Lab Tasks – complete these Use the information provided here, in the slideshow and in chapter 12 of our text to do the following exercise. Step 1: On the desktop or wherever you will be saving your java program, use notepador other basic text editor (NOT WORD) to create a .txt file. In this file, type out a list of 10 integers (one on each line or with a space between each). Save. Do this manually. Not with Java. Step 2. Now write a program with the following: 1) In the program creating a File object that connects to the .txt file that you just created. 2) Add code that will check if the file exists and if it doesn’t, it will create it. (Yes, we know it definitely exists but do this part anyway.) 3) Set up a Scanner so that you can read the values from the file and print them to the screen using a loop. You will probably need to use the .hasNext() method. Also, you may want to set up a counter to keep track of how many values there are for future use. (Note that the .length() method for File doesn’t give you the number of values. It gives you the number of bytes. Test it out and go check the file size of your txt file if you’re interested.) Step 3. Stop and do a check here. All values from your file should print to the screenwithout crashing. If this works, continue. Step 4. Continuing with your code, create another loop that reads the values again and then stores them in an array. (You may want to use the value you saved for your counter as the upper limit in your for loop and as the size of your array.)(Also, note that before we run this second loop, the scanner has already reached the bottom of the file and will not be able to find anything in it. To fix this, set up a new Scanner object and pass in the original File object again.) Also within this loop, calculate the total of all the values that are read into the array. Set up a print statement that prints the final total. Step 5. Stop and do a check here. All values and the total of all values should print to the screen. You should have an array in your code that is filled with your values. Step 6. Now, in your program, 1) Create a completely new file object in your code and use a file name that doesn’t exist yet. (We’ll let the program create it for us.) 2) Set up the code required to check if the file exists and if it doesn’t, it should create it. 3) Create a PrintWriter object so we can write to this new file. 4) Set up a loop that will read through your array and use PrintWriter’s .println() method to write each value to the new file BUT add 5 to each value before writing it. 5) Don’t forget to close out the PrintWriter stream or the data will not actually write to the file. What I will be looking for when it’s time to check: • The first .txt file in which you manually typed integers • Values from the first file and the sum printed to the screen • Your new .txt file with the new values in it (old values + 5) after program runs • Your code to make sure you’ve used everything correctly and set up an array Sample output: 1 2 3 4 5 6 7 8 9 10 Total: 55 6 7 8 9 10 11 12 13 14 15 in java
Lab Tasks – complete these
Use the information provided here, in the slideshow and in chapter 12 of our text to do the following exercise.
Step 1: On the desktop or wherever you will be saving your java program, use notepador other basic text editor (NOT WORD) to create a .txt file. In this file, type out a list of 10 integers (one on each line or with a space between each). Save. Do this manually. Not with Java.
Step 2. Now write a program with the following:
Step 3. Stop and do a check here. All values from your file should print to the screenwithout crashing. If this works, continue.
Step 4. Continuing with your code, create another loop that reads the values again and then stores them in an array. (You may want to use the value you saved for your counter as the upper limit in your for loop and as the size of your array.)(Also, note that before we run this second loop, the scanner has already reached the bottom of the file and will not be able to find anything in it. To fix this, set up a new Scanner object and pass in the original File object again.) Also within this loop, calculate the total of all the values that are read into the array. Set up a print statement that prints the final total.
Step 5. Stop and do a check here. All values and the total of all values should print to the screen. You should have an array in your code that is filled with your values.
Step 6. Now, in your program,
What I will be looking for when it’s time to check:
Sample output:
1
2
3
4
5
6
7
8
9
10
Total: 55
6
7
8
9
10
11
12
13
14
15

Step by step
Solved in 2 steps with 2 images

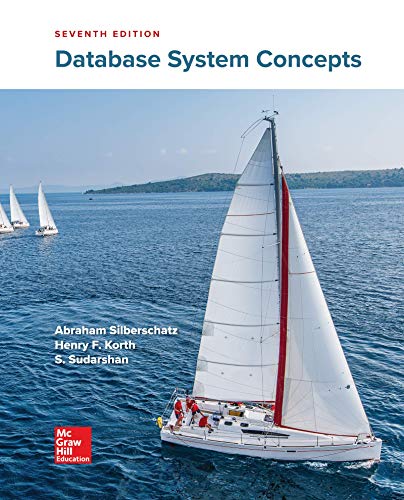
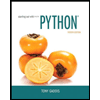
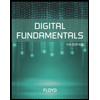
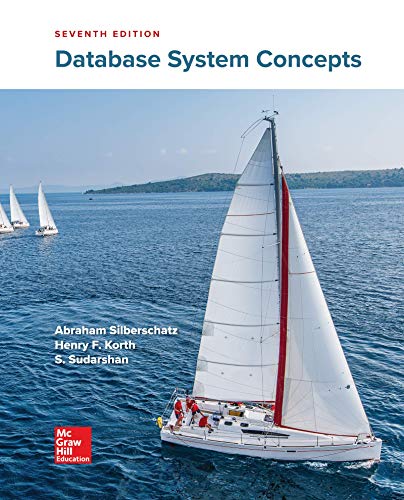
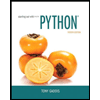
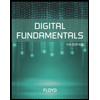
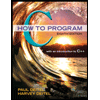
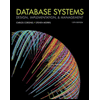
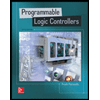