I am having difficulty understanding how the log works for this .cpp file. Can someone help me understand how the c++ language is working? #include "swimmingPool.h" #include using namespace std; // function to set the length, width, depth, waterFlowInRate, waterFlowOutRate and amountOfWaterInPool void swimmingPool::set (double l, double w, double d, double fi, double fo, double wInPool) { length = l; // feet width = w; // feet depth = d; // feet waterFlowInRate = fi; // gallons per minute waterFlowOutRate = fo; // gallons per minute amountOfWaterInPool = wInPool; // in gallons } // function to set the length void swimmingPool::setLength (double l) { length = l; } // function to set the width void swimmingPool::setWidth (double w) { width = w; } // function to set the depth void swimmingPool::setDepth (double d) { depth = d; } // function to set waterFlowInRate void swimmingPool::setWaterFlowRateIn (double fi) { waterFlowInRate = fi; } // function to set waterFlowOutRate void swimmingPool::setWaterFlowRateOut (double fo) { waterFlowOutRate = fo; } // function to add water to the pool void swimmingPool::addWater (double time, double fillRate) { // calculate the amount of water to add in gallons double waterToAdd = time * fillRate * GALLONS_IN_A_CUBIC_FEET; // check if adding the water will cause overflow, then display message and set amountOfWaterInPool to pool capacity if ((amountOfWaterInPool + waterToAdd) > poolTotalWaterCapacity ()) { cout << "Pool overflow" << endl; amountOfWaterInPool = poolTotalWaterCapacity (); } else amountOfWaterInPool += waterToAdd; // else add that amount of water to pool } // function to drain water from the pool void swimmingPool::drainWater (double time, double drainRate) { // calculate the amount of water to drain in gallons double waterToDrain = time * drainRate * GALLONS_IN_A_CUBIC_FEET; amountOfWaterInPool -= waterToDrain; // subtract that amount from current amount of water in pool // if negative, set it to 0 if (amountOfWaterInPool < 0) amountOfWaterInPool = 0; } // function to calculate and return the total capacity of pool in gallons double swimmingPool::poolTotalWaterCapacity () { return (length * width * depth * GALLONS_IN_A_CUBIC_FEET); } // function to return the length double swimmingPool::getLength () { return length; } // function to return the width double swimmingPool::getWidth () { return width; } // function to return the depth double swimmingPool::getDepth () { return depth; } // function to return the waterFlowInRate double swimmingPool::getWaterFlowRateIn () { return waterFlowInRate; } // function to return the waterFlowOutRate double swimmingPool::getWaterFlowRateOut () { return waterFlowOutRate; } // function to return the amount of water in pool double swimmingPool::getTotalWaterInPool () { return amountOfWaterInPool; } // function to calculate the time to completely fill the pool (in minutes) int swimmingPool::timeToFillThePool () { double remainingWater = waterNeededToFillThePool (); // get the total amount of water that can be added to the pool // if waterFlowInRate > 0, calculate the time if (waterFlowInRate > 0) return remainingWater / waterFlowInRate; return 0; // waterFlowInRate is 0 } // function to calculate the time to completely drain the pool (in minutes) int swimmingPool::timeToDrainThePool () { if (waterFlowOutRate > 0) // waterFlowOutRate > 0, calculate time return amountOfWaterInPool / waterFlowOutRate; return 0; // waterFlowOutRate is 0 } // function to calculate the total amount of water needed to fill the pool (in gallons) double swimmingPool::waterNeededToFillThePool () { return (poolTotalWaterCapacity () - amountOfWaterInPool); } // constructor to initialize the pool fields swimmingPool::swimmingPool (double l, double w, double d, double fi, double fo, double wInPool):length (l), width (w), depth (d), waterFlowInRate (fi), waterFlowOutRate (fo), amountOfWaterInPool (wInPool) { } //end of swimmingPool.cpp
I am having difficulty understanding how the log works for this .cpp file. Can someone help me understand how the c++ language is working?
#include "swimmingPool.h"
#include <iostream>
using namespace std;
// function to set the length, width, depth, waterFlowInRate, waterFlowOutRate and amountOfWaterInPool
void
swimmingPool::set (double l, double w, double d,
double fi, double fo, double wInPool)
{
length = l; // feet
width = w; // feet
depth = d; // feet
waterFlowInRate = fi; // gallons per minute
waterFlowOutRate = fo; // gallons per minute
amountOfWaterInPool = wInPool; // in gallons
}
// function to set the length
void
swimmingPool::setLength (double l)
{
length = l;
}
// function to set the width
void
swimmingPool::setWidth (double w)
{
width = w;
}
// function to set the depth
void
swimmingPool::setDepth (double d)
{
depth = d;
}
// function to set waterFlowInRate
void
swimmingPool::setWaterFlowRateIn (double fi)
{
waterFlowInRate = fi;
}
// function to set waterFlowOutRate
void
swimmingPool::setWaterFlowRateOut (double fo)
{
waterFlowOutRate = fo;
}
// function to add water to the pool
void
swimmingPool::addWater (double time, double fillRate)
{
// calculate the amount of water to add in gallons
double waterToAdd = time * fillRate * GALLONS_IN_A_CUBIC_FEET;
// check if adding the water will cause overflow, then display message and set amountOfWaterInPool to pool capacity
if ((amountOfWaterInPool + waterToAdd) > poolTotalWaterCapacity ())
{
cout << "Pool overflow" << endl;
amountOfWaterInPool = poolTotalWaterCapacity ();
}
else
amountOfWaterInPool += waterToAdd; // else add that amount of water to pool
}
// function to drain water from the pool
void
swimmingPool::drainWater (double time, double drainRate)
{
// calculate the amount of water to drain in gallons
double waterToDrain = time * drainRate * GALLONS_IN_A_CUBIC_FEET;
amountOfWaterInPool -= waterToDrain; // subtract that amount from current amount of water in pool
// if negative, set it to 0
if (amountOfWaterInPool < 0)
amountOfWaterInPool = 0;
}
// function to calculate and return the total capacity of pool in gallons
double
swimmingPool::poolTotalWaterCapacity ()
{
return (length * width * depth * GALLONS_IN_A_CUBIC_FEET);
}
// function to return the length
double
swimmingPool::getLength ()
{
return length;
}
// function to return the width
double
swimmingPool::getWidth ()
{
return width;
}
// function to return the depth
double
swimmingPool::getDepth ()
{
return depth;
}
// function to return the waterFlowInRate
double
swimmingPool::getWaterFlowRateIn ()
{
return waterFlowInRate;
}
// function to return the waterFlowOutRate
double
swimmingPool::getWaterFlowRateOut ()
{
return waterFlowOutRate;
}
// function to return the amount of water in pool
double
swimmingPool::getTotalWaterInPool ()
{
return amountOfWaterInPool;
}
// function to calculate the time to completely fill the pool (in minutes)
int
swimmingPool::timeToFillThePool ()
{
double remainingWater = waterNeededToFillThePool (); // get the total amount of water that can be added to the pool
// if waterFlowInRate > 0, calculate the time
if (waterFlowInRate > 0)
return remainingWater / waterFlowInRate;
return 0; // waterFlowInRate is 0
}
// function to calculate the time to completely drain the pool (in minutes)
int
swimmingPool::timeToDrainThePool ()
{
if (waterFlowOutRate > 0) // waterFlowOutRate > 0, calculate time
return amountOfWaterInPool / waterFlowOutRate;
return 0; // waterFlowOutRate is 0
}
// function to calculate the total amount of water needed to fill the pool (in gallons)
double
swimmingPool::waterNeededToFillThePool ()
{
return (poolTotalWaterCapacity () - amountOfWaterInPool);
}
// constructor to initialize the pool fields
swimmingPool::swimmingPool (double l, double w, double d, double fi, double fo, double wInPool):length (l), width (w), depth (d), waterFlowInRate (fi), waterFlowOutRate (fo),
amountOfWaterInPool
(wInPool)
{
}
//end of swimmingPool.cpp

The C++ code file that is being made available seems to be a component of a program that mimics a swimming pool and offers tools for controlling and managing the pool. The swimmingPool class, which is defined in this code, has member functions for configuring pool settings, adding and draining water, computing capacity and time-related metrics, and obtaining pool data.
Please refer to the following steps for the complete solution to the problem above.
Step by step
Solved in 3 steps

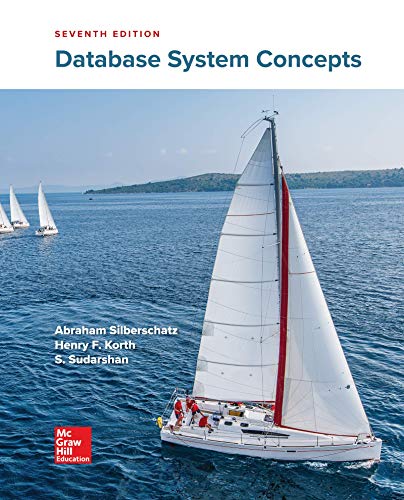
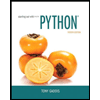
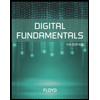
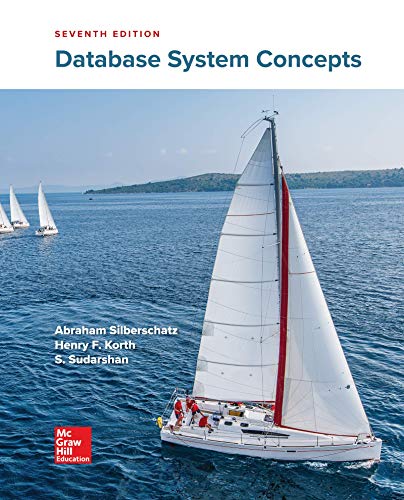
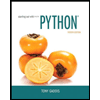
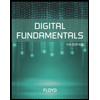
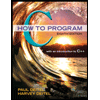
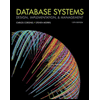
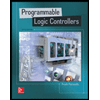