How will I use Tryparse() method in C# to ensure that each piece of the user's entry data is in the correct type and prompt the user to reenter the data when the data is not valid using a while loop? So the Tryparse() method statement should be where there is a WriteLine asking a user to enter data. Thank you. using static System.Console; using System.Globalization; class GreenvilleRevenue { static void Main() { int numLastYear; int numThisYear; double revenue; int fee = 25; const int MAX_CONTESTANTS = 30; string[] names = new string[MAX_CONTESTANTS]; char[] talents = new char[MAX_CONTESTANTS]; char[] talentCodes = { 'S', 'D', 'M', 'O' }; string[] talentCodesStrings = { "Singing", "Dancing", "Musical instrument", "Other" }; int[] counts = { 0, 0, 0, 0 }; ; numLastYear = GetContestantNumber("last", 0, 30); numThisYear = GetContestantNumber("this", 0, 30); WriteLine("Last year's competition had {0} contestants, and this year's has {1} contestants", numLastYear, numThisYear); revenue = (numThisYear * fee); WriteLine("Revenue expected this year is {0}", revenue.ToString("C", CultureInfo.GetCultureInfo("en-US"))); DisplayRelationship(numThisYear, numLastYear); GetContestantData(numThisYear, names, talents, talentCodes, talentCodesStrings, counts); GetLists(numThisYear, talentCodes, talentCodesStrings, names, talents, counts); } public static int GetContestantNumber(string when, int min, int max) { int contestants; string contestantString; WriteLine("Enter number of contestants {0} year >> ", when); contestantString = ReadLine(); while(! int.TryParse(contestantString, out contestants)) { WriteLine("Invalid"); WriteLine("Reenter the valid constestants number"); contestantString = ReadLine(); } return contestants; } public static void DisplayRelationship( int numThisYear2, int numLastYear2) { if (numThisYear2 > 2 * numLastYear2) WriteLine("The competition is more than twice as big this year!"); else if (numThisYear2 > numLastYear2) WriteLine("The competition is bigger than ever!"); else if (numThisYear2 < numLastYear2) WriteLine("A tighter race this year! Come out and cast your vote!"); } public static void GetContestantData(int numThisYear, string[] names, char[] talents, char[] talentCodes, string[] talentCodesStrings, int[] counts) { int x = 0; bool isValid; string talentString; while (x < numThisYear) { Write("Enter contestant name >> "); names[x] = Console.ReadLine(); WriteLine("Talent codes are:"); for (int y = 0; y < talentCodes.Length; ++y) WriteLine(" {0} {1}", talentCodes[y], talentCodesStrings[y]); Write(" Enter talent code >> "); talentString = ReadLine(); while(! char.TryParse(talentString, out talents[x])) { WriteLine(" Invalid format - entry must be a single character"); WriteLine("That is not a valid code"); Write(" Enter talent code >> "); talentString = ReadLine();} talents[x] = GetChar(); isValid = false; while (!isValid) {for (int z = 0; z < talentCodes.Length; ++z) { if (talents[x] == talentCodes[z]) {isValid = true; ++counts[z]; } } if (!isValid) {WriteLine("{0} is not a valid code", talents[x]); Write(" Enter talent code >> "); talents[x] = GetChar(); } } ++x; } } public static void GetLists(int numThisYear, char[] talentCodes, string[] talentCodesStrings, string[] names, char[] talents, int[] counts) {int x; char QUIT = 'Z'; char option; bool isValid; int pos = 0; bool found; WriteLine("\nThe types of talent are:"); for (x = 0; x < counts.Length; ++x) WriteLine("{0, -20} {1, 5}", talentCodesStrings[x], counts[x]); WriteLine("\nEnter a talent type or {0} to quit >> ", QUIT); option = GetChar(); while (option != QUIT) { isValid = false; for (int z = 0; z < talentCodes.Length; ++z) {if (option == talentCodes[z]) { isValid = true; pos = z; } } if (!isValid) WriteLine("{0} is not a valid code", option); else { WriteLine("\nContestants with talent {0} are:", talentCodesStrings[pos]); found = false; for (x = 0; x < numThisYear; ++x) { if (talents[x] == option) {WriteLine(names[x]); found = true;} } if (!found) Console.WriteLine("No contestants had talent {0}", talentCodesStrings[pos]); } WriteLine("\nEnter a talent type or {0} to quit >> ", QUIT); option = GetChar(); } } private static char GetChar() {char validChar = '0'; bool isValidChar = false; var charInput = string.Empty; while (!isValidChar) { charInput = Console.ReadLine(); isValidChar = Char.TryParse(charInput, out validChar); if (!isValidChar) { WriteLine("Invalid character - Please try again by entering one character!"); /* WriteLine("Invalid format - entry must be a single character"); } else WriteLine("That is not a valid code");*/ } } return validChar;- } }
How will I use Tryparse() method in C# to ensure that each piece of the user's entry data is in the correct type and prompt the user to reenter the data when the data is not valid using a while loop?
So the Tryparse() method statement should be where there is a WriteLine asking a user to enter data. Thank you.
using static System.Console;
using System.Globalization;
class GreenvilleRevenue
{
static void Main()
{
int numLastYear;
int numThisYear;
double revenue;
int fee = 25;
const int MAX_CONTESTANTS = 30;
string[] names = new string[MAX_CONTESTANTS];
char[] talents = new char[MAX_CONTESTANTS];
char[] talentCodes = { 'S', 'D', 'M', 'O' };
string[] talentCodesStrings = { "Singing", "Dancing", "Musical instrument", "Other" };
int[] counts = { 0, 0, 0, 0 }; ;
numLastYear = GetContestantNumber("last", 0, 30);
numThisYear = GetContestantNumber("this", 0, 30);
WriteLine("Last year's competition had {0} contestants, and this year's has {1} contestants", numLastYear, numThisYear);
revenue = (numThisYear * fee);
WriteLine("Revenue expected this year is {0}", revenue.ToString("C", CultureInfo.GetCultureInfo("en-US")));
DisplayRelationship(numThisYear, numLastYear);
GetContestantData(numThisYear, names, talents, talentCodes, talentCodesStrings, counts);
GetLists(numThisYear, talentCodes, talentCodesStrings, names, talents, counts);
}
public static int GetContestantNumber(string when, int min, int max)
{
int contestants;
string contestantString;
WriteLine("Enter number of contestants {0} year >> ", when);
contestantString = ReadLine();
while(! int.TryParse(contestantString, out contestants))
{
WriteLine("Invalid");
WriteLine("Reenter the valid constestants number");
contestantString = ReadLine();
}
return contestants;
}
public static void DisplayRelationship( int numThisYear2, int numLastYear2)
{ if (numThisYear2 > 2 * numLastYear2)
WriteLine("The competition is more than twice as big this year!");
else
if (numThisYear2 > numLastYear2)
WriteLine("The competition is bigger than ever!");
else
if (numThisYear2 < numLastYear2)
WriteLine("A tighter race this year! Come out and cast your vote!"); }
public static void GetContestantData(int numThisYear, string[] names, char[] talents, char[] talentCodes, string[] talentCodesStrings, int[] counts)
{ int x = 0;
bool isValid;
string talentString;
while (x < numThisYear)
{ Write("Enter contestant name >> ");
names[x] = Console.ReadLine();
WriteLine("Talent codes are:");
for (int y = 0; y < talentCodes.Length; ++y)
WriteLine(" {0} {1}", talentCodes[y], talentCodesStrings[y]);
Write(" Enter talent code >> ");
talentString = ReadLine();
while(! char.TryParse(talentString, out talents[x]))
{ WriteLine(" Invalid format - entry must be a single character");
WriteLine("That is not a valid code");
Write(" Enter talent code >> ");
talentString = ReadLine();}
talents[x] = GetChar();
isValid = false;
while (!isValid)
{for (int z = 0; z < talentCodes.Length; ++z)
{
if (talents[x] == talentCodes[z])
{isValid = true;
++counts[z];
}
}
if (!isValid)
{WriteLine("{0} is not a valid code", talents[x]);
Write(" Enter talent code >> ");
talents[x] = GetChar();
}
}
++x;
}
}
public static void GetLists(int numThisYear, char[] talentCodes, string[] talentCodesStrings, string[] names, char[] talents, int[] counts)
{int x;
char QUIT = 'Z';
char option;
bool isValid;
int pos = 0;
bool found;
WriteLine("\nThe types of talent are:");
for (x = 0; x < counts.Length; ++x)
WriteLine("{0, -20} {1, 5}", talentCodesStrings[x], counts[x]);
WriteLine("\nEnter a talent type or {0} to quit >> ", QUIT);
option = GetChar();
while (option != QUIT)
{ isValid = false;
for (int z = 0; z < talentCodes.Length; ++z)
{if (option == talentCodes[z])
{ isValid = true;
pos = z;
}
}
if (!isValid)
WriteLine("{0} is not a valid code", option);
else
{ WriteLine("\nContestants with talent {0} are:", talentCodesStrings[pos]);
found = false;
for (x = 0; x < numThisYear; ++x)
{ if (talents[x] == option)
{WriteLine(names[x]);
found = true;}
}
if (!found)
Console.WriteLine("No contestants had talent {0}", talentCodesStrings[pos]);
}
WriteLine("\nEnter a talent type or {0} to quit >> ", QUIT);
option = GetChar();
}
}
private static char GetChar()
{char validChar = '0';
bool isValidChar = false;
var charInput = string.Empty;
while (!isValidChar)
{ charInput = Console.ReadLine();
isValidChar = Char.TryParse(charInput, out validChar);
if (!isValidChar)
{
WriteLine("Invalid character - Please try again by entering one character!");
/* WriteLine("Invalid format - entry must be a single character");
}
else
WriteLine("That is not a valid code");*/
}
}
return validChar;-
}
}

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

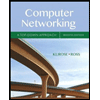
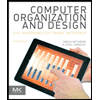
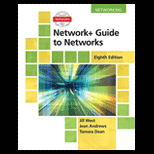
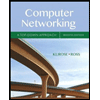
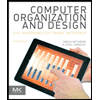
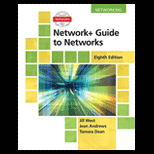
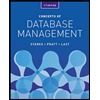
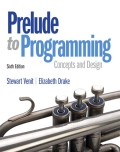
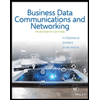