H26.CPP ---------------------------------------------------------------- #include #include #include using namespace std; #include "h26.h" // Add your code here PLEASE WRITE THE CODE HERE //////////////////////// STUDENT TESTING ////////////////////////// #include #include int run() { cout << "Add your own tests here" << endl; // istringstream in("8 9 Q 4 5"); // FlexArray a; // in >> a; // cout << "a->" << a << endl; return 0; } H26.H #ifndef H26_H_ #define H26_H_ #include #include const size_t INITIAL_CAPACITY = 2; struct FlexArray { size_t size_ = 0; std::unique_ptr data_; }; /** * Read integers from a stream into a FlexArray. * @param[in] in the stream to read from. * @param[out] the FlexArray to store the data in * @return a reference to the modified FlexArray * @post size_ will contain the number of elements * @post data_ will contain exactly size_ elements * @post in will be at end of file or a non-integer */ FlexArray& readData(std::istream& in, FlexArray& a); /** * Return a string representation of a FlexArray. * @param a the array to represent. * @return a comma separated, brace delimited contents. */ std::string toString(const FlexArray& a); inline std::ostream& operator<<(std::ostream& out, const FlexArray& a) { out << toString(a); return out; } inline std::istream& operator>>(std::istream& in, FlexArray& a) { readData(in, a); return in; } #endif
H26.CPP
----------------------------------------------------------------
#include <string>
#include <iostream>
#include <memory>
using namespace std;
#include "h26.h"
// Add your code here
PLEASE WRITE THE CODE HERE
//////////////////////// STUDENT TESTING //////////////////////////
#include <iostream>
#include <sstream>
int run()
{
cout << "Add your own tests here" << endl;
// istringstream in("8 9 Q 4 5");
// FlexArray a;
// in >> a;
// cout << "a->" << a << endl;
return 0;
}
H26.H
#ifndef H26_H_
#define H26_H_
#include <iostream>
#include <memory>
const size_t INITIAL_CAPACITY = 2;
struct FlexArray
{
size_t size_ = 0;
std::unique_ptr<int[]> data_;
};
/**
* Read integers from a stream into a FlexArray.
* @param[in] in the stream to read from.
* @param[out] the FlexArray to store the data in
* @return a reference to the modified FlexArray
* @post size_ will contain the number of elements
* @post data_ will contain exactly size_ elements
* @post in will be at end of file or a non-integer
*/
FlexArray& readData(std::istream& in, FlexArray& a);
/**
* Return a string representation of a FlexArray.
* @param a the array to represent.
* @return a comma separated, brace delimited contents.
*/
std::string toString(const FlexArray& a);
inline std::ostream& operator<<(std::ostream& out, const FlexArray& a)
{
out << toString(a);
return out;
}
inline std::istream& operator>>(std::istream& in, FlexArray& a)
{
readData(in, a);
return in;
}
#endif



Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

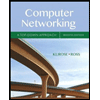
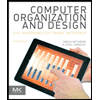
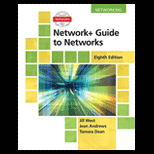
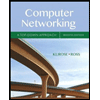
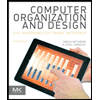
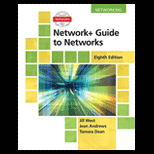
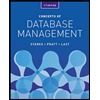
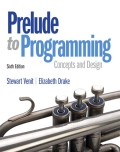
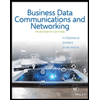