Hello, Can someone please help me how to write these functions in Java, But only using the java bit operators & (and), | (or),^ (xor), >> (right shift), >>> (right shift logical), and << (left shift)? Thank you!
Hello, Can someone please help me how to write these functions in Java, But only using the java bit operators & (and), | (or),^ (xor), >> (right shift), >>> (right shift logical), and << (left shift)? Thank you!
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
100%
Hello, Can someone please help me how to write these functions in Java, But only using the java bit operators & (and), | (or),^ (xor), >> (right shift), >>> (right shift logical), and << (left shift)? Thank you!

Transcribed Image Text:### Java Utility Functions for Integer Manipulation
Below are various Java static functions used for manipulating integers. Each function serves a specific purpose, from checking whether a number is odd to manipulating bits.
#### 1. Checking If a Number Is Odd
**Method:**
```java
public static int isOdd(int x)
```
**Description:**
This function returns `1` if the number is odd and `0` if it is even.
- **Example Outputs:**
- `isOdd(6)` returns `0`.
- `isOdd(5)` returns `1`.
#### 2. Division by 4
**Method:**
```java
public static int DivBy4(int x)
```
**Description:**
This function performs integer division by 4.
- **Example Outputs:**
- `DivBy4(6)` returns `1`.
- `DivBy4(13)` returns `3`.
- `DivBy4(3)` returns `0`.
- `DivBy4(-16)` returns `-4`.
#### 3. Nearest Odd Number
**Method:**
```java
public static int nearestOdd(int x)
```
**Description:**
This function rounds up the number to the nearest odd number.
- **Example Outputs:**
- `nearestOdd(6)` returns `7`.
- `nearestOdd(5)` returns `5`.
- `nearestOdd(-4)` returns `-3`.
#### 4. Flipping Parity
**Method:**
```java
public static int flipParity(int x)
```
**Description:**
This function adds `1` to even numbers, and subtracts `1` from odd numbers.
- **Example Outputs:**
- `flipParity(6)` returns `7`.
- `flipParity(5)` returns `4`.
- `flipParity(-3)` returns `-4`.
#### 5. Checking If a Number Is Negative
**Method:**
```java
public static int isNegative(int x)
```
**Description:**
This function returns `0` if `x` is positive, and `1` if `x` is negative.
- **Example Outputs:**
- `isNegative(4)` returns `0`.
- `isNegative(-3)` returns `1`.
#### 6. Clearing Specific Bits
**Method:**
```java
public static

Transcribed Image Text:For example, say I asked you to write a function named `oneIsOne` that sets bit one of an integer to one while leaving the rest of the bits unchanged. My code for that function would look like this:
```java
public static int oneIsOne(int x){
return x | 2;
}
```
Explanation:
In this code snippet, a function named `oneIsOne` is defined. The function takes an integer `x` as an argument and returns an integer. The operation `x | 2` uses the bitwise OR operator to set the second least significant bit (bit one) of the integer `x` to 1. The rest of the bits in the integer remain unchanged.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
Recommended textbooks for you
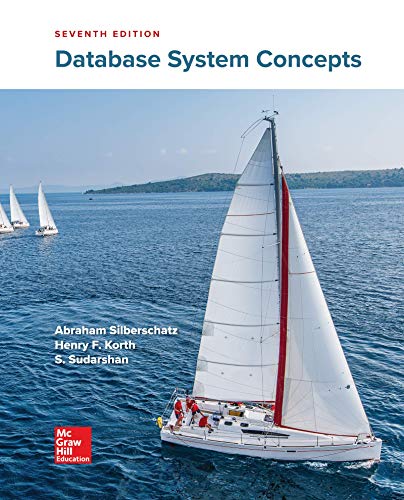
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
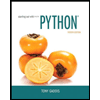
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
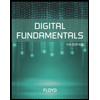
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
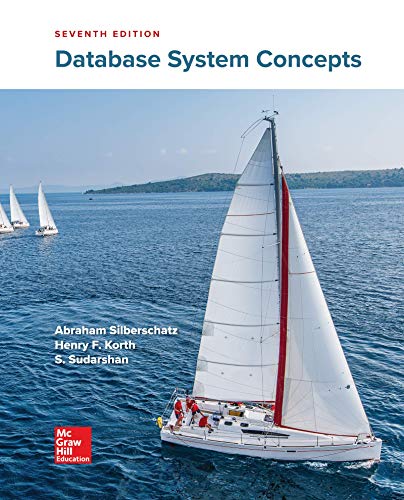
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
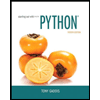
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
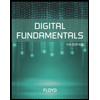
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
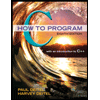
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
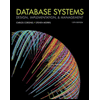
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
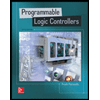
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education