I am to write a python function that receives the root of a binary tree (t) and returns a list of all the nodes up to a specific node (k). the funcion must use recursion Starting code: def path_to_k(t,k): return [t.key]
I am to write a python function that receives the root of a binary tree (t) and returns a list of all the nodes up to a specific node (k). the funcion must use recursion
Starting code:
def path_to_k(t,k):
return [t.key]

Problem Statement:
You are given a binary tree, and your task is to write a Python function that finds and returns a list of all nodes from the root of the binary tree up to a specific target node k
. The function must use recursion to accomplish this.
Introduction:
In this problem, you are working with a binary tree data structure. A binary tree consists of nodes where each node has at most two children: a left child and a right child. The top node is called the "root," and nodes with no children are called "leaves." Each node contains a key or value.
Your goal is to find the path from the root of the binary tree to a specific target node k
and return a list containing all the nodes along this path. If the target node is not present in the tree, an empty list should be returned.
Logic:
To solve this problem, we can use a recursive approach. We'll start at the root of the tree and traverse it in a depth-first manner.
At each node, we'll check if the current node's key matches the target node
k
. If it does, we've found the target node, and we'll return a list containing only the current node's key.If the current node's key is not the target node
k
, we'll recursively search fork
in the left subtree and the right subtree.If we find
k
in either the left or right subtree, we'll prepend the current node's key to the path obtained from the subtree search and return it.If
k
is not found in either subtree, we'll return an empty list to indicate that the target node is not present in the current subtree.We'll repeat these steps until we either find the target node or exhaust all nodes in the tree.
Step by step
Solved in 4 steps with 1 images

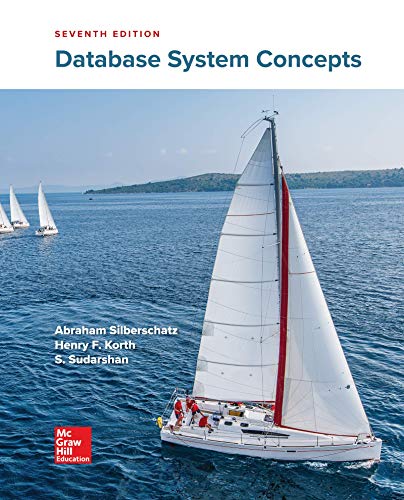
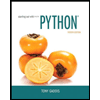
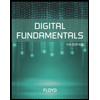
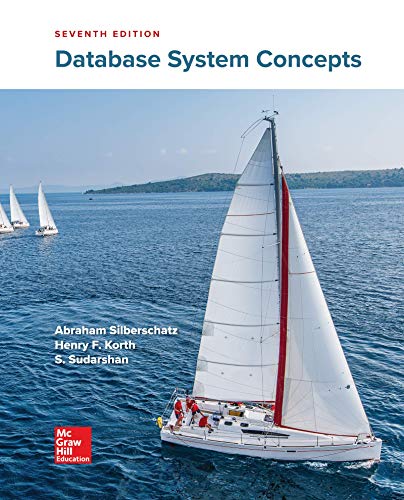
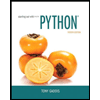
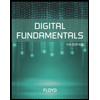
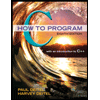
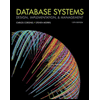
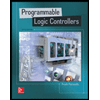