how can i remove the spaces at the end of each line in my code ? code: def load_course(course_name): try: file = open(course_name+'.txt') return file.readlines() except FileNotFoundError as e: return None def word_wrap_text(text,lineWidth): words = list(text.split()) linelen = 0 lines = [] line = '' for word in words: if linelen + len(word) > lineWidth: lines.append(line) line = '' line += word + ' ' linelen = len(line) if line !='': lines.append(line) return '\n'.join(lines) def enhanced_word_wrap_text(text,lineWidth): words = list(text.split()) linelen = 0 lines = [] line = '' for word in words: if linelen + len(word) + 1 > lineWidth: lines.append(line + word[:lineWidth-linelen]) line = word[lineWidth-linelen:] + ' ' else: line += word + ' ' linelen = len(line) if line != '': lines.append(line) return '\n'.join(lines) lineWidth = int(input("")) while True: course = input("")
how can i remove the spaces at the end of each line in my code ?
code:
def load_course(course_name):
try:
file = open(course_name+'.txt')
return file.readlines()
except FileNotFoundError as e:
return None
def word_wrap_text(text,lineWidth):
words = list(text.split())
linelen = 0
lines = []
line = ''
for word in words:
if linelen + len(word) > lineWidth:
lines.append(line)
line = ''
line += word + ' '
linelen = len(line)
if line !='':
lines.append(line)
return '\n'.join(lines)
def enhanced_word_wrap_text(text,lineWidth):
words = list(text.split())
linelen = 0
lines = []
line = ''
for word in words:
if linelen + len(word) + 1 > lineWidth:
lines.append(line + word[:lineWidth-linelen])
line = word[lineWidth-linelen:] + ' '
else:
line += word + ' '
linelen = len(line)
if line != '':
lines.append(line)
return '\n'.join(lines)
lineWidth = int(input(""))
while True:
course = input("")
if course == 'q':
break
lines = load_course(course)
if lines == None:
print('Unable to load course information for course',course)
else:
for line in lines:
print(word_wrap_text(line,lineWidth),'\n')


Trending now
This is a popular solution!
Step by step
Solved in 2 steps

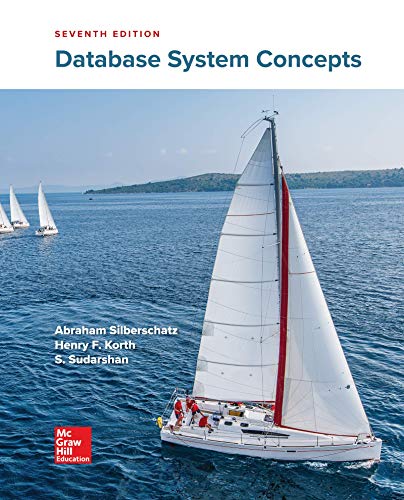
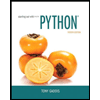
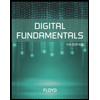
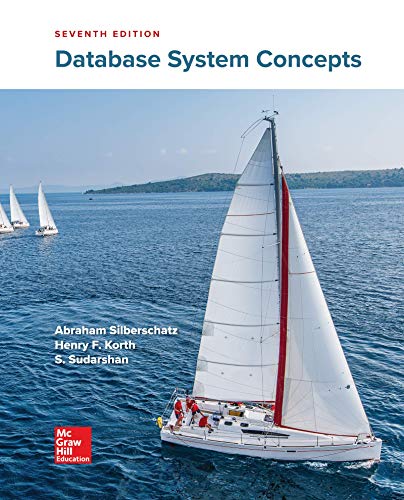
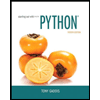
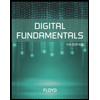
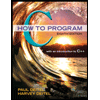
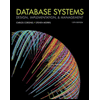
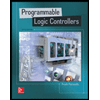