How do I handle the ArrayIndexOutOfBoundsException using standard error? Desired Output: MyCat: There is no input. Output: Exception in thread "main" java.lang.ArrayIndexOutOfBoundsException: Index 0 out of bounds for length 0 at cs1302.exceptions.MyCat.main(MyCat.java:22) Code: package cs1302.exceptions; import java.util.Scanner; import java.io.File; import java.io.FileNotFoundException; import cs1302.exceptions.Printer; /** * A simpler version of the Unix cat command. */ public class MyCat { /** * Entry point for the application. Exactly zero or one command-line arguments are expected. * If a filename is given as an argument, then the program should print the contents of that * file to standard output. If a single dash (i.e., "-") is given as an argument, then * the program should print the contents of standard input. * * @param args the command-line arguments */ public static void main(String[] args) { try { String filename = args[0]; Scanner input = null; if (filename.equals("-")) { Printer.printStdInLines(); } else { File file = new File(filename); Printer.printFileLines(file); } // if } catch (FileNotFoundException fnfe) { System.err.println("Sorry, we cannot find the file..."); System.err.println(fnfe); } catch (ArrayIndexOutOfBoundsException e) { System.err.println("MyCat: There is no input."); System.err.println(e); } } // Main Method } // MyCat
How do I handle the ArrayIndexOutOfBoundsException using standard error?
Desired Output:
MyCat: There is no input.
Output:
Exception in thread "main" java.lang.ArrayIndexOutOfBoundsException: Index 0 out of bounds for length 0
at cs1302.exceptions.MyCat.main(MyCat.java:22)
Code:
package cs1302.exceptions;
import java.util.Scanner;
import java.io.File;
import java.io.FileNotFoundException;
import cs1302.exceptions.Printer;
/**
* A simpler version of the Unix <code>cat</code> command.
*/
public class MyCat {
/**
* Entry point for the application. Exactly zero or one command-line arguments are expected.
* If a filename is given as an argument, then the
* file to standard output. If a single dash (i.e., "-") is given as an argument, then
* the program should print the contents of standard input.
*
* @param args the command-line arguments
*/
public static void main(String[] args) {
try {
String filename = args[0];
Scanner input = null;
if (filename.equals("-")) {
Printer.printStdInLines();
} else {
File file = new File(filename);
Printer.printFileLines(file);
} // if
} catch (FileNotFoundException fnfe) {
System.err.println("Sorry, we cannot find the file...");
System.err.println(fnfe);
} catch (ArrayIndexOutOfBoundsException e) {
System.err.println("MyCat: There is no input.");
System.err.println(e);
}
} // Main Method
} // MyCat

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

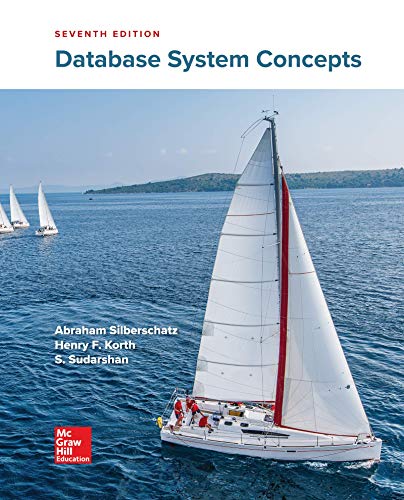
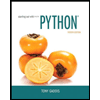
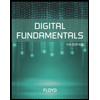
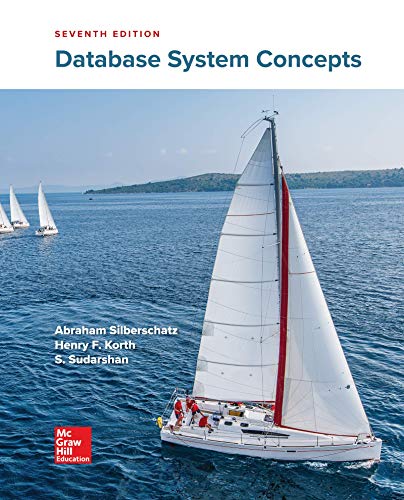
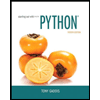
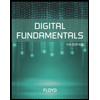
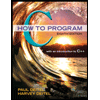
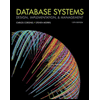
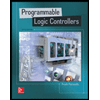