How do I start creating a program to shuffle and deal a deck of cards? The program should consist of class Card. The implementation code for the following class is: class DeckOfCards{public: DeckOfCards(); // constructor initializes deck void shuffle(); // shuffles cards in deck Card dealCard(); // deals cards in deck bool moreCards() const; // are there any more cards leftprivate: std::vector< Card > deck; // represents deck of cards unsigned currentCard; // index of next card to be dealt}; // end class DeckOfCards Class Card should provide: a) Data members face and suit -- use enumerations to represent the faces and suits. b) A constructor that receives two enumeration constants representating the face and suit and uses them to initialize the data members. c) Two static arrays of strings representing the faces and suits. d) A toString function that returns the Card as a string in the form "face of suit." You can use the + operator to concatenate strings.
How do I start creating a program to shuffle and deal a deck of cards?
The program should consist of class Card.
The implementation code for the following class is:
class DeckOfCards
{
public:
DeckOfCards(); // constructor initializes deck
void shuffle(); // shuffles cards in deck
Card dealCard(); // deals cards in deck
bool moreCards() const; // are there any more cards left
private:
std::
unsigned currentCard; // index of next card to be dealt
}; // end class DeckOfCards
Class Card should provide:
a) Data members face and suit -- use enumerations to represent the faces and suits.
b) A constructor that receives two enumeration constants representating the face and suit and uses them to initialize the data members.
c) Two static arrays of strings representing the faces and suits.
d) A toString function that returns the Card as a string in the form "face of suit." You can use the + operator to concatenate strings.

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 1 images

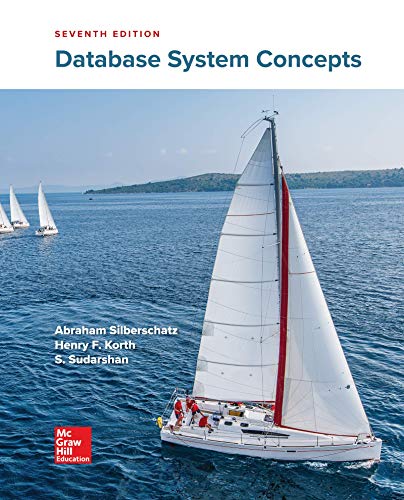
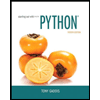
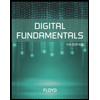
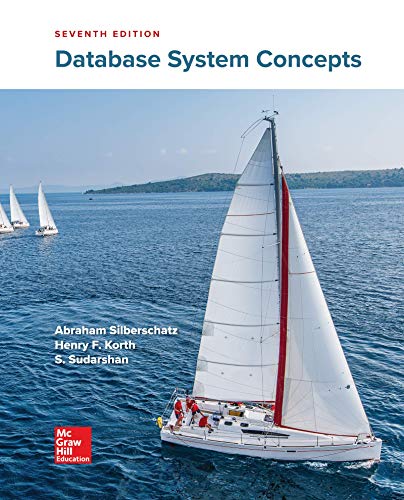
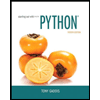
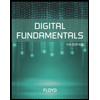
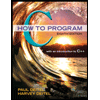
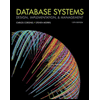
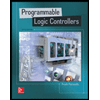