How do I fix the issue? Write a public static method named odds that takes in 1 argument int a, and returns a String containing all positive odd numbers, with each separated by a comma, from 1 up to that number inclusive if it is also odd. Remember if the argument is even not to include it in the output. Remember there should be no trailing comma after the values. If the argument a is negative, return a String that says “NONE” (Do not print the String) Example: odds(5); String returned by method: “1,3,5” Example: odds(8); String returned by method: “1,3,5,7” Example: odds(9); String returned by method: “1,3,5,7,9” Example: odds(-5); String returned by method: “NONE” Example: odds(0); String returned by method: “” Example: odds(1); String returned by method: “1” public class MidtermProblems{ public static String odds(int a){ if(a<0) return "NONE"; String s=""; for(int i=0;i<=a;i++){ if(i%2==0) s+=i+" "; } return s.substring(0,s.length()-1); } public static void main(String[] args) { System.out.println(odds(5)); System.out.println(odds(8)); System.out.println(odds(9)); System.out.println(odds(-5)); System.out.println(odds(0)); System.out.println(odds(1)); } }
How do I fix the issue?
Write a public static method named odds that takes in 1 argument int a, and returns a String containing all positive odd numbers, with each separated by a comma, from 1 up to that number inclusive if it is also odd.
Remember if the argument is even not to include it in the output.
Remember there should be no trailing comma after the values.
If the argument a is negative, return a String that says “NONE”
(Do not print the String) Example: odds(5); String returned by method: “1,3,5” Example: odds(8); String returned by method: “1,3,5,7” Example: odds(9); String returned by method: “1,3,5,7,9” Example: odds(-5); String returned by method: “NONE” Example: odds(0); String returned by method: “” Example: odds(1); String returned by method: “1”
public class MidtermProblems{
public static String odds(int a){
if(a<0)
return "NONE";
String s="";
for(int i=0;i<=a;i++){
if(i%2==0)
s+=i+" ";
}
return s.substring(0,s.length()-1);
}
public static void main(String[] args) {
System.out.println(odds(5));
System.out.println(odds(8));
System.out.println(odds(9));
System.out.println(odds(-5));
System.out.println(odds(0));
System.out.println(odds(1));
}
}


Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 2 images

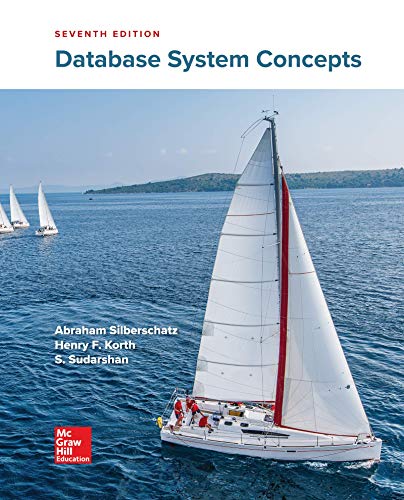
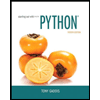
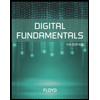
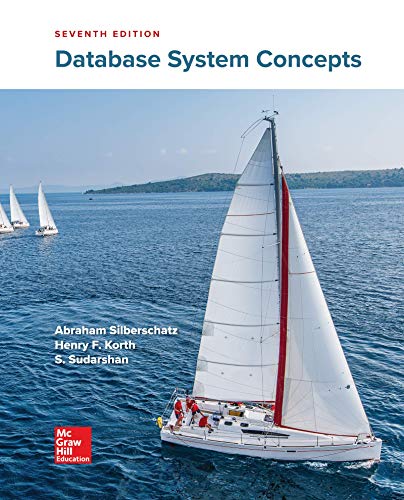
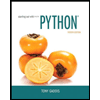
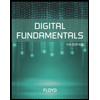
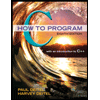
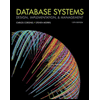
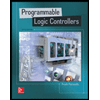