Have a Python program ask the user to enter the number of times they ran around a track (laps). Next, use a 'for' or a 'while' loop to have the user enter the time it took to run each lap. When the loop is finished, display the total number of laps they ran and the total time it took them to run all laps. Label the time numbers in seconds. (Do not use 'if' or 'else' please, I have not learned about that yet so I want to stay simple). Here is my current code, it isn't working because it will not return the total number of laps the user inputs and the total time for each lap. It keeps saying 'You ran 1 laps, total time spent:" whatever the last number is, not the total time. # Display the variables for lap and time spent total_lap = 0 total_time = 0 # Have the user input how many laps they ran num_lap = int(input("Input the number of laps: ")) # Create a while loop to have the user input the time spent on all laps for lap in range(1, num_lap + 1): lap_time = float(input(f"Enter the amount of seconds spent for lap {lap}: ")) # Combine all laps total_lap += 1 # Combine lap time and total time spent total_time += lap_time lap += 1 # Show end result and have the units at the end print(f"You ran {total_lap} laps") print(f"Total time spent: {total_time} seconds{'s' * (total_time != 1)}") What can I do to fix this code so it works properly? Thanks!
Types of Loop
Loops are the elements of programming in which a part of code is repeated a particular number of times. Loop executes the series of statements many times till the conditional statement becomes false.
Loops
Any task which is repeated more than one time is called a loop. Basically, loops can be divided into three types as while, do-while and for loop. There are so many programming languages like C, C++, JAVA, PYTHON, and many more where looping statements can be used for repetitive execution.
While Loop
Loop is a feature in the programming language. It helps us to execute a set of instructions regularly. The block of code executes until some conditions provided within that Loop are true.
Hello! I am independently learning about functions on my own. I am trying to challenge myself when writing Python to create this: I will write it out specifically so you know how I am basing my code,
Have a Python program ask the user to enter the number of times they ran around a track (laps). Next, use a 'for' or a 'while' loop to have the user enter the time it took to run each lap. When the loop is finished, display the total number of laps they ran and the total time it took them to run all laps. Label the time numbers in seconds. (Do not use 'if' or 'else' please, I have not learned about that yet so I want to stay simple).
Here is my current code, it isn't working because it will not return the total number of laps the user inputs and the total time for each lap. It keeps saying 'You ran 1 laps, total time spent:" whatever the last number is, not the total time.
# Display the variables for lap and time spent
total_lap = 0
total_time = 0
# Have the user input how many laps they ran
num_lap = int(input("Input the number of laps: "))
# Create a while loop to have the user input the time spent on all laps
for lap in range(1, num_lap + 1):
lap_time = float(input(f"Enter the amount of seconds spent for lap {lap}: "))
# Combine all laps
total_lap += 1
# Combine lap time and total time spent
total_time += lap_time
lap += 1
# Show end result and have the units at the end
print(f"You ran {total_lap} laps")
print(f"Total time spent: {total_time} seconds{'s' * (total_time != 1)}")
What can I do to fix this code so it works properly? Thanks!

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 2 images

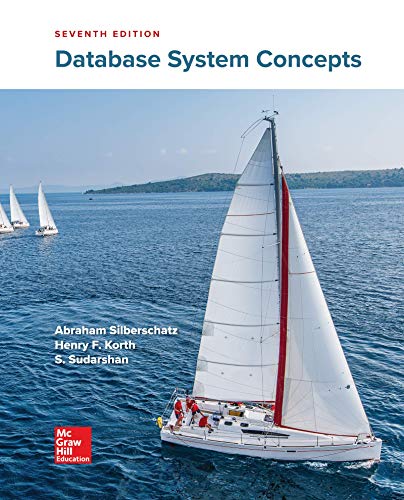
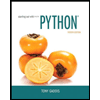
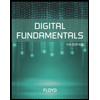
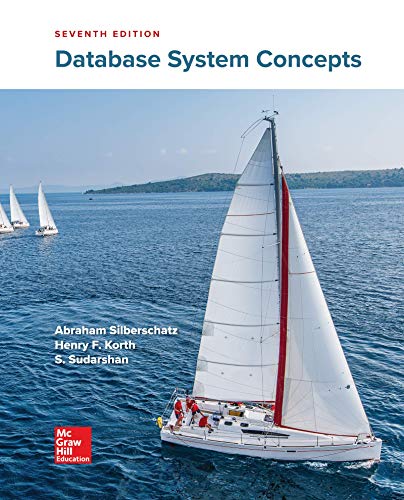
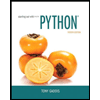
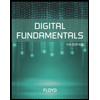
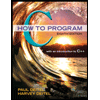
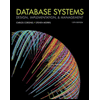
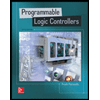