I am having trouble figuring out part b. Part b is important because the calculate button determines the entire problem, and I cannot figure out how to make it so it shows any text in the total due box. This is what I have for code so far, and none of it has 'errors', it just doesn't properly work. Public Class frmMain Private Function GetBusiness(ByVal dblConnections As Double) As Double ' Calculate the amount due. Dim dblAmountDue As Double dblAmountDue = 106.5 If dblConnections >= 10 Then dblAmountDue += 4 * (dblConnections - 10) End If Return dblAmountDue End Function Private Function GetResidential(ByVal dblChannels As Double) As Double ' Calculate the amount due. Dim dblAmountDue As Double dblAmountDue = 34.5 If dblChannels >= 1 Then dblAmountDue += 5 * (dblChannels - 1) End If Return dblAmountDue End Function Private Sub frmMain_Load(sender As Object, e As EventArgs) Handles MyBase.Load ' Populate lists. For intPremium As Integer = 0 To 20 ' Step 1 lstPremium.Items.Add(intPremium) Next intPremium lstPremium.SelectedIndex = 0 For intConnections As Integer = 0 To 100 ' Step 2 lstConnections.Items.Add(intConnections) Next intConnections lstConnections.SelectedIndex = 0 End Sub Private Sub btnExit_Click(sender As Object, e As EventArgs) Handles btnExit.Click Me.Close() End Sub Private Sub btnCalc_Click(sender As Object, e As EventArgs) Handles btnCalc.Click ' Display the amount due. Dim dblTotalDue As Double Dim dblPremium As Double Dim dblConnections As Double Dim dblChannels As Double Double.TryParse(txtTotalDue.Text, dblTotalDue) Double.TryParse(lstPremium.SelectedItem.ToString, dblPremium) Double.TryParse(lstConnections.SelectedItem.ToString, dblConnections) Dim dblAmountDue As Double ' Determine which radio button is selected and assign the fee. If radResidential.Checked = True Then dblAmountDue = 34.5 ElseIf dblChannels >= 1 Then dblAmountDue += 5 * (dblChannels - 1) ElseIf radBusiness.Checked = True Then dblAmountDue = 106.5 ElseIf dblConnections >= 10 Then dblAmountDue += 4 * (dblConnections - 10) End If End Sub Private Sub radBusiness_CheckedChanged(sender As Object, e As EventArgs) Handles radBusiness.CheckedChanged txtTotalDue.Text = String.Empty End Sub Private Sub radResidential_CheckedChanged(sender As Object, e As EventArgs) Handles radResidential.CheckedChanged txtTotalDue.Text = String.Empty End Sub Private Sub frmMain_FormClosing(sender As Object, e As System.Windows.Forms.FormClosingEventArgs) Handles Me.FormClosing ' Verify that the user wants to exit the application. Dim dlgButton As DialogResult dlgButton = MessageBox.Show("Do you want to exit?", "Chambers Sales", MessageBoxButtons.YesNo, MessageBoxIcon.Exclamation) ' If the No button is selected, do not close the form. If dlgButton = DialogResult.No Then e.Cancel = True End If End Sub End Class
I am having trouble figuring out part b. Part b is important because the calculate button determines the entire problem, and I cannot figure out how to make it so it shows any text in the total due box. This is what I have for code so far, and none of it has 'errors', it just doesn't properly work. Public Class frmMain Private Function GetBusiness(ByVal dblConnections As Double) As Double ' Calculate the amount due. Dim dblAmountDue As Double dblAmountDue = 106.5 If dblConnections >= 10 Then dblAmountDue += 4 * (dblConnections - 10) End If Return dblAmountDue End Function Private Function GetResidential(ByVal dblChannels As Double) As Double ' Calculate the amount due. Dim dblAmountDue As Double dblAmountDue = 34.5 If dblChannels >= 1 Then dblAmountDue += 5 * (dblChannels - 1) End If Return dblAmountDue End Function Private Sub frmMain_Load(sender As Object, e As EventArgs) Handles MyBase.Load ' Populate lists. For intPremium As Integer = 0 To 20 ' Step 1 lstPremium.Items.Add(intPremium) Next intPremium lstPremium.SelectedIndex = 0 For intConnections As Integer = 0 To 100 ' Step 2 lstConnections.Items.Add(intConnections) Next intConnections lstConnections.SelectedIndex = 0 End Sub Private Sub btnExit_Click(sender As Object, e As EventArgs) Handles btnExit.Click Me.Close() End Sub Private Sub btnCalc_Click(sender As Object, e As EventArgs) Handles btnCalc.Click ' Display the amount due. Dim dblTotalDue As Double Dim dblPremium As Double Dim dblConnections As Double Dim dblChannels As Double Double.TryParse(txtTotalDue.Text, dblTotalDue) Double.TryParse(lstPremium.SelectedItem.ToString, dblPremium) Double.TryParse(lstConnections.SelectedItem.ToString, dblConnections) Dim dblAmountDue As Double ' Determine which radio button is selected and assign the fee. If radResidential.Checked = True Then dblAmountDue = 34.5 ElseIf dblChannels >= 1 Then dblAmountDue += 5 * (dblChannels - 1) ElseIf radBusiness.Checked = True Then dblAmountDue = 106.5 ElseIf dblConnections >= 10 Then dblAmountDue += 4 * (dblConnections - 10) End If End Sub Private Sub radBusiness_CheckedChanged(sender As Object, e As EventArgs) Handles radBusiness.CheckedChanged txtTotalDue.Text = String.Empty End Sub Private Sub radResidential_CheckedChanged(sender As Object, e As EventArgs) Handles radResidential.CheckedChanged txtTotalDue.Text = String.Empty End Sub Private Sub frmMain_FormClosing(sender As Object, e As System.Windows.Forms.FormClosingEventArgs) Handles Me.FormClosing ' Verify that the user wants to exit the application. Dim dlgButton As DialogResult dlgButton = MessageBox.Show("Do you want to exit?", "Chambers Sales", MessageBoxButtons.YesNo, MessageBoxIcon.Exclamation) ' If the No button is selected, do not close the form. If dlgButton = DialogResult.No Then e.Cancel = True End If End Sub End Class
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
100%
I am having trouble figuring out part b. Part b is important because the calculate button determines the entire problem, and I cannot figure out how to make it so it shows any text in the total due box. This is what I have for code so far, and none of it has 'errors', it just doesn't properly work.
Public Class frmMain
Private Function GetBusiness(ByVal dblConnections As Double) As Double
' Calculate the amount due.
Dim dblAmountDue As Double
dblAmountDue = 106.5
If dblConnections >= 10 Then
dblAmountDue += 4 * (dblConnections - 10)
End If
Return dblAmountDue
End Function
Private Function GetResidential(ByVal dblChannels As Double) As Double
' Calculate the amount due.
Dim dblAmountDue As Double
dblAmountDue = 34.5
If dblChannels >= 1 Then
dblAmountDue += 5 * (dblChannels - 1)
End If
Return dblAmountDue
End Function
Private Sub frmMain_Load(sender As Object, e As EventArgs) Handles MyBase.Load
' Populate lists.
For intPremium As Integer = 0 To 20 ' Step 1
lstPremium.Items.Add(intPremium)
Next intPremium
lstPremium.SelectedIndex = 0
For intConnections As Integer = 0 To 100 ' Step 2
lstConnections.Items.Add(intConnections)
Next intConnections
lstConnections.SelectedIndex = 0
End Sub
Private Sub btnExit_Click(sender As Object, e As EventArgs) Handles btnExit.Click
Me.Close()
End Sub
Private Sub btnCalc_Click(sender As Object, e As EventArgs) Handles btnCalc.Click
' Display the amount due.
Dim dblTotalDue As Double
Dim dblPremium As Double
Dim dblConnections As Double
Dim dblChannels As Double
Double.TryParse(txtTotalDue.Text, dblTotalDue)
Double.TryParse(lstPremium.SelectedItem.ToString, dblPremium)
Double.TryParse(lstConnections.SelectedItem.ToString, dblConnections)
Dim dblAmountDue As Double
' Determine which radio button is selected and assign the fee.
If radResidential.Checked = True Then
dblAmountDue = 34.5
ElseIf dblChannels >= 1 Then
dblAmountDue += 5 * (dblChannels - 1)
ElseIf radBusiness.Checked = True Then
dblAmountDue = 106.5
ElseIf dblConnections >= 10 Then
dblAmountDue += 4 * (dblConnections - 10)
End If
End Sub
Private Sub radBusiness_CheckedChanged(sender As Object, e As EventArgs) Handles radBusiness.CheckedChanged
txtTotalDue.Text = String.Empty
End Sub
Private Sub radResidential_CheckedChanged(sender As Object, e As EventArgs) Handles radResidential.CheckedChanged
txtTotalDue.Text = String.Empty
End Sub
Private Sub frmMain_FormClosing(sender As Object, e As System.Windows.Forms.FormClosingEventArgs) Handles Me.FormClosing
' Verify that the user wants to exit the application.
Dim dlgButton As DialogResult
dlgButton = MessageBox.Show("Do you want to exit?",
"Chambers Sales",
MessageBoxButtons.YesNo,
MessageBoxIcon.Exclamation)
' If the No button is selected, do not close the form.
If dlgButton = DialogResult.No Then
e.Cancel = True
End If
End Sub
End Class

Transcribed Image Text:residential customer with 3 premium channels is $49.50.)
Figure 6-51
Interface for Exercise 11
Cable Direct
Business customers
Processing fee
Basic service fee
Premium channels
Business
Residential
Total due:
Figure 6-52
Cable rates for Exercise 11
Residential customers
Processing fee
Basic service fee
Premium channels
Premium channels:
Ist Premiu
$4.50
$30
$5 per channel
Calculate
Connections:
Ist Conne
Exit
$16.50
$80 for the first 10 connections; $4 each additional connection
$50 per channel for any number of connections

Transcribed Image Text:Advanced
11. In this exercise, you create an application that calculates a customer's cable bill. Create a Windows
Forms application. Use the following names for the project and solution, respectively: Cable Direct
Project and Cable Direct Solution. Save the application in the VB9e\Chap06 folder. (F6.1, F6.3, F6.5,
A6.4, A6.5, A6.7-A6.10)
a. Create the interface shown in Figure 6-51. Display numbers from 0 through 20 in the
IstPremium control. Display numbers from 0 through 100 in the IstConnections control. When
the interface appears, the first item in each list box should be selected.
b. The Calculate button's Click event procedure should calculate and display a customer's cable
bill. The cable rates are shown in Figure 6-52. Business customers must have at least one
connection. Use two functions: one to calculate and return the total due for business
customers, and one to calculate and return the total due for residential customers.
c. The form's FormClosing event procedure should verify that the user wants to close the
application.
d. The total due should be cleared when a change is made to a radio button or list box.
e. Save the solution and then start and test the application. (The total due for a business
customer with 3 premium channels and 12 connections is $254.50. The total due for a
residential customer with 3 premium channels is $49.50.)
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 3 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
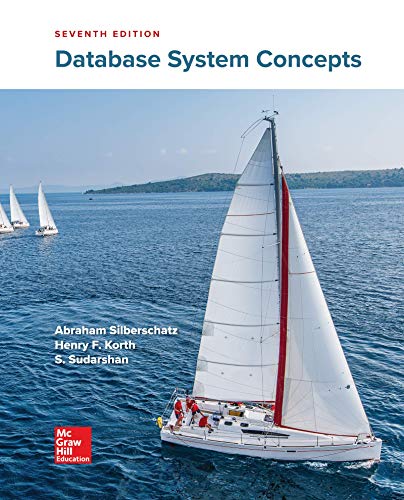
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
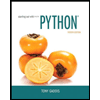
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
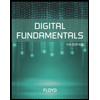
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
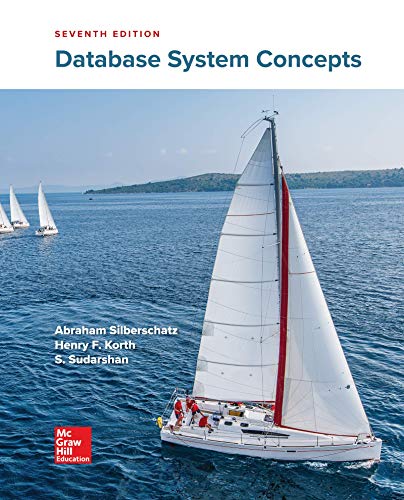
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
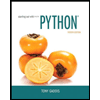
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
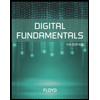
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
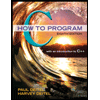
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
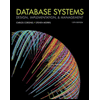
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
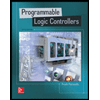
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education