How many passports will we be creating today? 2 Please input ID of passport 1: 15625 What is the gender? M First Name? (end with a dot)Kyle. Last Name? (end with a dot)Smith. Address? (end with a dot)123 Lead Ave SE. Please input ID of passport 2: 15626 What is the gender? F First Name? (end with a dot)Clair. Last Name? (end with a dot)Smith. Address? (end with a dot)1059 Dr MLK Jr NE. Info of User 1: ID: 15625 Gender: M First Name: Kyle Last Name: Smith Address: 123LeadAveSE Info of User 2: ID: 15626 Gender: F First Name: Clair Last Name: Smith Address: 1059DrMLKJrNE
First, you should ask how many passports will be issued.
Then, ask for the first name, last name, address, passport ID and gender of each passport.
Lastly, print out the information.
Follow some guidelines when writing your code: You should have one structure definition with the following members:
· FirstName: should be a character array, accepting at most 100 characters
· LastName: should be a character array, accepting at most 100 characters
· Address: should also be a char array, accepting at most 100 characters
· PassportID: should be an integer
· Gender: should be a single character
Please without using #include<stdlib.h>
Just with #include<stdio.h> please
The code has to have at least one structure definition and five different functions (including main).
Example of the output:


The program requires to ask user for their information to create the passports. The program uses structure to store the information of each person.
The following points are important to be able to write this program:
- Include header file stdio.h for using scanf() and printf() functions.
- In this program, two functions have been defined:
- Function getinformation() is used to ask the user for the number of passports to be entered. The user then enters value for the passport.
- Function print() is used to print all the information stored in the array of the structure.
- The main() function , creates a pointer to the structure, and then calls the function getinformation() and print().
- In the structure Person, there are following entities:
- FirstName
- LastName
- Address
- PassportID
- Gender
Program:
#include <stdio.h>
//structure Person
struct Person
{
//char array for storing firstname
char firstname[100];
//char array for storing lastname
char lastname[100];
//char array for storing address
char address[100];
//int variable to store passport ID
int passID;
//char variable to store gender
char gender;
};
//function that inputs values of the persons
//return pointer to the array of persons
struct Person * getinformation(int *size)
{
int num;
//memory buffer
char fname[100],lastname[100],add[100];
int ID;
char g;
//iterator variable
int i=0;
//ask user to input number of passports
printf("How many passports will we be creating today?\n");
//input number of passports
scanf("%d",&num);
//pointer to structure of Person
struct Person * info;
//loop until number of passports
for(i=0;i<num;i++)
{
//input passport ID
printf("Please input ID of passport %d ",i+1);
scanf(" %d",&info[i].passID);
//input gender
printf("What is the gender? ");
scanf(" %c",&info[i].gender);
//input first name
printf("First Name? (end with a dot) ");
scanf(" %[^.]%*c",info[i].firstname);
//input last name
printf("Last Name? (end with a dot) ");
scanf(" %[^.]%*c",info[i].lastname);
//input address
printf("Address? (end with a dot) ");
scanf(" %[^.\n]%*c",info[i].lastname);
}
//size of array referenced
*size = num;
//return pointer to the array of structure
return info;
}
//function to print the information
void print(struct Person * p,int len)
{
//iterator variable
int i;
//loop until length of passport array
for(i=0;i<len;i++){
//print all info
printf("Info of user %d",i+1);
printf("ID of passport : ");
printf("%d\n",p[i].passID);
printf(" Gender: ");
printf(" %c\n",p[i].gender);
printf(" First Name: ");
printf(" %s\n",p[i].firstname);
printf(" Last Name: ");
printf(" %s\n",p[i].lastname);
printf(" Address: ");
printf(" %s\n",p[i].address);
}
}
//main() function
int main()
{
//variable to store size
int size=0;
//pointer to
struct Person *A;
A = getinformation(&size);
print(A,size);
}
Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

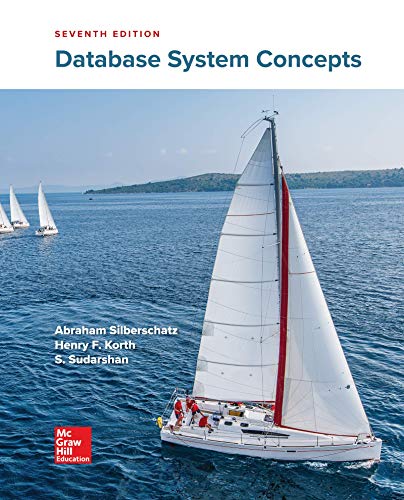
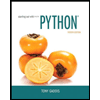
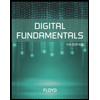
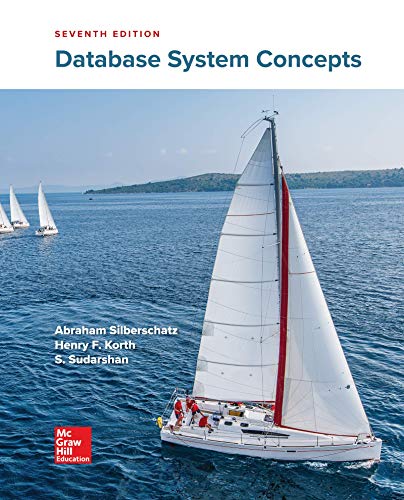
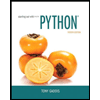
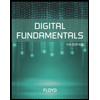
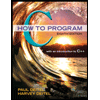
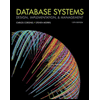
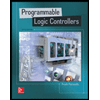