How do you generate all the permutations of a list in the C program for the problem: "In how many different orders can five runners finish a race if no ties are allowed?". This is what I have gotten so far but not yet corrected, please help me to fix and finish the code: #include #define MAX 200 int x[MAX]; int n, k; void Print() { int i; for (i = 1; i <= k; i++) { printf("%6d", x[i]); printf("\n"); } return; } void Try(int start) { int i, j; int temp; for (i = x[start - 1]; i < n; i++) { for (j = i + 1; j < n - 1; j++) { if (x[i] > x[j]) { temp = x[i]; x[i] = x[j]; x[j] = temp; } if (x[i] < x[j]) { temp = x[j]; x[j] = x[i]; x[i] = temp; } } } Print(); return; } int main() { n = 5; k = 5; x[0] = 0; Try(1); return 0; } The following is the example of the output of another problem:
How do you generate all the permutations of a list in the C program for the problem: "In how many different orders can five runners finish a
race if no ties are allowed?". This is what I have gotten so far but not yet corrected, please help me to fix and finish the code:
#include <stdio.h>
#define MAX 200
int x[MAX];
int n, k;
void Print()
{
int i;
for (i = 1; i <= k; i++)
{
printf("%6d", x[i]);
printf("\n");
}
return;
}
void Try(int start)
{
int i, j;
int temp;
for (i = x[start - 1]; i < n; i++) {
for (j = i + 1; j < n - 1; j++) {
if (x[i] > x[j]) {
temp = x[i];
x[i] = x[j];
x[j] = temp;
}
if (x[i] < x[j]) {
temp = x[j];
x[j] = x[i];
x[i] = temp;
}
}
}
Print();
return;
}
int main()
{
n = 5;
k = 5;
x[0] = 0;
Try(1);
return 0;
}
The following is the example of the output of another problem:
![permutations ( [1, 2, 3])
[1, 2, 3]
[1, 3, 2]
[2, 1, 3]
[2, 3, 1]
[3, 1, 2]
[3, 2, 1]](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fae4b0d98-45d8-473d-ad4c-873f648738cd%2F684a6b2e-bb95-476a-a53c-363fde4ac982%2Fm1hecsk_processed.png&w=3840&q=75)

Step by step
Solved in 2 steps with 1 images

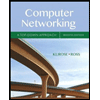
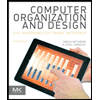
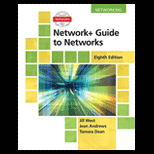
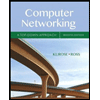
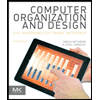
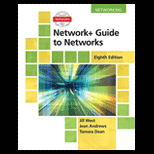
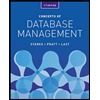
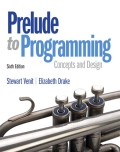
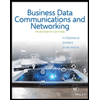