Hi! I need help with the following JAVASCRIPT programming challenge. I need this to be done using simple and beginner level techniques and in pure javascript as in nothing meant for webpages or similar. I have provided some code that i started but I cant get it to work properly. Please use my code as reference to methods required and please help me understand where i went wrong. Thank you!! Introduction: In this assignment we will demonstrate an understanding of functions and modules. 1. I struggle with doing metric conversions, so I'm going to have you write a conversion utility module to help me. Start by creating a file called conversionutils.js. In this file, code the following functions: a. centi_to_milli: Takes a value for centimeters and returns the value in millimeters. b. meter_to_centi: Takes a value for meters and returns the value in centimeters. c. kilo_to_meter: Takes a value for kilometers and returns the value in meters. d. inch_to_centi: Takes a value for inches and returns the value in centimeters. e. feet_to_centi: Takes a value for feet and returns the value in centimeters. f. yard_to_meter: Takes a value for yards and returns the value in meters. g. mile_to_meter: Takes a value for miles and returns the value in meters. h. mile_to_kilo: Takes a value for miles and returns the value in kilometers. Make sure to export your functions to make this a module. 2. Create a file called conversion.js. Start by importing your conversionutils module. When I run the conversion program, it should display a menu with all the different conversion choices. When I make a selection, it should prompt me for a value and display the conversion result by invoking the function in the module. The program should continuously display the menu until I select the exit option. The program should display an error message if I select an invalid option and prompt me until I select a valid menu option. Save your program in a folder called assignment_4. Zip that folder and submit the zip file in the dropbox. My code: // Functions to convert each unit to another unit// function centi_to_milli(centi) { return centi * 10; } module.exports = centi_to_milli; function meter_to_centi(meter) { return meter * 100; } module.exports = meter_to_centi; function kilo_to_meter(kilo) { return kilo * 1000; } module.exports = kilo_to_meter; function inch_to_centi(inch) { return inch * 2.54; } module.exports = inch_to_centi; function feet_to_centi(feet) { return feet * 30.48; } module.exports = feet_to_centi; function yard_to_meter(yard) { return yard * 91.44; } module.exports = yard_to_meter; function mile_to_meter(mile) { return mile * 1609.344; } module.exports = mile_to_meter; function mile_to_kilo(mile2) { return mile2 * 1.61; } module.exports = mile_to_kilo; second file: // Import the two modules const centi_to_milli = require('./centi_to_milli'); const meter_to_centi = require('./meter_to_centi'); //Then, you can display the menu with the user input options: // Display the menu console.log('Please select an option from the menu:'); console.log('1: Convert centimeters to millimeters'); console.log('2: Convert meters to centimeters'); // Get user input const option = prompt('Please select an option:'); // Choose the right function depending on the user input if (option === '1') { const cm = prompt('Please enter the amount of centimeters:'); const mm = cmToMm(cm); console.log(`${cm} cm is equal to ${mm} mm`); } else if (option === '2') { const m = prompt('Please enter the amount of meters:'); const cm = mToCm(m); console.log(`${m} m is equal to ${cm} cm`); } else { console.log('Invalid option.');
Hi! I need help with the following JAVASCRIPT programming challenge.
I need this to be done using simple and beginner level techniques and in pure javascript as in nothing meant for webpages or similar. I have provided some code that i started but I cant get it to work properly. Please use my code as reference to methods required and please help me understand where i went wrong. Thank you!!
Introduction: In this assignment we will demonstrate an understanding of functions and modules.
1. I struggle with doing metric conversions, so I'm going to have you write a conversion utility module to help me.
Start by creating a file called conversionutils.js. In this file, code the following functions:
a. centi_to_milli: Takes a value for centimeters and returns the value in millimeters.
b. meter_to_centi: Takes a value for meters and returns the value in centimeters.
c. kilo_to_meter: Takes a value for kilometers and returns the value in meters.
d. inch_to_centi: Takes a value for inches and returns the value in centimeters.
e. feet_to_centi: Takes a value for feet and returns the value in centimeters.
f. yard_to_meter: Takes a value for yards and returns the value in meters.
g. mile_to_meter: Takes a value for miles and returns the value in meters.
h. mile_to_kilo: Takes a value for miles and returns the value in kilometers.
Make sure to export your functions to make this a module.
2. Create a file called conversion.js. Start by importing your conversionutils module. When I run the conversion program, it should display a menu with all the different conversion choices. When I make a selection, it should prompt me for a value and display the conversion result by invoking the function in the module. The program should continuously display the menu until I select the exit option. The program should display an error message if I select an invalid option and prompt me until I select a valid menu option.
Save your program in a folder called assignment_4. Zip that folder and submit the zip file in the dropbox.
My code:
// Functions to convert each unit to another unit//
function centi_to_milli(centi)
{
return centi * 10;
}
module.exports = centi_to_milli;
function meter_to_centi(meter)
{
return meter * 100;
}
module.exports = meter_to_centi;
function kilo_to_meter(kilo)
{
return kilo * 1000;
}
module.exports = kilo_to_meter;
function inch_to_centi(inch)
{
return inch * 2.54;
}
module.exports = inch_to_centi;
function feet_to_centi(feet)
{
return feet * 30.48;
}
module.exports = feet_to_centi;
function yard_to_meter(yard)
{
return yard * 91.44;
}
module.exports = yard_to_meter;
function mile_to_meter(mile)
{
return mile * 1609.344;
}
module.exports = mile_to_meter;
function mile_to_kilo(mile2)
{
return mile2 * 1.61;
}
module.exports = mile_to_kilo;
second file:
// Import the two modules
const centi_to_milli = require('./centi_to_milli');
const meter_to_centi = require('./meter_to_centi');
//Then, you can display the menu with the user input options:
// Display the menu
console.log('Please select an option from the menu:');
console.log('1: Convert centimeters to millimeters');
console.log('2: Convert meters to centimeters');
// Get user input
const option = prompt('Please select an option:');
// Choose the right function depending on the user input
if (option === '1')
{
const cm = prompt('Please enter the amount of centimeters:');
const mm = cmToMm(cm);
console.log(`${cm} cm is equal to ${mm} mm`);
}
else if (option === '2')
{
const m = prompt('Please enter the amount of meters:');
const cm = mToCm(m);
console.log(`${m} m is equal to ${cm} cm`);
}
else
{
console.log('Invalid option.');
}


Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

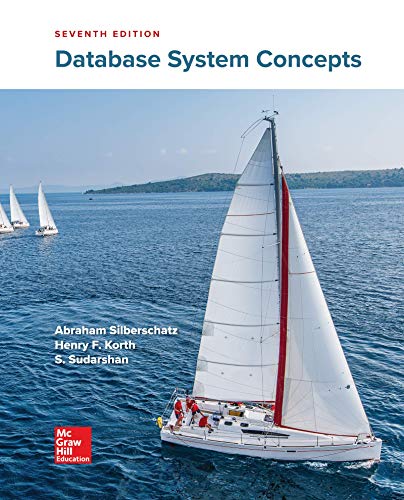
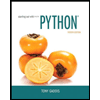
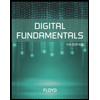
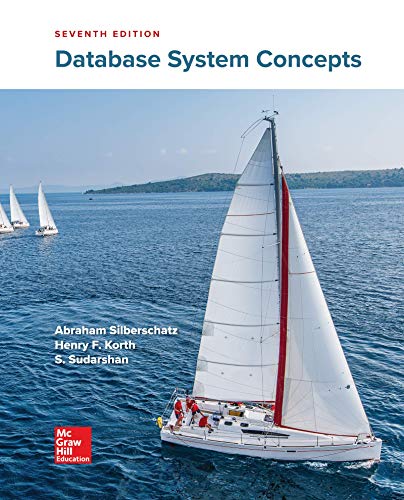
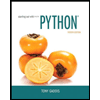
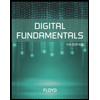
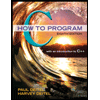
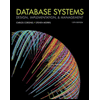
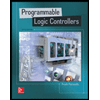