I am having an issue with declaring a construtor - LINE 104 TimeOff::TimeOff(string, int, double, double, double, double, double) Can you review my code and let me know what I am doing wrong. I got help from your site, however, they created 2 files, a header and a source cpp. My assignment is to turn in one file, with the headers and source code. See below and attached //Sharissa R Sullivan //03.18.23 //More about Classes #include #include using namespace std; //Number Days Class class NumDays { // Access specifer private: double hours; double days; public: NumDays(double h = 0) { hours = h; days = h / 8; } void setHours(double h) { hours = h; days = h / 8; } void setDays(double d) { days = d; hours = d * 8; } double getHours()const { return hours; } double getDays()const { return days; } //overloaded + operator NumDays operator+(NumDays& right) { NumDays temp; temp.setHours(hours + right.getHours()); return temp; } //overloaded - operator NumDays operator -(NumDays& right) { NumDays temp; temp.setHours(hours - right.getHours()); return temp; } //overloaded prefix ++ NumDays operator ++() { ++hours; days = hours / 8; return *this; } //overloaded operator postfix ++ NumDays operator ++(int) { hours++; NumDays temp(hours); days = hours / 8; return temp; } //overloaded operator prefix -- NumDays operator --() { --hours; days = hours / 8; return *this; } //overloaded operator postfix-- NumDays operator --(int) { hours--; NumDays temp(hours); days = hours / 8; return temp; } }; class TimeOff { // Declare name and ID number variables private: string name; int empNumber; // create objects from the Numdays Class NumDays maxSickDays, sickTaken, maxVacation, vacTaken, maxUnpaid, unpaidTaken; public: //Declare Constructor TimeOff(string = "", int = 0, double = 0, double = 0, double = 0, double = 0, double = 0); // Mutators/ SET fucntions void setname(string n) { name = n; } void setMaxSick(double h) { maxSickDays.setHours(h); } //call the function "set hours" by passing hours void setSickTaken(double h) { sickTaken.setHours(h); //subtract "getMaxsick from hours setMaxSick(getMaxSick() - h); } void setMaxVacation(double h) { if (maxVacation.getHours() + h > 240) maxVacation.setHours(240); else // call the "max vaction with hours maxVacation.setHours(h); } //call the function set hours by passign hours void setVactionTaken(double h) { vacTaken.setHours(h); setMaxVacation(getMaxVacation() - h); } void setmaxUnpaid(double h) { maxUnpaid.setHours(h); } void setUnpaid(double h) { unpaidTaken.setHours(h); setmaxUnpaid(_getmaxstdio() - h); } // Get Functions/Accessors // return max Sick days string getName() const { return name; } double getMaxSick() const { return sickTaken.getHours(); } double getMaxVacation() const { return maxVacation.getHours(); } double getVactionTake() const { return vacTaken.getHours(); } double getMaxUnpaid() const { return maxUnpaid.getHours(); } double getUnpaidTaken() const { return unpaidTaken.getHours(); } double getMaxSickDays() const { return maxSickDays.getDays(); } double getSickTakenDays() const { return sickTaken.getDays(); } double getMaxVactionDays() const { return maxVacation.getDays(); } double getVacatoionDaysTaken() const { return vacTaken.getDays(); } double getMaxUnpaidDays() const { return maxUnpaid.getDays(); } double getUnpaidTakenDays()const { return unpaidTaken.getDays(); } }; int main() { // create ogject for Time off class and pass values TimeOff t("John,1234,0,0,0,0,0,0"); //declare varaibles int months; // v=vacation time s= sick time double v,s; //Get the user to input the months cout << "enter the months has" << t.getName() << "worked at the company:"; cin >> months; // Calculate vanction and sick days v = months * 12; s = months * 8; //call the function setMaxVacation t.setMaxVacation(v); t.setMaxSick(s); //Dosplay the output cout << "\nYour available vacation leave for" << t.getName() << "is:" << t.getMaxVactionDays() << "days\n"; cout << "\nYour available sick leave for" << t.getName() << "is:" << t.getMaxSickDays() << "days\n"; return 0; }
I am having an issue with declaring a construtor - LINE 104 TimeOff::TimeOff(string, int, double, double, double, double, double)
Can you review my code and let me know what I am doing wrong.
I got help from your site, however, they created 2 files, a header and a source cpp. My assignment is to turn in one file, with the headers and source code. See below and attached
//Sharissa R Sullivan
//03.18.23
//More about Classes
#include <iostream>
#include <string>
using namespace std;
//Number Days Class
class NumDays
{
// Access specifer
private:
double hours;
double days;
public:
NumDays(double h = 0)
{
hours = h;
days = h / 8;
}
void setHours(double h)
{
hours = h;
days = h / 8;
}
void setDays(double d)
{
days = d;
hours = d * 8;
}
double getHours()const
{
return hours;
}
double getDays()const
{
return days;
}
//overloaded + operator
NumDays operator+(NumDays& right)
{
NumDays temp;
temp.setHours(hours + right.getHours());
return temp;
}
//overloaded - operator
NumDays operator -(NumDays& right)
{
NumDays temp;
temp.setHours(hours - right.getHours());
return temp;
}
//overloaded prefix ++
NumDays operator ++()
{
++hours;
days = hours / 8;
return *this;
}
//overloaded operator postfix ++
NumDays operator ++(int)
{
hours++;
NumDays temp(hours);
days = hours / 8;
return temp;
}
//overloaded operator prefix --
NumDays operator --()
{
--hours;
days = hours / 8;
return *this;
}
//overloaded operator postfix--
NumDays operator --(int)
{
hours--;
NumDays temp(hours);
days = hours / 8;
return temp;
}
};
class TimeOff
{
// Declare name and ID number variables
private:
string name;
int empNumber;
// create objects from the Numdays Class
NumDays
maxSickDays,
sickTaken,
maxVacation,
vacTaken,
maxUnpaid,
unpaidTaken;
public:
//Declare Constructor
TimeOff(string = "", int = 0, double = 0, double = 0, double = 0, double = 0, double = 0);
// Mutators/ SET fucntions
void setname(string n)
{
name = n;
}
void setMaxSick(double h)
{
maxSickDays.setHours(h);
}
//call the function "set hours" by passing hours
void setSickTaken(double h)
{
sickTaken.setHours(h);
//subtract "getMaxsick from hours
setMaxSick(getMaxSick() - h);
}
void setMaxVacation(double h)
{
if (maxVacation.getHours() + h > 240)
maxVacation.setHours(240);
else
// call the "max vaction with hours
maxVacation.setHours(h);
}
//call the function set hours by passign hours
void setVactionTaken(double h)
{
vacTaken.setHours(h);
setMaxVacation(getMaxVacation() - h);
}
void setmaxUnpaid(double h)
{
maxUnpaid.setHours(h);
}
void setUnpaid(double h)
{
unpaidTaken.setHours(h);
setmaxUnpaid(_getmaxstdio() - h);
}
// Get Functions/Accessors
// return max Sick days
string getName() const
{
return name;
}
double getMaxSick() const
{
return sickTaken.getHours();
}
double getMaxVacation() const
{
return maxVacation.getHours();
}
double getVactionTake() const
{
return vacTaken.getHours();
}
double getMaxUnpaid() const
{
return maxUnpaid.getHours();
}
double getUnpaidTaken() const
{
return unpaidTaken.getHours();
}
double getMaxSickDays() const
{
return maxSickDays.getDays();
}
double getSickTakenDays() const
{
return sickTaken.getDays();
}
double getMaxVactionDays() const
{
return maxVacation.getDays();
}
double getVacatoionDaysTaken() const
{
return vacTaken.getDays();
}
double getMaxUnpaidDays() const
{
return maxUnpaid.getDays();
}
double getUnpaidTakenDays()const
{
return unpaidTaken.getDays();
}
};
int main()
{
// create ogject for Time off class and pass values
TimeOff t("John,1234,0,0,0,0,0,0");
//declare varaibles
int months;
// v=vacation time s= sick time
double v,s;
//Get the user to input the months
cout << "enter the months has" << t.getName() << "worked at the company:";
cin >> months;
// Calculate vanction and sick days
v = months * 12;
s = months * 8;
//call the function setMaxVacation
t.setMaxVacation(v);
t.setMaxSick(s);
//Dosplay the output
cout << "\nYour available vacation leave for" << t.getName() << "is:" << t.getMaxVactionDays() << "days\n";
cout << "\nYour available sick leave for" << t.getName() << "is:" << t.getMaxSickDays() << "days\n";
return 0;
}


Trending now
This is a popular solution!
Step by step
Solved in 4 steps

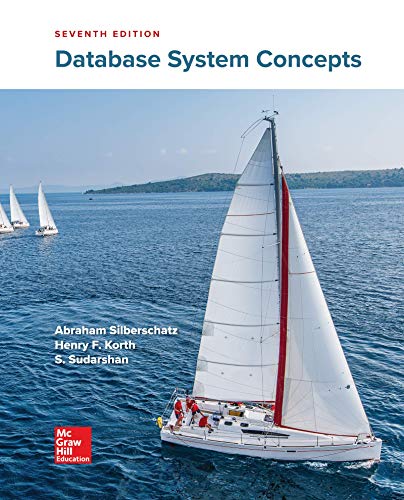
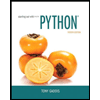
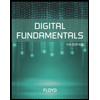
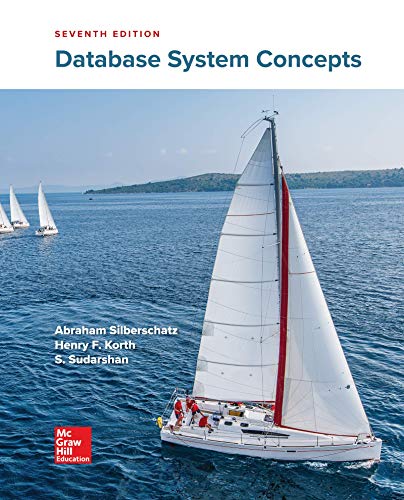
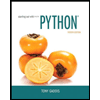
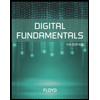
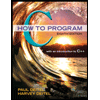
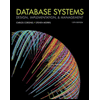
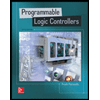