is =%d from file input1.txt", fmax);
}
else
{
fmax=secondNum;
printf("Maximum value is =%d from file input2.txt", fmax);
}
fclose(file1);
fclose(file2);
return 0;
}

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 3 images

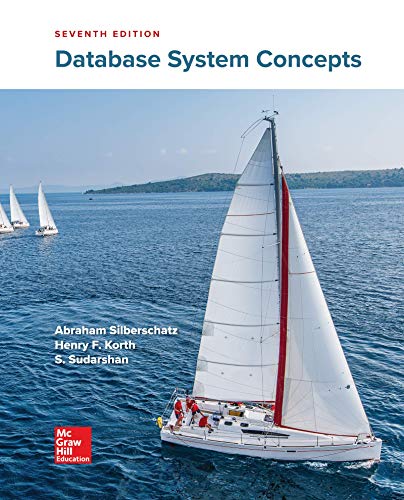
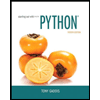
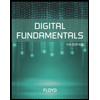
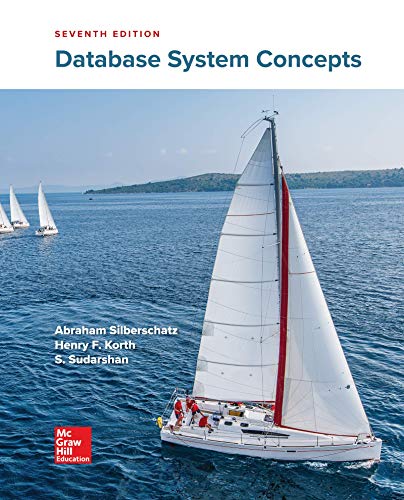
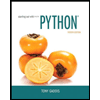
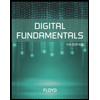
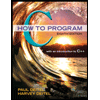
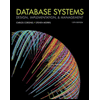
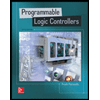