I am getting a few errors in this java program. I need help figuring out what I am doing wrong. Thanks so much for your help. public class BSTTree { private TreeNode root; public void insert (int value) { if (root == null) { root = new TreeNode(value); } else{ root.insert(value); } } public TreeNode get(int value){ if (root != null) { return root.get(value); } return null; } public int min(){ if (root == null){ return Integer.MAX_VALUE; } else { return root.max(); } } public void traverseInOrder(){ if (root != null){ root.traverseInOrder(); } } public void delete(TreeNode root, int value) { root = delete(root, value); } private TreeNode delete(TreeNode subtreeRoot, int value){ if (subtreeRoot == null){ System.out.println("fail to find the node and reach the leaf"); return subtreeRoot; } if (value < subtreeRoot.getData()){ subtreeRoot.setLeftChild(delete(subtreeRoot.getLeftChild(), value)); } else if (value > subtreeRoot.getData()){ subtreeRoot.setRightChild(delete(subtreeRoot.getRightChild(), value)); } else { if (subtreeRoot.getLeftChild() == null){ System.out.println("case 1 and 2 parent " + subtreeRoot+ " RightChild " + subtreeRoot.getRightChild()); return subtreeRoot.getRightChild(); } else if (subtreeRoot.getRightChild() == null){ System.out.println("case 1 and 2 parent " + subtreeRoot + " LeftChild " + subtreeRoot.getLeftChild()); return subtreeRoot.getLeftChild(); } else { System.out.println("node with two children to be deleted " + subtreeRoot); System.out.println("case 3: replacement node " + subtreeRoot.getRightChild().min()); subtreeRoot.setData(subtreeRoot.getRightChild().min()); System.out.println("delete min value in subtree"); subtreeRoot.setRightChild(delete(subtreeRoot.getRightChild(), subtreeRoot.getData())); } } System.out.print("Original node " + subtreeRoot + " "); return subtreeRoot; } }
I am getting a few errors in this java
public class BSTTree {
private TreeNode root;
public void insert (int value) {
if (root == null) {
root = new TreeNode(value);
}
else{
root.insert(value);
}
}
public TreeNode get(int value){
if (root != null) {
return root.get(value);
}
return null;
}
public int min(){
if (root == null){
return Integer.MAX_VALUE;
}
else {
return root.max();
}
}
public void traverseInOrder(){
if (root != null){
root.traverseInOrder();
}
}
public void delete(TreeNode root, int value) { root = delete(root, value); }
private TreeNode delete(TreeNode subtreeRoot, int value){
if (subtreeRoot == null){
System.out.println("fail to find the node and reach the leaf");
return subtreeRoot;
}
if (value < subtreeRoot.getData()){
subtreeRoot.setLeftChild(delete(subtreeRoot.getLeftChild(), value));
}
else if (value > subtreeRoot.getData()){
subtreeRoot.setRightChild(delete(subtreeRoot.getRightChild(), value));
}
else {
if (subtreeRoot.getLeftChild() == null){
System.out.println("case 1 and 2 parent " + subtreeRoot+ " RightChild " + subtreeRoot.getRightChild());
return subtreeRoot.getRightChild();
}
else if (subtreeRoot.getRightChild() == null){
System.out.println("case 1 and 2 parent " + subtreeRoot + " LeftChild " + subtreeRoot.getLeftChild());
return subtreeRoot.getLeftChild();
} else {
System.out.println("node with two children to be deleted " + subtreeRoot);
System.out.println("case 3: replacement node " + subtreeRoot.getRightChild().min());
subtreeRoot.setData(subtreeRoot.getRightChild().min());
System.out.println("delete min value in subtree");
subtreeRoot.setRightChild(delete(subtreeRoot.getRightChild(), subtreeRoot.getData()));
}
}
System.out.print("Original node " + subtreeRoot + " ");
return subtreeRoot;
}
}

Trending now
This is a popular solution!
Step by step
Solved in 3 steps

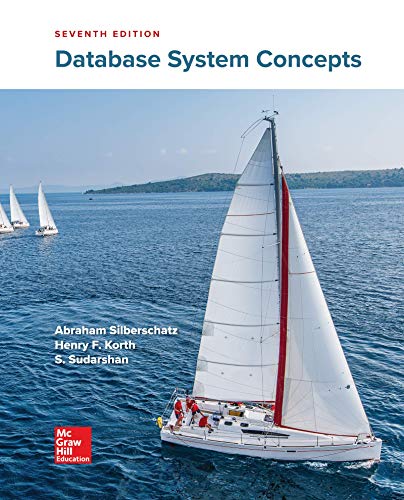
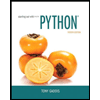
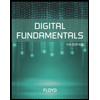
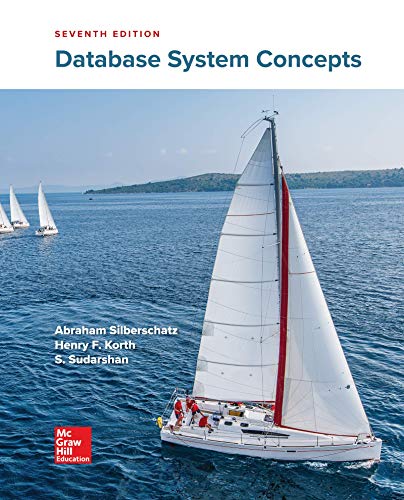
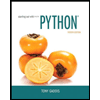
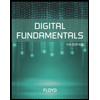
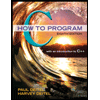
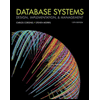
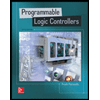