Write a java program called TempConvert.java that will contain many methods. The first method, called processData(), will be called from the main method. It will ask the user for a temperature value, the current temperature scale (Fahrenheit, Celsius or Kelvin), and a temperature scale to convert to (Fahrenheit, Celsius or Kelvin). This method will not return anything. The method header is below: public static void processData() The processData() method will then pass both values to a method, called checkValidity(), that will check to see if the temperature is within a valid range. The method will return true if the range is valid or false if it is not. The method header is: public static boolean checkValidity(double temp, String scale) Valid ranges for the temperatures are: Fahrenheit: -459.40 – 1000 degrees Celsius: -273 – 1000 degrees Kelvin: 0 – 1000 degrees The processData() method will then, if the value returned is true, call the appropriate conversion method. You will have a choice of six conversion methods to choose from. Here are the headers: public static double convertFahtoCel(double temp) public static double convertFahtoKel(double temp) public static double convertCeltoFah(double temp) public static double convertCeltoKel (double temp) public static double convertKeltoCel(double temp) public static double convertKeltoFah(double temp) The conversions needed are: Fahrenheit to Celsius: C = (F – 32) / (9/5) Celsius to Fahrenheit: F = (C * 9/5) + 32 Celsius to Kelvin: K = C + 273.15 • Kelvin to Celsius: C = K – 273.15 Each method will return a double temperature. If the current scale and scale to convert to are the same, then this step will be skipped by the processData() method. Once the conversions are completed or if no conversion was needed, the processData() method will then output the result of the conversion in the format specified below: 68 degrees Fahrenheit is 20 degrees Celsius or 68 degrees Fahrenheit is 68 degrees Fahrenheit If the conversions were determined to be not feasible, (This would happen if the checkValidity() method returned false.) the method will then output: -20 degrees Kelvin is not a valid temperature Once the output is displayed processData() should ask the question: Do you want to convert another temperature amount? If the answer is not “quit”, then processData() will loop again to convert another temperature amount. All outputs should be rounded to two decimal spaces using printf(). Below is a sample run. Enter the temperature to convert: 68.5 Enter the current scale of the temperature: Fahrenheit Enter the scale you want to convert the temperature to: Kelvin 68.50 degrees Fahrenheit is 293.4278 degrees Kelvin Would you like to convert another temperature? Enter quit to exit. yes Enter the temperature to convert: -20 Enter the current scale of the temperature: Kelvin Enter the scale you want to convert the temperature to: Fahrenheit -20 degrees Kelvin is not a valid temperature Would you like to convert another temperature? Enter quit to exit. quit
Write a java program called TempConvert.java that will contain many methods. The first method, called processData(), will be called from the main method. It will ask the user for a temperature value, the current temperature scale (Fahrenheit, Celsius or Kelvin), and a temperature scale to convert to (Fahrenheit, Celsius or Kelvin). This method will not return anything. The method header is below:
public static void processData()
The processData() method will then pass both values to a method, called checkValidity(), that will check to see if the temperature is within a valid range. The method will return true if the range is valid or false if it is not. The method header is:
public static boolean checkValidity(double temp, String scale)
Valid ranges for the temperatures are:
-
Fahrenheit: -459.40 – 1000 degrees
-
Celsius: -273 – 1000 degrees
-
Kelvin: 0 – 1000 degrees
The processData() method will then, if the value returned is true, call the appropriate conversion method. You will have a choice of six conversion methods to choose from. Here are the headers:
public static double convertFahtoCel(double temp) public static double convertFahtoKel(double temp) public static double convertCeltoFah(double temp) public static double convertCeltoKel (double temp) public static double convertKeltoCel(double temp) public static double convertKeltoFah(double temp)The conversions needed are:
-
Fahrenheit to Celsius: C = (F – 32) / (9/5)
-
Celsius to Fahrenheit: F = (C * 9/5) + 32
-
Celsius to Kelvin: K = C + 273.15
• Kelvin to Celsius: C = K – 273.15
Each method will return a double temperature. If the current scale and scale to convert
to are the same, then this step will be skipped by the processData() method.
Once the conversions are completed or if no conversion was needed, the processData() method will then output the result of the conversion in the format specified below:
68 degrees Fahrenheit is 20 degrees Celsius or
68 degrees Fahrenheit is 68 degrees Fahrenheit
If the conversions were determined to be not feasible, (This would happen if the checkValidity() method returned false.) the method will then output:
-20 degrees Kelvin is not a valid temperature
Once the output is displayed processData() should ask the question: Do you want to convert another temperature amount?
If the answer is not “quit”, then processData() will loop again to convert another temperature amount.
All outputs should be rounded to two decimal spaces using printf(). Below is a sample run.
Enter the temperature to convert:
68.5
Enter the current scale of the temperature:
Fahrenheit
Enter the scale you want to convert the temperature to:
Kelvin
68.50 degrees Fahrenheit is 293.4278 degrees Kelvin
Would you like to convert another temperature? Enter quit to exit.
yes
Enter the temperature to convert:
-20
Enter the current scale of the temperature:
Kelvin
Enter the scale you want to convert the temperature
to:
Fahrenheit
-20 degrees Kelvin is not a valid temperature
Would you like to convert another temperature? Enter quit to exit.
quit

Step by step
Solved in 3 steps with 1 images

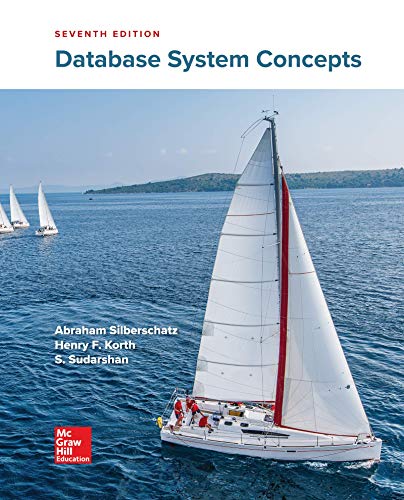
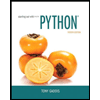
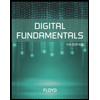
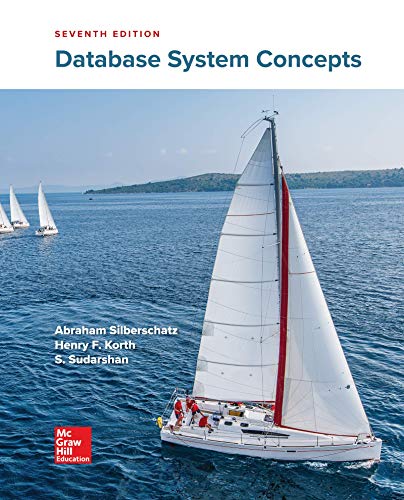
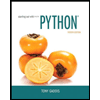
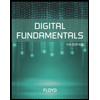
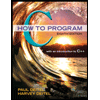
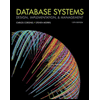
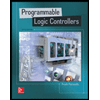