hello! can you help debug my program? it's in Java! here are the errors I'm getting: line 189: total_weight cannot be resolved or is not a fieldline 93: the type Snake must implement the inherited abstract method Animal.makeNoise()line 139: the method actual_num_animals(int) is undefined for the type Zooline 152: the left-hand side of an assignment must be a variableline 169: syntax error, insert } to complete Blockline 152: syntax error, insert “. class” to complete expressionline 139: syntax error on token “<“, ( expectedline 139: syntax error on token “{“ invalid AssignmentOperatorline 152: i cannot be resolved to a variableline 152: i cannot be resolved to a variableline 154: i cannot be resolved to a variable here is my code: package zoo; public abstract class Animal { private String name; private double weight; private int age; public Animal() { name=""; weight=0; age=0; } public Animal(String name, double weight, int age) { this.name = name; this.weight = weight; this.age = age; } public abstract String makeNoise(); public double getWeight() { return weight; } public String toString() { return name+ ",weight" + weight+ ", age "+ age; } } public class Cow extends Animal { private int num_spots; public Cow() { super(); num_spots = 0; } public Cow(String name, double weight, int age, int num_spots) { super(name, weight, age); this.num_spots = num_spots; } @Override public String makeNoise() { return "Moooooo"; } public String toString() { return "Cow" + super.toString()+ ". Number of spots: "+num_spots; } } //horse public class Horse extends Animal { private double top_speed; public Horse() { super(); top_speed = 0.0; } public Horse(String name, double weight, int age, double top_speed) { super(name, weight, age); this.top_speed = top_speed; } @Override public String makeNoise() { return "Whinny"; } public String toString() { return "Horse "+super.toString()+". Top speed: "+top_speed; } } //Snake public class Snake extends Animal { private int num_fangs; public Snake() { super(); num_fangs = 0; } public Snake(String name, double weight, int age, int num_fangs) { super(name, weight, age); this.num_fangs=num_fangs; } public String toString() { return "Snake "+ super.toString() + ". Number of fangs: " + num_fangs; } } //here is the zoo public class Zoo { private int actual_num_animals; private int num_cages; private Animal[] animals; public Zoo() { actual_num_animals = 0; num_cages = 20; animals = new Animal[num_cages]; } public Zoo(int num_cages) { actual_num_animals =0; this.num_cages=num_cages; animals=new Animal[num_cages]; } public void add(Animal a) { if(actual_num_animals < num_cages) { animals[actual_num_animals< num_cages) ]{ animals[actual_num_animals]=a; actual_num_animals++; } else { System.out.println("Cannot add more animals to the zoo."); } } public double total_weight() { double totalWeight = 0.0; for (int = 0; i<actual_num_animals; i++) { totalWeight += animals[i].getWeight(); } return totalWeight; } public void make_all_noises() { for (int i=0; i < actual_num_animals; i++) { System.out.println(animals[i].makeNoise()); } } public void print_all_animals() { for (int i = 0; i < actual_num_animals; i++) { System.out.println(animals[i]); } public static void main(String[]args) { Zoo z = new Zoo(); Snake sly = new Snake("Sly", 5.0, 2, 2); Snake sly2 = new Snake("Slyme", 10.0, 1, 2); Cow blossy = new Cow("Blossy", 900., 5, 10); Horse prince = new Horse("Prince", 1000., 5, 23.2); //Following not allowed because Animal is abstract //Animal spot = new Animal("Spot, 10., 4); z.add(sly); z.add(sly2); z.add(blossy); z.add(prince);; z.make_all_noises(); System.out.println("Total weight ="+ z.total_weight); System.out.println("**************"); System.out.println("Animal Printout:"); z.print_all_animals(); } }
hello! can you help debug my program? it's in Java!
here are the errors I'm getting:
line 189: total_weight cannot be resolved or is not a field
line 93: the type Snake must implement the inherited abstract method Animal.makeNoise()
line 139: the method actual_num_animals(int) is undefined for the type Zoo
line 152: the left-hand side of an assignment must be a variable
line 169: syntax error, insert } to complete Block
line 152: syntax error, insert “. class” to complete expression
line 139: syntax error on token “<“, ( expected
line 139: syntax error on token “{“ invalid AssignmentOperator
line 152: i cannot be resolved to a variable
line 152: i cannot be resolved to a variable
line 154: i cannot be resolved to a variable
here is my code:
package zoo;
public abstract class Animal {
private String name;
private double weight;
private int age;
public Animal() {
name="";
weight=0;
age=0;
}
public Animal(String name, double weight, int age)
{
this.name = name;
this.weight = weight;
this.age = age;
}
public abstract String makeNoise();
public double getWeight()
{
return weight;
}
public String toString()
{
return name+ ",weight" + weight+ ", age "+ age;
}
}
public class Cow extends Animal
{
private int num_spots;
public Cow()
{
super();
num_spots = 0;
}
public Cow(String name, double weight, int age, int num_spots)
{
super(name, weight, age);
this.num_spots = num_spots;
}
@Override
public String makeNoise()
{
return "Moooooo";
}
public String toString()
{
return "Cow" + super.toString()+ ". Number of spots: "+num_spots;
}
}
//horse
public class Horse extends Animal
{
private double top_speed;
public Horse()
{
super();
top_speed = 0.0;
}
public Horse(String name, double weight, int age, double top_speed)
{
super(name, weight, age);
this.top_speed = top_speed;
}
@Override
public String makeNoise()
{
return "Whinny";
}
public String toString()
{
return "Horse "+super.toString()+". Top speed: "+top_speed;
}
}
//Snake
public class Snake extends Animal
{
private int num_fangs;
public Snake()
{
super();
num_fangs = 0;
}
public Snake(String name, double weight, int age, int num_fangs)
{
super(name, weight, age);
this.num_fangs=num_fangs;
}
public String toString()
{
return "Snake "+ super.toString() + ". Number of fangs: " + num_fangs;
}
}
//here is the zoo
public class Zoo {
private int actual_num_animals;
private int num_cages;
private Animal[] animals;
public Zoo()
{
actual_num_animals = 0;
num_cages = 20;
animals = new Animal[num_cages];
}
public Zoo(int num_cages)
{
actual_num_animals =0;
this.num_cages=num_cages;
animals=new Animal[num_cages];
}
public void add(Animal a)
{
if(actual_num_animals < num_cages)
{
animals[actual_num_animals< num_cages) ]{
animals[actual_num_animals]=a;
actual_num_animals++;
}
else
{
System.out.println("Cannot add more animals to the zoo.");
}
}
public double total_weight()
{
double totalWeight = 0.0;
for (int = 0; i<actual_num_animals; i++)
{
totalWeight += animals[i].getWeight();
}
return totalWeight;
}
public void make_all_noises()
{
for (int i=0; i < actual_num_animals; i++)
{
System.out.println(animals[i].makeNoise());
}
}
public void print_all_animals()
{
for (int i = 0; i < actual_num_animals; i++)
{
System.out.println(animals[i]);
}
public static void main(String[]args)
{
Zoo z = new Zoo();
Snake sly = new Snake("Sly", 5.0, 2, 2);
Snake sly2 = new Snake("Slyme", 10.0, 1, 2);
Cow blossy = new Cow("Blossy", 900., 5, 10);
Horse prince = new Horse("Prince", 1000., 5, 23.2);
//Following not allowed because Animal is abstract
//Animal spot = new Animal("Spot, 10., 4);
z.add(sly);
z.add(sly2);
z.add(blossy);
z.add(prince);;
z.make_all_noises();
System.out.println("Total weight ="+ z.total_weight);
System.out.println("**************");
System.out.println("Animal Printout:");
z.print_all_animals();
}
}

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

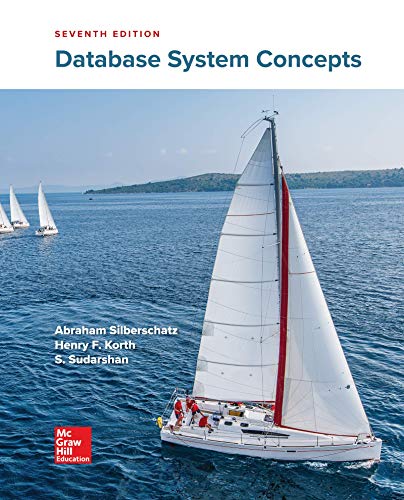
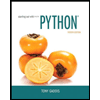
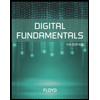
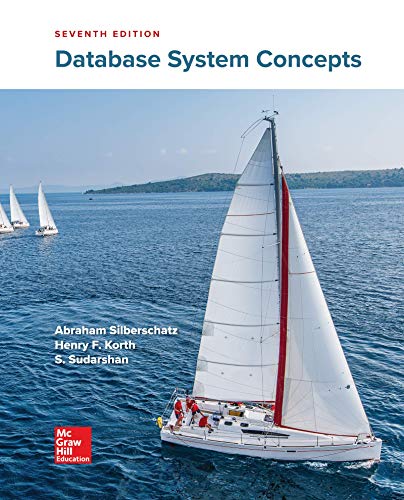
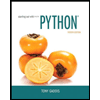
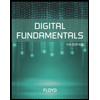
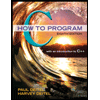
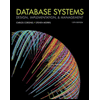
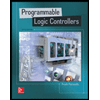