I am attempting to create a digital clock. However, I have run into an issue I am trying to create a method name findNormalizedHour which converts from a 24-hour format to a 12-hour format. This code is passing all of my tests except for it is allowing hours greater than 23 (it is supposed to throw an error) and it is allowing negative numbers (it is supposed to throw an error). How do I fix these two things? I would appreciate any assitance! So far this is what I have: public int findNormalizedHour(int hour) { // if hour is less than 12 but not equal to 0 if(hour<12 && hour != 0){ return hour; } if(hour>12) { return hour - 12; } if(hour == 12) { return hour; } if (hour>23 ) { throw new IllegalArgumentException("Invalid input caused by an hour greater than 23."); } if (hour < 0) { throw new IllegalArgumentException("Invalid input caused by a negative hour."); } // otherwise else{ return 12 - hour; } }
I am attempting to create a digital clock. However, I have run into an issue I am trying to create a method name findNormalizedHour which converts from a 24-hour format to a 12-hour format. This code is passing all of my tests except for it is allowing hours greater than 23 (it is supposed to throw an error) and it is allowing negative numbers (it is supposed to throw an error). How do I fix these two things? I would appreciate any assitance!
So far this is what I have:
public int findNormalizedHour(int hour) {
// if hour is less than 12 but not equal to 0
if(hour<12 && hour != 0){
return hour;
}
if(hour>12) {
return hour - 12;
}
if(hour == 12) {
return hour;
}
if (hour>23 ) {
throw new IllegalArgumentException("Invalid input caused by an hour greater than 23.");
}
if (hour < 0) {
throw new IllegalArgumentException("Invalid input caused by a negative hour.");
}
// otherwise
else{
return 12 - hour;
}
}

You need to keep them in try and catch block and last else is not needed all conditions you have already mentioned.
And i have some of lines of you for one case if the hour==12 you can include in first if condition
public int findNormalizedHour(int hour) {
// if hour is less than 12 but not equal to 0
if(hour<=12 && hour != 0){
return hour;
}
if(hour>12) {
return hour - 12;
}
if (hour>23 ) {
try{
throw new IllegalArgumentException("Invalid input caused by an hour greater than 23.");
}
catch(MyException e){
System.outr.println(e.getMessage());
}
}
if (hour < 0) {
try{
throw new IllegalArgumentException("Invalid input caused by a negative hour.");
}
catch(MyException e){
System.outr.println(e.getMessage());
}
}
// otherwise
else{
return 12 - hour;
}
}
Step by step
Solved in 2 steps

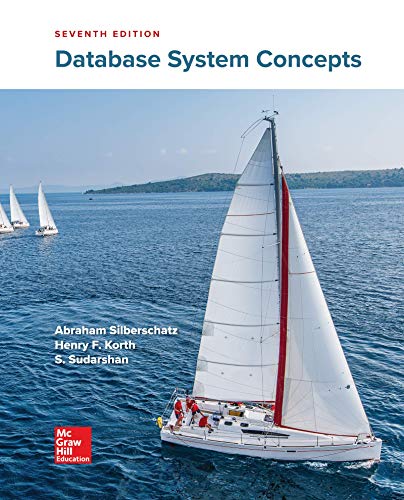
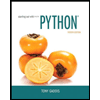
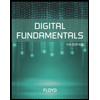
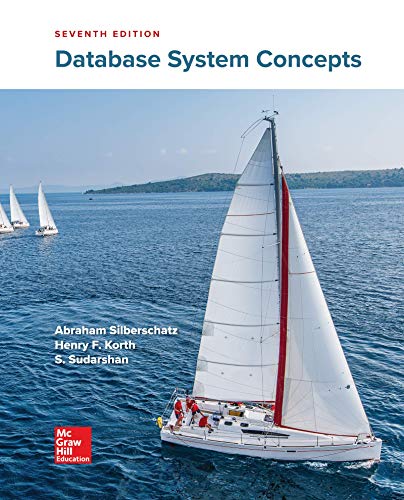
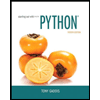
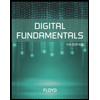
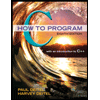
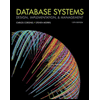
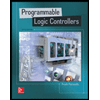