How to write down the Junit test? My goal is to test each method ( reverse and getReserve). Can we write down the Junit Test for the void method?How to test reverse? Here's the code: public class ArrayController { public static void reverse(int[] array1, int[] array2){ for (int i = 0; i
How to write down the Junit test? My goal is to test each method ( reverse and getReserve).
Can we write down the Junit Test for the void method?How to test reverse?
Here's the code:
public class ArrayController {
public static void reverse(int[] array1, int[] array2){
for (int i = 0; i <array1.length;i++){
array2[i] = array1[array1.length - i - 1];
}
}
public static int[] getReverse(int[] array){
// in which it reverses the array passed in the argument and returns this array
int[] temp = new int[array.length];
reverse(array,temp);
System.out.println(Arrays.toString(array));
System.arraycopy(temp, 0, array, 0, array.length);
return array;
}
public static void main(String[] args) {System.out.println();
int[] array1 = {1,2,3,4};
int[] reversed = getReverse(array1);
System.out.println(Arrays.toString(reversed));//display array to the compiler
}
}
Junit-Testing for getReverse:
import static controllers.ArrayController.getReverse;
import static org.junit.jupiter.api.Assertions.*;
import org.junit.jupiter.api.Test;
import java.util.Arrays;
@Test
void testGetReverse(){
int[] array1 = {8, 1, 12, 9, 5};
int[] array2 = {5, 9, 12, 1, 8, 6, 4};
int[] array3 = {5, 9, 12, 1, 8};
int[] array4 = {5, 9, 12, 1, 8};
int[] array5 = {6, 3, 12, 1, 8};
assertTrue(Arrays.equals(getReverse(array1),array3));
assertFalse(Arrays.equals(getReverse(array1),array2));
assertFalse(Arrays.equals(getReverse(array5),array4));
}
}

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

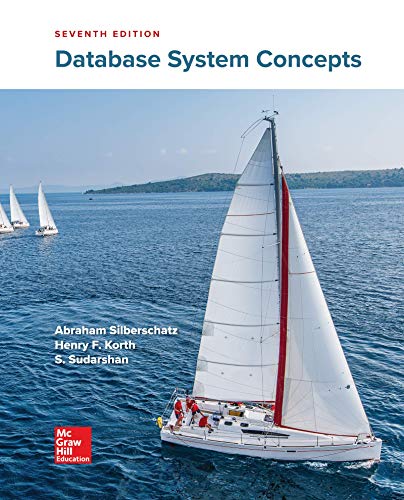
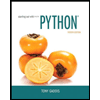
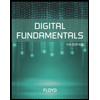
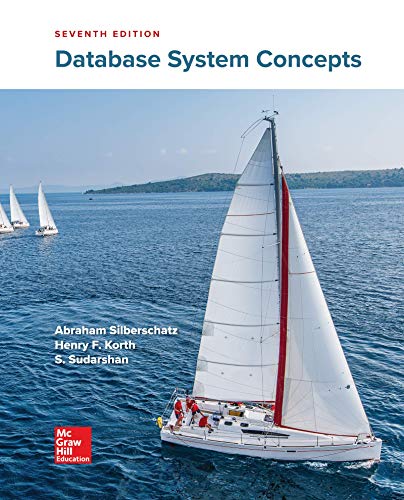
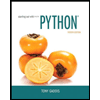
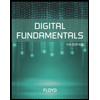
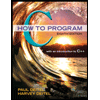
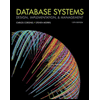
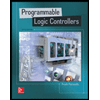