here is the file/starter code: public class LinkedListPracticeLab { private LinkedListPracticeLab(){} public static void main(String[] args) { // Test all methods here } /** * Given the heads of two sorted linked lists, merges the two lists in a one sorted list. * The list is made by splicing together the nodes of the original two lists, without * creating any new nodes. * * Returns the head of the merged linked list. */ public static ListNode mergeLists(ListNode head1, ListNode head2) { return null; } /** * Given the head of a sorted linked list, deletes all duplicates such that each element appears only once. * * Returns the head of the resulting linked list, which is still sorted. */ public static ListNode deleteDuplicates(ListNode head) { return null; } /** * Given the head of a linked list and an integer val, removes all the nodes of the linked list that has * Node.val == val. * * Returns the head of the resulting list. */ public static ListNode removeElements(ListNode head, int val) { return null; } /** * Given the head of a zero-indexed linked list and two indices i and j, swaps the elements at these indices. * * Returns the head of the resulting list. */ public static ListNode swapElements(ListNode head, int i, int j) { return null; } /** * Given the head of a singly linked list, reverse the list, and return the reversed list. */ public static ListNode reverseList(ListNode head) { return null; } /** * Given the head of a singly linked list, returns the middle node of the linked list. * * If there are an even number of elements -- and thus two middle nodes -- returns the second middle node. */ public static ListNode middleNode(ListNode head) { return null; } }
here is the file/starter code:
public class LinkedListPracticeLab {
private LinkedListPracticeLab(){}
public static void main(String[] args) {
// Test all methods here
}
/**
* Given the heads of two sorted linked lists, merges the two lists in a one
sorted list.
* The list is made by splicing together the nodes of the original two lists,
without
* creating any new nodes.
*
* Returns the head of the merged linked list.
*/
public static ListNode mergeLists(ListNode head1, ListNode head2) {
return null;
}
/**
* Given the head of a sorted linked list, deletes all duplicates such that
each element appears only once.
*
* Returns the head of the resulting linked list, which is still sorted.
*/
public static ListNode deleteDuplicates(ListNode head) {
return null;
}
/**
* Given the head of a linked list and an integer val, removes all the nodes of
the linked list that has
* Node.val == val.
*
* Returns the head of the resulting list.
*/
public static ListNode removeElements(ListNode head, int val) {
return null;
}
/**
* Given the head of a zero-indexed linked list and two indices i and j, swaps
the elements at these indices.
*
* Returns the head of the resulting list.
*/
public static ListNode swapElements(ListNode head, int i, int j) {
return null;
}
/**
* Given the head of a singly linked list, reverse the list, and return the
reversed list.
*/
public static ListNode reverseList(ListNode head) {
return null;
}
/**
* Given the head of a singly linked list, returns the middle node of the
linked list.
*
* If there are an even number of elements -- and thus two middle nodes --
returns the second middle node.
*/
public static ListNode middleNode(ListNode head) {
return null;
}
}


Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

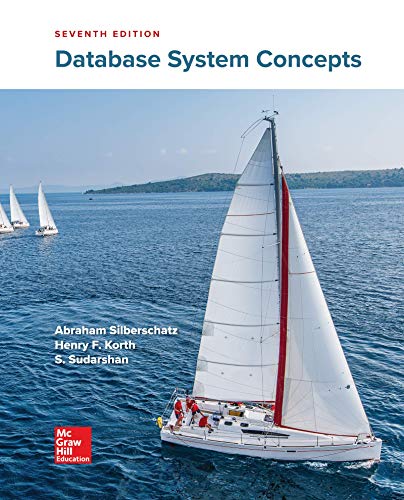
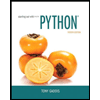
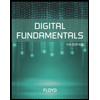
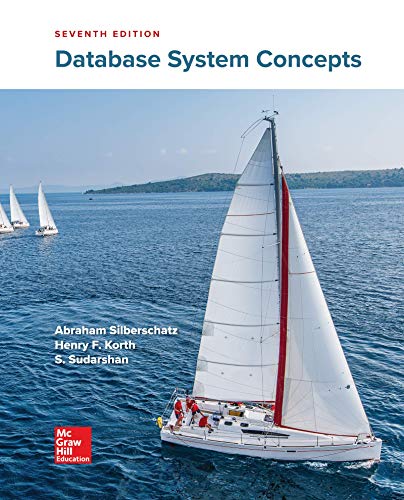
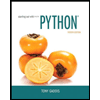
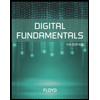
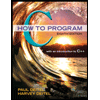
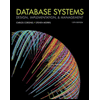
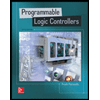