How do I change numCastMembers retrieved to 1 to numCastMembers retrieved to 0? Test: OK --- method getNumMinutes exists OK --- method getNumCastMembers exists OK --- method getMovieName exists OK --- method isKidFriendly exists OK --- method getCastMembers exists OK --- movieName retrieved Flick OK --- numMinutes retrieved to 0 OK --- isKidFriendly retrieved to false OK --- numCastMembers retrieved to 1 OK --- getCastMembers returns copy of the original array with the same content OK --- movieName retrieved Tape OK --- numMinutes retrieved to 10 OK --- isKidFriendly retrieved to true OK --- numCastMembers retrieved to 3 OK --- getCastMembers returns copy of the original array with the same content OK --- movieName retrieved Screening OK --- numMinutes retrieved to 120 OK --- isKidFriendly retrieved to false OK --- numCastMembers retrieved to 5 OK --- getCastMembers returns copy of the original array with the same content OK --- movieName retrieved Film OK --- numMinutes retrieved to 144 OK --- isKidFriendly retrieved to true OK --- numCastMembers retrieved to 2 OK --- getCastMembers returns copy of the original array with the same content OK --- movieName retrieved Never Ending Story OK --- numMinutes retrieved to 321 OK --- isKidFriendly retrieved to true OK --- numCastMembers retrieved to 8 OK --- getCastMembers returns copy of the original array with the same content SUCCESS --- passed getter methods tests Code: import java.util.*; import java.util.Arrays; public class Movie { private String movieName; private int numMinutes; private boolean isKidFriendly; private int numCastMembers; private String[] castMembers; // default constructor public Movie() { this.movieName = "Flick"; this.numMinutes = 0; this.isKidFriendly = false; this.numCastMembers = 0; this.castMembers = new String[10]; } // overloaded parameterized constructor public Movie(String movieName, int numMinutes, boolean isKidFriendly, String[] castMembers) { this.movieName = movieName; this.numMinutes = numMinutes; this.isKidFriendly = isKidFriendly; this.numCastMembers = castMembers.length; this.castMembers = new String[numCastMembers]; for (int i = 0; i < castMembers.length; i++) { this.castMembers[i] = castMembers[i]; } } // set the number of minutes public void setNumMinutes(int numMinutes) { this.numMinutes = numMinutes; } // set the movie name public void setMovieName(String movieName)
How do I change numCastMembers retrieved to 1 to numCastMembers retrieved to 0?
Test:
OK --- method getNumMinutes exists
OK --- method getNumCastMembers exists
OK --- method getMovieName exists
OK --- method isKidFriendly exists
OK --- method getCastMembers exists
OK --- movieName retrieved Flick
OK --- numMinutes retrieved to 0
OK --- isKidFriendly retrieved to false
OK --- numCastMembers retrieved to 1
OK --- getCastMembers returns copy of the original array with the same content
OK --- movieName retrieved Tape
OK --- numMinutes retrieved to 10
OK --- isKidFriendly retrieved to true
OK --- numCastMembers retrieved to 3
OK --- getCastMembers returns copy of the original array with the same content OK --- movieName retrieved Screening
OK --- numMinutes retrieved to 120
OK --- isKidFriendly retrieved to false
OK --- numCastMembers retrieved to 5
OK --- getCastMembers returns copy of the original array with the same content OK --- movieName retrieved Film
OK --- numMinutes retrieved to 144
OK --- isKidFriendly retrieved to true
OK --- numCastMembers retrieved to 2
OK --- getCastMembers returns copy of the original array with the same content
OK --- movieName retrieved Never Ending Story
OK --- numMinutes retrieved to 321
OK --- isKidFriendly retrieved to true
OK --- numCastMembers retrieved to 8
OK --- getCastMembers returns copy of the original array with the same content
SUCCESS --- passed getter methods tests
Code:
import java.util.*;
import java.util.Arrays;
public class Movie
{
private String movieName;
private int numMinutes;
private boolean isKidFriendly;
private int numCastMembers;
private String[] castMembers;
// default constructor
public Movie()
{
this.movieName = "Flick";
this.numMinutes = 0;
this.isKidFriendly = false;
this.numCastMembers = 0;
this.castMembers = new String[10];
}
// overloaded parameterized constructor
public Movie(String movieName, int numMinutes, boolean isKidFriendly, String[] castMembers)
{
this.movieName = movieName;
this.numMinutes = numMinutes;
this.isKidFriendly = isKidFriendly;
this.numCastMembers = castMembers.length;
this.castMembers = new String[numCastMembers];
for (int i = 0; i < castMembers.length; i++)
{
this.castMembers[i] = castMembers[i];
}
}
// set the number of minutes
public void setNumMinutes(int numMinutes)
{
this.numMinutes = numMinutes;
}
// set the movie name
public void setMovieName(String movieName)
{
this.movieName = movieName;
}
// set if the movie is kid friendly or not
public void setIsKidFriendly(boolean isKidFriendly)
{
this.isKidFriendly = isKidFriendly;
}
// return the movie name
public String getMovieName()
{
return this.movieName;
}
// return the number of minutes
public int getNumMinutes()
{
return this.numMinutes;
}
// return true if movie is kid friendly else false
public boolean isKidFriendly()
{
return this.isKidFriendly;
}
// return the array of cast members
public String[] getCastMembers()
{
// create a deep copy of the array
String[] copyCastMembers = new String[this.castMembers.length];
// copy the strings from the array to the copy
for (int i = 0; i < this.castMembers.length; i++)
{
copyCastMembers[i] = this.castMembers[i];
}
return copyCastMembers;
}
// return the number of cast members
public int getNumCastMembers()
{
return this.numCastMembers;
}
// method that allows the name of a castMember at an index in the castMembers
// array to be changed
public boolean replaceCastMember(int index, String castMemberName)
{
if (index < 0 || index >= numCastMembers)
return false;
castMembers[index] = castMemberName;
return true;
}
// method that determines the equality of two String arrays and returns a
// boolean, by comparing the value at each index location.
// Return true if all elements of both arrays match, return false if there is
// any mismatch.
public boolean doArraysMatch(String[] arr1, String[] arr2)
{
if (arr1 == null && arr2 == null)
return true;
else if (arr1 == null || arr2 == null) // one of the array is null
return false;
else if (arr1.length != arr2.length) // length of arrays do not match
return false;
for (int i = 0; i < arr1.length; i++)
{
if (!arr1[i].equalsIgnoreCase(arr2[i]))
return false;
}
return true;
}
public String getCastMemberNamesAsString()
{
if (numCastMembers == 0)
{
return "none";
}
String names = castMembers[0];
for (int i = 1; i < numCastMembers; i++)
{
names += ", " + castMembers[i];
}
return names;
}
public String toString() {
String movie = "Movie: [ Minutes "+String.format("%03d", numMinutes)+" | Movie Name: %21s |";
if (isKidFriendly)
movie +=" is kid friendly |";
else
movie +=" not kid friendly |";
movie +=" Number of Cast Members: "+ numCastMembers+ " | Cast Members: "+getCastMemberNamesAsString()+" ]";
return String.format(movie,movieName);
}
public boolean equals(Object o)
{
if (o instanceof Movie)
{
Movie other = (Movie) o;
return ((movieName.equalsIgnoreCase(other.movieName)) && (isKidFriendly == other.isKidFriendly)
&&
(numMinutes == other.numMinutes) && (numCastMembers == other.numCastMembers)
&&
(doArraysMatch(castMembers, other.castMembers)));
}
return false;
}
public static void main(String args[])
{
}
}

Step by step
Solved in 2 steps

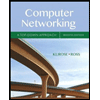
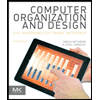
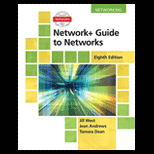
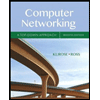
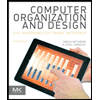
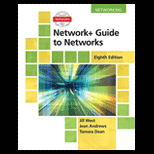
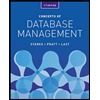
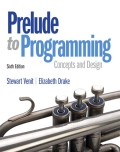
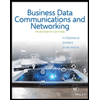