Use C++ Using dynamic arrays, implement a polynomial class with polynomial addition, subtraction, and multiplication. Implement addition and subtraction functions first.
Use C++
Using dynamic arrays, implement a polynomial class with polynomial addition, subtraction, and multiplication.
Implement addition and subtraction functions first.
it should have five files
One is the main.cpp file
One is the Polynomial.cpp file it'sit's in the picture
One is the Polynomial.h file
One is A.txt
One is B.txt
The contents of A and B.txt are in the picture
Here is the main.cpp code I have:
#include <iostream>
#include <fstream>
#include "polynomial.h"
using namespace std;
int main()
{
// read data from A.txt
ifstream infile;
infile.open("A.txt");
if (infile.fail()) {
cout << "Input file opening failed." << endl;
exit(1);
}
int degree_A;
infile >> degree_A;
int* coef_A = new int[degree_A+1];
for (int i = 0; i < degree_A + 1; i++)
coef_A[i] = 0;
int coef, exp;
while (!infile.eof())
{
infile >> coef >> exp;
coef_A[exp] = coef;
}
infile.close();
// read data from B.txt
infile.open("B.txt");
if (infile.fail()) {
cout << "Input file opening failed." << endl;
exit(1);
}
int degree_B;
infile >> degree_B;
int* coef_B = new int[degree_B + 1];
for (int i = 0; i < degree_B + 1; i++)
coef_B[i] = 0;
while (!infile.eof())
{
infile >> coef >> exp;
coef_B[exp] = coef;
}
infile.close();
Polynomial p1(coef_A, degree_A);
Polynomial p2(coef_B, degree_B);
Polynomial p3 = add(p1, p2);
Polynomial p4 = subtract(p1, p2);
// Print out p1, p2, p3
return 0;
}



Trending now
This is a popular solution!
Step by step
Solved in 3 steps

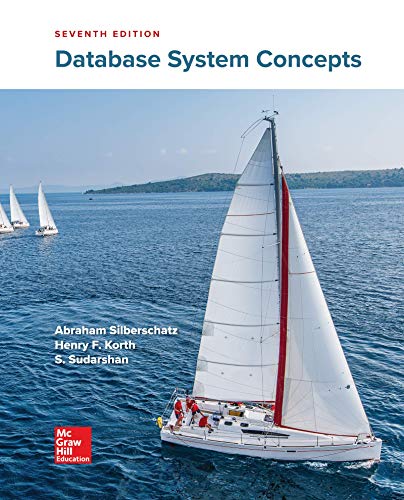
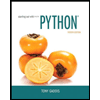
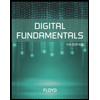
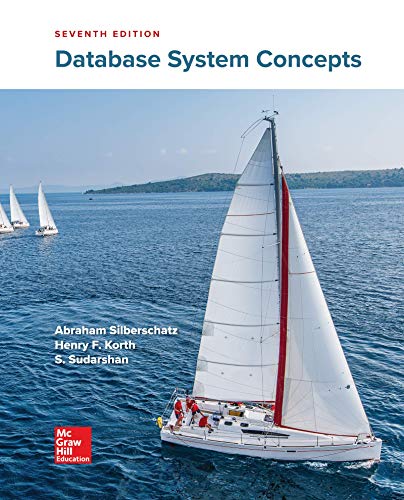
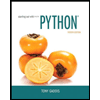
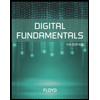
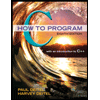
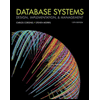
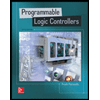