Hello, the debugging question is in the screenshot and I included the HTML and JS below, thank you! HTML Hands-on Project 4-5 Hands-on Project 4-5 Angular Degrees (°) to Circular Radians (rad) Converter Angle in Degrees ↔ Angle in Radians Enter an angular degree or a circular radian in either input box and press Tab to convert. JAVASCRIPT "use strict"; // Function to convert degrees to radians function degreesToRadians(degrees) { return degrees*PI/80; } // Function to convert radians to degrees function radiansToDegrees(radians) { return radians*180/PI; } // Event handler that converts radians to degrees when the input box is changed document.getElementById("rValue").onchange = function( { let radians = document.getElementById("rValue").value; document.getElementById("aValue").value = formatValue3(radiansToDegrees(radian)); } // Event handler that converts degrees to radians when the input box is changed document.getElementById("aValue").onchange = function() { let degrees = document.getElementById("aValue").value; document.getElementById("rValue").value = formatValue3(degreesToRadians(degrees); } /* ================================================================= */ // Function to display a numeric value in the format ##.### function formatValue3(value) { return value.toFixed(3); }
Hello, the debugging question is in the screenshot and I included the HTML and JS below, thank you!
HTML
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8" />
<meta name="viewport" content="width=device-width,initial-scale=1.0">
<title>Hands-on Project 4-5</title>
<link rel="stylesheet" href="styles.css" />
<script src="project04-05_txt.js" defer></script>
</head>
<body>
<header>
<h1>Hands-on Project 4-5</h1>
</header>
<article>
<h2>Angular Degrees (°) to Circular Radians (rad) Converter</h2>
<form>
<div>
<h3>Angle in Degrees</h3>
<input type="number" id="aValue" value="90" />
</div>
<div id="arrow">↔</div>
<div>
<h3>Angle in Radians</h3>
<input type="number" id="rValue" value="1.5708" />
</div>
</form>
<footer>
Enter an angular degree or a circular radian in either input box and press Tab to convert.
</footer>
</article>
</body>
</html>
JAVASCRIPT
"use strict";
// Function to convert degrees to radians
function degreesToRadians(degrees) {
return degrees*PI/80;
}
// Function to convert radians to degrees
function radiansToDegrees(radians) {
return radians*180/PI;
}
// Event handler that converts radians to degrees when the input box is changed
document.getElementById("rValue").onchange = function( {
let radians = document.getElementById("rValue").value;
document.getElementById("aValue").value = formatValue3(radiansToDegrees(radian));
}
// Event handler that converts degrees to radians when the input box is changed
document.getElementById("aValue").onchange = function() {
let degrees = document.getElementById("aValue").value;
document.getElementById("rValue").value = formatValue3(degreesToRadians(degrees);
}
/* ================================================================= */
// Function to display a numeric value in the format ##.###
function formatValue3(value) {
return value.toFixed(3);
}


The following are the errors that needs to be fixed in the javascript file.
1. Missing closing bracket in the line
document.getElementById("rValue").onchange = function() {
2. Missing closing braacket in the line
document.getElementById("rValue").value = formatValue3(degreesToRadians(degrees));
3. changing of variable name to radians in the line
4. PI value is not defined> we can use Math.PI
return degrees*(Math.PI/180);
5. The logical error is 80 is there instead of 180.
return degrees*(Math.PI/180);
After doing the changes the javascript code is
"use strict";
// Function to convert degrees to radians
function degreesToRadians(degrees) {
return degrees*Math.PI/180;
}
// Function to convert radians to degrees
function radiansToDegrees(radians) {
return radians*180/Math.PI;
}
// Event handler that converts radians to degrees when the input box is changed
document.getElementById("rValue").onchange = function() {
let radians = document.getElementById("rValue").value;
console.log("Radians ="+radians);
document.getElementById("aValue").value = formatValue3(radiansToDegrees(radians));
}
// Event handler that converts degrees to radians when the input box is changed
document.getElementById("aValue").onchange = function() {
let degrees = document.getElementById("aValue").value;
console.log("Degrees = "+degrees);
document.getElementById("rValue").value = formatValue3(degreesToRadians(degrees));
}
/* ================================================================= */
// Function to display a numeric value in the format ##.###
function formatValue3(value) {
return value.toFixed(3);
}
Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

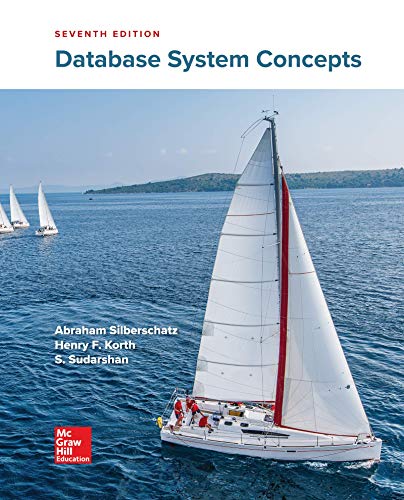
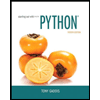
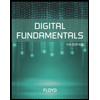
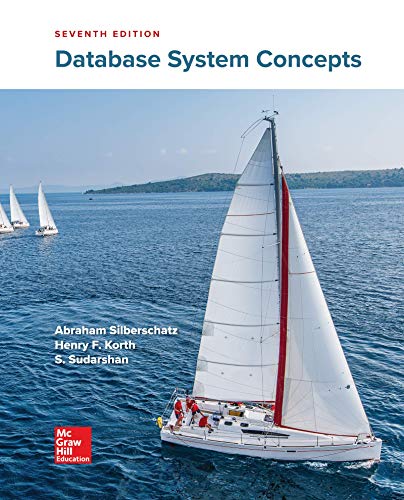
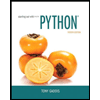
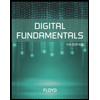
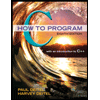
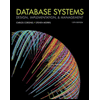
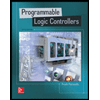