Background Often, data are stored in a very compact but not human-friendly way. Think of how dishes in a menu can be stored in a restaurant database somewhere in the cloud. One way a dish object can be stored is this: { "name": "Margherita", "calories": 800, "price": 18.90, "is_vegetarian": "yes", "spicy_level": 2 } Obviously, this format is not user-readable, so the restaurant's frontend team produced many lines of code to render and display this content in a way that's more understandable to us, the restaurant users. We have a mini-version of the same task coming up. Given a list of similar complex objects (represented by Python dictionaries), display them in a user-friendly way that we'll define in these instructions. What is a "dish"? In this project, one dish item is a dictionary object that is guaranteed to have the following keys: "name": a string that stores the dish's name. "calories": an integer representing the calorie intake for one serving of the dish. "price": a float to represent the cost of the dish. "is_vegetarian": a string (yes/no) to indicate if a dish is vegetarian. "spicy_level": an integer (e.g., from 1 to 4) representing the level of spiciness of the dish. To create a menu that contains all dishes served in a restaurant, we define a list containing dictionaries of dishes information. Here is an example list containing 2 dishes of a restaurant menu that can be used to test our system as we develop it. You can add more of your own examples as long as they adhere to the correct formats and types of dictionary values. restaurant_menu_list = [ { "name": "burrito", "calories": 500, "price": 12.90, "is_vegetarian": "yes", "spicy_level": 2 }, { "name": "rice bowl", "calories": 400, "price": 14.90, "is_vegetarian": "no", "spicy_level": 3 }, { "name": "margherita", "calories": 800, "price": 18.90, "is_vegetarian": "no", "spicy_level": 2 } ] Your TODO Create a variable to hold a list of all dictionaries of dish information data at the top of your main program. We will refer to it as restaurant_menu. Additionally, you need to add a dictionary spicy_scale_map, which will contain the mapping of the spicy level values, as strings, to their textual interpretation (see example below)spicy_scale_map = { 1: "Not spicy", 2: "Low-key spicy", 3: "Hot", 4: "Diabolical", } Define a new function print_restaurant_menu() to display the menu of a restaurant . Add the implementation based on the documentation provided below. note that vegetarian_only is a Boolean option in this function, which then looks at the "is_vegetarian" key of the restaurant_menu dictionary - the function uses it to filter and display the vegetarian-only dishes based on the string "yes". def print_restaurant_menu(restaurant_menu, spicy_scale_map, name_only, show_idx, start_idx, vegetarian_only): """ param: restaurant_menu (list) - a list object that holds the dictionaries for each dish param: spicy_scale_map (dict) - a dictionary object that is expected to have the integer keys that correspond to the "level of spiciness." param: name_only (Boolean) If False, then only the name of the dish is printed. Otherwise, displays the formatted dish information. param: show_idx (Boolean) If False, then the index of the menu is not displayed. Otherwise, displays the "{idx + start_idx}." before the dish name, where idx is the 0-based index in the list. param: start_idx (int) an expected starting value for idx that gets displayed for the first dish, if show_idx is True. param: vegetarian_only (Boolean) If set to False, prints all dishes, regardless of their is_vegetarian status ("yes/no" field status). If set to True , display only the dishes with "is_vegetarian" status set to "yes". returns: None; only prints the restaurant menu """ # Go through the dishes in the restaurant menu: for ...: # if vegetarian_only is True and the dish is not vegetarian, skip if vegetarian_only and ...: ..... # if the index of the task needs to be displayed (show_idx is True), print dish index only if show_idx == True: print(.....) # print the name of the dish # if name_only is False if not name_only: print(.....) print(.....) print(.....) print(.....) Template def print_restaurant_menu(restaurant_menu, spicy_scale_map, name_only, show_idx, start_idx, vegetarian_only): """ Use the template provided above to display the restaurant menu """ pass if __name__ == '__main__': # Add needed variables # Print all dishes print("Full menu:") # restaurant_menu, spicy_scale_map, name_only, show_idx, start_idx, vegetarian_only print_restaurant_menu(restaurant_menu, spicy_scale_map, ..., vegetarian_only=False) # Print only vegetarian dishes print("Vegetarian menu:") print_restaurant_menu(restaurant_menu, spicy_scale_map, ..., vegetarian_only=True)
Background
Often, data are stored in a very compact but not human-friendly way. Think of how dishes in a menu can be stored in a restaurant
{ "name": "Margherita", "calories": 800, "price": 18.90, "is_vegetarian": "yes", "spicy_level": 2 }
Obviously, this format is not user-readable, so the restaurant's frontend team produced many lines of code to render and display this content in a way that's more understandable to us, the restaurant users.
We have a mini-version of the same task coming up. Given a list of similar complex objects (represented by Python dictionaries), display them in a user-friendly way that we'll define in these instructions.
What is a "dish"?
In this project, one dish item is a dictionary object that is guaranteed to have the following keys:
- "name": a string that stores the dish's name.
- "calories": an integer representing the calorie intake for one serving of the dish.
- "price": a float to represent the cost of the dish.
- "is_vegetarian": a string (yes/no) to indicate if a dish is vegetarian.
- "spicy_level": an integer (e.g., from 1 to 4) representing the level of spiciness of the dish.
To create a menu that contains all dishes served in a restaurant, we define a list containing dictionaries of dishes information. Here is an example list containing 2 dishes of a restaurant menu that can be used to test our system as we develop it. You can add more of your own examples as long as they adhere to the correct formats and types of dictionary values.
restaurant_menu_list = [ { "name": "burrito", "calories": 500, "price": 12.90, "is_vegetarian": "yes", "spicy_level": 2 }, { "name": "rice bowl", "calories": 400, "price": 14.90, "is_vegetarian": "no", "spicy_level": 3 }, { "name": "margherita", "calories": 800, "price": 18.90, "is_vegetarian": "no", "spicy_level": 2 } ]
Your TODO
-
Create a variable to hold a list of all dictionaries of dish information data at the top of your main program. We will refer to it as restaurant_menu.
-
Additionally, you need to add a dictionary spicy_scale_map, which will contain the mapping of the spicy level values, as strings, to their textual interpretation (see example below)spicy_scale_map = { 1: "Not spicy", 2: "Low-key spicy", 3: "Hot", 4: "Diabolical", }
Define a new function print_restaurant_menu() to display the menu of a restaurant .
-
-
- Add the implementation based on the documentation provided below.
- note that vegetarian_only is a Boolean option in this function, which then looks at the "is_vegetarian" key of the restaurant_menu dictionary - the function uses it to filter and display the vegetarian-only dishes based on the string "yes".
- def print_restaurant_menu(restaurant_menu, spicy_scale_map, name_only, show_idx, start_idx, vegetarian_only): """ param: restaurant_menu (list) - a list object that holds the dictionaries for each dish param: spicy_scale_map (dict) - a dictionary object that is expected to have the integer keys that correspond to the "level of spiciness." param: name_only (Boolean) If False, then only the name of the dish is printed. Otherwise, displays the formatted dish information. param: show_idx (Boolean) If False, then the index of the menu is not displayed. Otherwise, displays the "{idx + start_idx}." before the dish name, where idx is the 0-based index in the list. param: start_idx (int) an expected starting value for idx that gets displayed for the first dish, if show_idx is True. param: vegetarian_only (Boolean) If set to False, prints all dishes, regardless of their is_vegetarian status ("yes/no" field status). If set to True , display only the dishes with "is_vegetarian" status set to "yes". returns: None; only prints the restaurant menu """ # Go through the dishes in the restaurant menu: for ...: # if vegetarian_only is True and the dish is not vegetarian, skip if vegetarian_only and ...: ..... # if the index of the task needs to be displayed (show_idx is True), print dish index only if show_idx == True: print(.....) # print the name of the dish # if name_only is False if not name_only: print(.....) print(.....) print(.....) print(.....)
-
Template
def print_restaurant_menu(restaurant_menu, spicy_scale_map, name_only, show_idx, start_idx, vegetarian_only):
"""
Use the template provided above to display the restaurant menu
"""
pass
if __name__ == '__main__':
# Add needed variables
# Print all dishes
print("Full menu:")
# restaurant_menu, spicy_scale_map, name_only, show_idx, start_idx, vegetarian_only
print_restaurant_menu(restaurant_menu, spicy_scale_map, ..., vegetarian_only=False)
# Print only vegetarian dishes
print("Vegetarian menu:")
print_restaurant_menu(restaurant_menu, spicy_scale_map, ..., vegetarian_only=True)

Trending now
This is a popular solution!
Step by step
Solved in 5 steps with 3 images

Hi sorry can you write it as
Full menu:
1. BURRITO
* Calories: 500
* Price: $12.90
* Vegetarian? yes
* Spicy level: Low-key spicy
2. RICE BOWL
* Calories: 400
* Price: $14.90
* Vegetarian? no
* Spicy level: Hot
3. MARGHERITA
* Calories: 800
*Price: $18.90
* Vegetarian? no
* Spicy level: Low-key spicy
Vegetarian menu:
1. BURRITO
* Calories: 500
* Price: $12.90
* Vegetarian? yes
* Spicy level: Low-key spicy
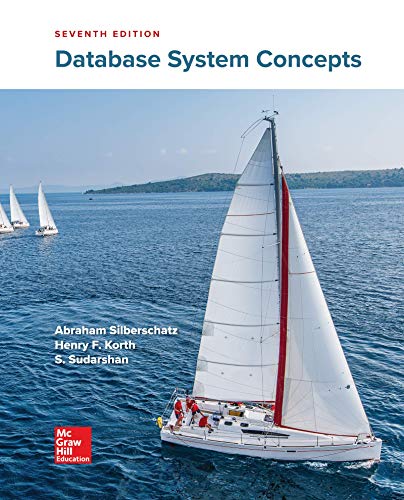
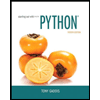
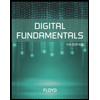
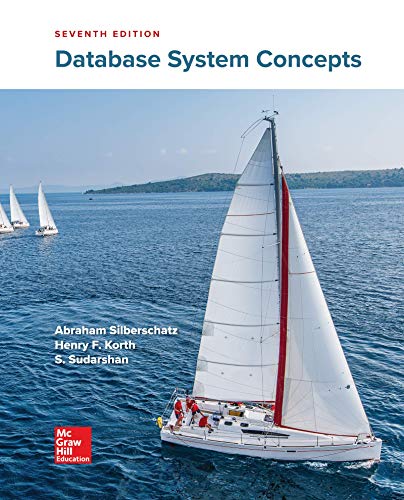
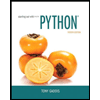
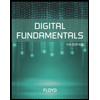
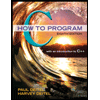
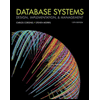
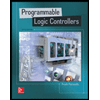