Attached is the python file server.py. There are errors in these codes, so make sure to show screenshots of the fixed code of the python file along with the screenshot of the output as well.
Attached is the python file server.py. There are errors in these codes, so make sure to show screenshots of the fixed code of the python file along with the screenshot of the output as well.


For the offered Python code to function as intended, it looks to have a number of problems and defects that need to be resolved. It appears that a server-side script manages file transfer client connections. To make it work, we'll need to fix these problems.
Please refer to the following steps for the complete solution to the problem above.
Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 2 images

I ran the code and still got the same error. Please fix the error in the python file and show screenshots of the fixed code of the python file along with the screenshot of the output as well.
![import socket
import sys
def handle_client (client_socket):
while True:
command = client_socket.recv(1824).decode() # Fixed the buffer size and added missing colon
if not command:
break
if command == 'quit': # Fixed the comparison operator
break
elif command == 'put': #Fixed the comparison operator
receive_file(client_socket)
elif command == 'get': #Fixed the comparison operator
send_file(client_socket)
else:
if
client_socket.close()
def receive_file(client_socket):
#Implement file receiving Logic from the client.
pass
def send_file(client_socket):
# Implement file sending Logic to the client.
pass
def main():
print("Invalid command.")
break
if len(sys.argv) != 3:
print("Usage: server.py <port> <transport_protocol>") # Added missing angle brackets
return
port, transport_protocol = int(sys.argv[1]), sys.argv[2]
server_socket = socket.socket (socket.AF_INET, socket.SOCK_STREAM)
server socket.bind((¹0.0.0.0, port))
server_socket.listen(5)
print (f"Server listening on port (port}...") # Fixed the string formatting
while True:
name
main()
client_socket, addr = server_socket.accept()
print (F"Accepted connection From [addr[0]}: {addr[1]}") # Fixed the string formatting
handle_client (client_socket)
__main__": # Fixed the if statement
ValueError
30
31
---> 32
Traceback (most recent call last)
-\AppData\Local\Temp/ipykernel_25236/122814978.py in <module>
41
42 if __name__ == "__main__": #Fixed the if statement
---> 43
main()
~\AppData\Local\Temp/ipykernel_25236/122814978.py in main()
print("Usage: server.py <port> <transport_protocol>") # Added missing angle brackets
return
port, transport_protocol = int(sys.argv[1]), sys.argv[2]
33 server_socket = socket.socket (socket.AF_INET, socket.SOCK_STREAM)
34 server_socket.bind(("0.0.0.0", port))
ValueError: invalid literal for int() with base 10: '-'](https://content.bartleby.com/qna-images/question/e7ddc10c-4670-40fd-b02c-6a60c5fcc2f2/6fd5c763-ae55-471b-b66f-9ea692e8208c/e1q2thg_thumbnail.png)
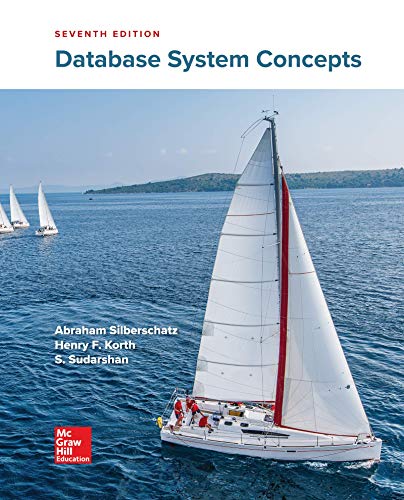
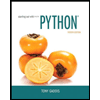
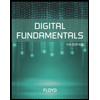
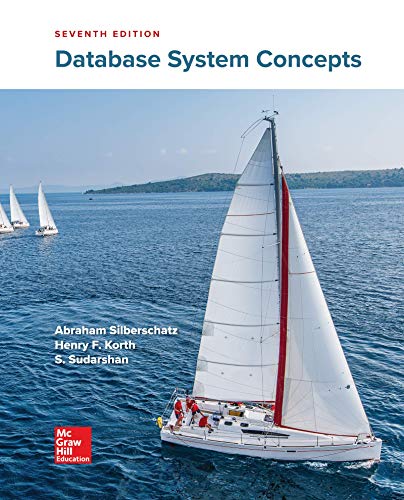
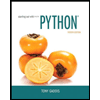
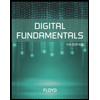
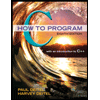
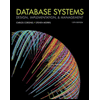
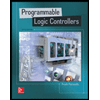