Can you help me write a C++ program to do the following because I am having trouble doing it. Create a class Record that holds all of the data for one student: Last name First name Array of 3 grades Average grade Letter grade Make the data private. Create a method (member function or operator) to read one Record Create a method (member function or operator) to display one Record Create a member function to calculate average grade and letter grade for one Record Create a member function that returns the last name of a Record That’s the end of the class. Remember to end the class definition with }; In the main function, first create a vector of Records and a single Record variable Open the file, read each Record from the file “grades.txt” (this is a txt file I already have) into your single Record variable, then push that Record onto the vector. Close the file. Tell the user how many records you read. Go through each element of the vector and call your calculation function. Now you have a vector full of fully-calculated data. Create a loop where a user can input a last name , and, if you have any students where the last name in the record matches the input last name , you display the record.
Can you help me write a C++ program to do the following because I am having trouble doing it.
Create a class Record that holds all of the data for one student:
- Last name
- First name
- Array of 3 grades
- Average grade
- Letter grade
Make the data private.
Create a method (member function or operator) to read one Record
Create a method (member function or operator) to display one Record
Create a member function to calculate average grade and letter grade for one Record
Create a member function that returns the last name of a Record
That’s the end of the class. Remember to end the class definition with };
In the main function, first create a
Open the file, read each Record from the file “grades.txt” (this is a txt file I already have) into your single Record variable, then push that Record onto the vector. Close the file. Tell the user how many records you read.
Go through each element of the vector and call your calculation function.
Now you have a vector full of fully-calculated data. Create a loop where a user can input a last name , and, if you have any students where the last name in the record matches the input last name , you display the record.

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 3 images

I had an error with grades[i] = stoi because it is saying that stoi is not declared. What should I do to fix this?
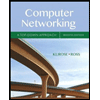
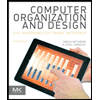
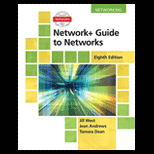
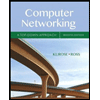
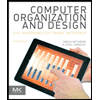
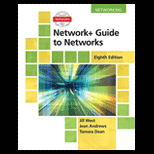
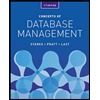
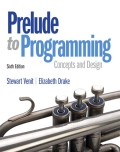
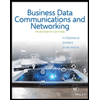