Help me please in C++: Design a class named largeIntegers such that an object of this class can store an integer of any number of digits. Add operations to add, subtract, multiply, and compare integers stored in two objects. Also add constructors to properly initialize objects and functions to set, retrieve, and print the values of objects. Write a program to test your class. There are 3 tabs: main.cpp, largeIntegers.cpp, largeIntegers.h. Also the code should implement the following test: int numList1[] = {2,2,2,2,2,2,2,2,2,2,2,2,2,2,2,2,2,2,2,2,2}; // 21 characters int numList2[] = {1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1}; // 21 characters int numResults[] = {3,3,3,3,3,3,3,3,3,3,3,3,3,3,3,3,3,3,3,3,3}; largeIntegers num1(numList1, 21, '+'); largeIntegers num2(numList2, 21, '+'); largeIntegers result(numResults, 21, '+'); TEST(Add, 0) { ASSERT_TRUE(num1.add(num2).equal(result)); }
Help me please in C++:
Design a class named largeIntegers such that an object of this class can store an integer of any number of digits. Add operations to add, subtract, multiply, and compare integers stored in two objects. Also add constructors to properly initialize objects and functions to set, retrieve, and print the values of objects. Write a program to test your class. There are 3 tabs: main.cpp, largeIntegers.cpp, largeIntegers.h. Also the code should implement the following test:
int numList1[] = {2,2,2,2,2,2,2,2,2,2,2,2,2,2,2,2,2,2,2,2,2}; // 21 characters int numList2[] = {1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1}; // 21 characters
int numResults[] = {3,3,3,3,3,3,3,3,3,3,3,3,3,3,3,3,3,3,3,3,3};
largeIntegers num1(numList1, 21, '+');
largeIntegers num2(numList2, 21, '+');
largeIntegers result(numResults, 21, '+');
TEST(Add, 0)
{ ASSERT_TRUE(num1.add(num2).equal(result)); }

Step by step
Solved in 7 steps with 1 images

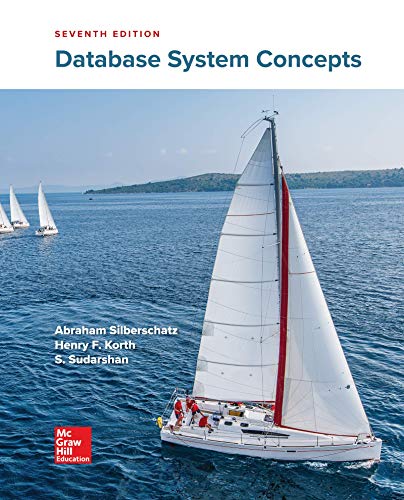
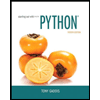
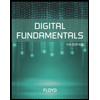
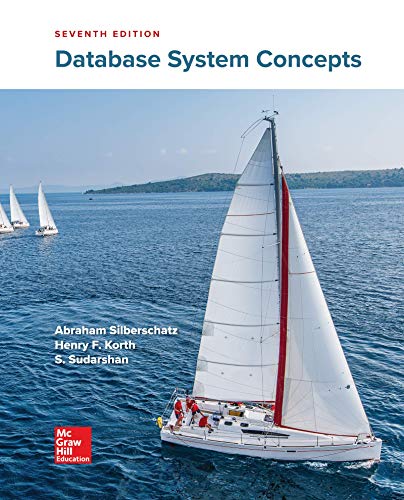
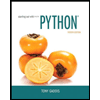
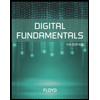
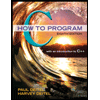
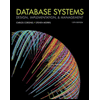
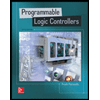