Here is the Python code for our Truth Table Generator function truthTable() which takes two parameters: A Boolean expression: e.g. A AND NOT (B OR C) The number of inputs: either 2, 3 or 4: A, B, C and D Main.py def truthTable(expression,inputs=2): print("Boolean Expression:") print(" X = " + expression.upper()) expression = expression.lower() #replace Boolean Operators with bitwise operators expression = expression.replace("and","&") expression = expression.replace("xor","^") expression = expression.replace("or","|") expression = expression.replace("not","~") print("\nTruth Table:") if inputs==2: print(" -------------") print(" | A | B | X |") print(" -------------") for a in range(0,2): for b in range(0,2): x = eval(expression) print(" | " + str(a) + " | " + str(b) + " | " + str(x) + " |" ) print(" -------------") elif inputs==3: print(" -----------------") print(" | A | B | C | X |") print(" -----------------") for a in range(0,2): for b in range(0,2): for c in range(0,2): x = eval(expression) print(" | " + str(a) + " | " + str(b) + " | " + str(c) + " | " + str(x) + " |" ) print(" -----------------") elif inputs==4: print(" ---------------------") print(" | A | B | C | D | X |") print(" ---------------------") for a in range(0,2): for b in range(0,2): for c in range(0,2): for d in range(0,2): x = eval(expression) print(" | " + str(a) + " | " + str(b) + " | " + str(c) + " | " + str(d) + " | " + str(x) + " |" ) print(" ---------------------") ############################################## expression = "A AND NOT (B XOR C)" truthTable(expression,3) TASK: Write an additional function to perform a bitwise left shift or a bitwise right shift using the bitwise operators << and >>. Check that performing a left shift by n place(s) is equivalent to multiplying a number by 2n. For instance 5 << 3 is the same as 5×23 = 40. Check that performing a right shift by n place(s) is equivalent to dividing a number by 2n (whole division). For instance 40 >> 3 is the same as 40/23 = 5.
Here is the Python code for our Truth Table Generator function truthTable() which takes two parameters:
- A Boolean expression: e.g. A AND NOT (B OR C)
- The number of inputs: either 2, 3 or 4: A, B, C and D
Main.py
def truthTable(expression,inputs=2):
print("Boolean Expression:")
print(" X = " + expression.upper())
expression = expression.lower()
#replace Boolean Operators with bitwise operators
expression = expression.replace("and","&")
expression = expression.replace("xor","^")
expression = expression.replace("or","|")
expression = expression.replace("not","~")
print("\nTruth Table:")
if inputs==2:
print(" -------------")
print(" | A | B | X |")
print(" -------------")
for a in range(0,2):
for b in range(0,2):
x = eval(expression)
print(" | " + str(a) + " | " + str(b) + " | " + str(x) + " |" )
print(" -------------")
elif inputs==3:
print(" -----------------")
print(" | A | B | C | X |")
print(" -----------------")
for a in range(0,2):
for b in range(0,2):
for c in range(0,2):
x = eval(expression)
print(" | " + str(a) + " | " + str(b) + " | " + str(c) + " | " + str(x) + " |" )
print(" -----------------")
elif inputs==4:
print(" ---------------------")
print(" | A | B | C | D | X |")
print(" ---------------------")
for a in range(0,2):
for b in range(0,2):
for c in range(0,2):
for d in range(0,2):
x = eval(expression)
print(" | " + str(a) + " | " + str(b) + " | " + str(c) + " | " + str(d) + " | " + str(x) + " |" )
print(" ---------------------")##############################################
expression = "A AND NOT (B XOR C)"
truthTable(expression,3)
TASK:Write an additional function to perform a bitwise left shift or a bitwise right shift using the bitwise operators << and >>.
Check that performing a left shift by n place(s) is equivalent to multiplying a number by 2n.
For instance 5 << 3 is the same as 5×23 = 40.
Check that performing a right shift by n place(s) is equivalent to dividing a number by 2n (whole division).
For instance 40 >> 3 is the same as 40/23 = 5.

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

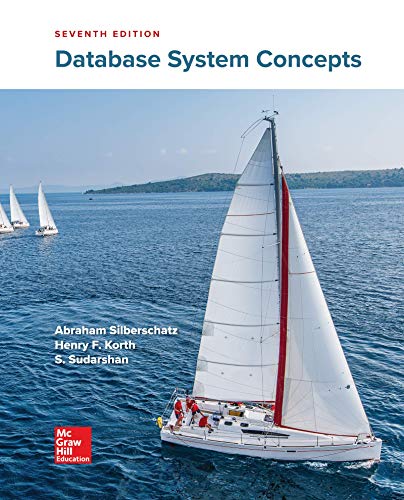
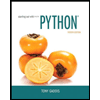
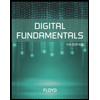
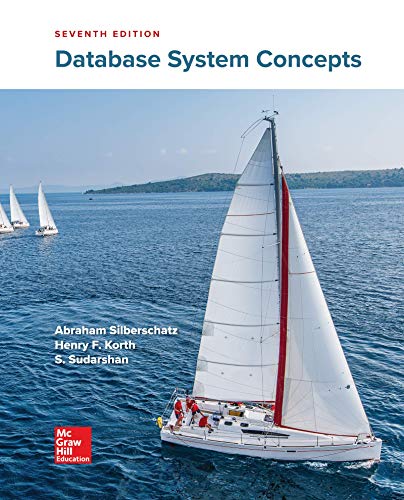
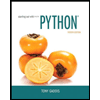
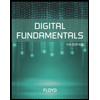
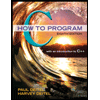
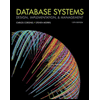
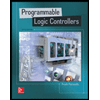