grades.c grades.c will contain 2 functions, grade and gradePrint. grade will do the following: • Receive 9 positive integers. The first 4 integers will be the student average for attendance, assignments, programs and quizzes. The 5 integer will be the final exam grade. The last 4 integers will represent the percentage values for the attendance, assignments, programs, and quizzes. • Return an integer that will be the final grade for the course. . If any grade received is less than 0 or more than 105, the function will return -1. If any percentage received is less than 0 or more than 100, the function will return . • • The sum of all the percentages can not exceed 100. If they do, the function will return-3. • The percentage for the final will be computed as the difference between the sum of the percentages received and 100. gradePrint will do the following: • Receive an integer that represents the numeric grade for the course. . If the integer received is not in the range of 0-105, then the error message"## is not a valid grade", where ## is the value received. It will then exit with a value of 1 • Convert the course numeric grade into a letter grade. See the class syllabus final grade assignments to do the conversion. Note: A+ goes to a grade of 105 Display the course grade and a message using the following and return a value of 0: • • grade A+, A, or A- Message: Excellent grade B+, B, or B- Message: Good grade C+, C, or C- Message: Fair grade D+, D, or D- Message: Poor grade F Message: Failing Grade
[This is not a graded assignment! ]
Please help me modify this code. It is complete and it runs perfectly :], but does not follow the assignment.
Main
#include <stdio.h>
#include "grades.h"
int main() {
Student students[] = {
{"Adams, Tom", {95, 93, 95, 99, 94}, 90, 85, 80, 75},
{"Curry, Jane", {95, 86, 88, 87, 89}, 90, 85, 80, 75},
{"Franklin, John", {70, 50, 65, 50, 57}, 90, 85, 80, 75},
{"George, Pat", {85, 78, 81, 82, 88}, 90, 85, 80, 75},
{"Keene, Mary", {85, 72, 75, 68, 75}, 90, 85, 80, 75},
{"Kraft, Martin", {80, 85, 80, 88, 93}, 90, 85, 80, 75},
{"Martin, James", {75, 65, 65, 52, 55}, 90, 85, 80, 75},
{"Oakley, Ann", {95, 85, 95, 88, 92}, 90, 85, 80, 75},
{"Smith, Luke", {95, 85, 75, 81, 75}, 90, 85, 80, 75},
};
int num_students = sizeof(students) / sizeof(Student);
int i;
for (i = 0; i < num_students; i++) {
Student student = students[i];
double grade = calculate_final_grade(&student);
char* letter_grade = get_letter_grade(grade);
char message[50];
get_message(letter_grade, message);
printf("%s %s\n", student.name, message);
}
return 0;
}
Grade.h
#include <stdio.h>
#include "grades.h"
int main() {
Student students[] = {
{"Adams, Tom", {95, 93, 95, 99, 94}, 90, 85, 80, 75},
{"Curry, Jane", {95, 86, 88, 87, 89}, 90, 85, 80, 75},
{"Franklin, John", {70, 50, 65, 50, 57}, 90, 85, 80, 75},
{"George, Pat", {85, 78, 81, 82, 88}, 90, 85, 80, 75},
{"Keene, Mary", {85, 72, 75, 68, 75}, 90, 85, 80, 75},
{"Kraft, Martin", {80, 85, 80, 88, 93}, 90, 85, 80, 75},
{"Martin, James", {75, 65, 65, 52, 55}, 90, 85, 80, 75},
{"Oakley, Ann", {95, 85, 95, 88, 92}, 90, 85, 80, 75},
{"Smith, Luke", {95, 85, 75, 81, 75}, 90, 85, 80, 75},
};
int num_students = sizeof(students) / sizeof(Student);
int i;
for (i = 0; i < num_students; i++) {
Student student = students[i];
double grade = calculate_final_grade(&student);
char* letter_grade = get_letter_grade(grade);
char message[50];
get_message(letter_grade, message);
printf("%s %s\n", student.name, message);
}
return 0;
}
grades.c
#include <stdlib.h>
#include <stdio.h>
#include "grades.h"
double calculate_final_grade(Student* student) {
double assignment_weight = 0.40;
double midterm_weight = 0.15;
double final_exam_weight = 0.35;
double participation_weight = 0.10;
double total_grade = 0.0;
int i;
for (i = 0; i < 5; i++) {
total_grade += student->grades[i];
}
double average_grade = total_grade / 5.0;
total_grade = (average_grade * assignment_weight) + (student->midterm * midterm_weight)
+ (student->final_exam * final_exam_weight) + (student->participation * participation_weight);
return total_grade;
}
char* get_letter_grade(double grade) {
if (grade >= 90.0) {
return "A";
} else if (grade >= 80.0) {
return "B";
} else if (grade >= 70.0) {
return "C";
} else if (grade >= 60.0) {
return "D";
} else {
return "F";
}
}
void get_message(char* letter_grade, char* message) {
if (letter_grade[0] == 'A') {
sprintf(message, "is doing excellent work");
} else if (letter_grade[0] == 'B') {
sprintf(message, "is doing good work");
} else if (letter_grade[0] == 'C') {
sprintf(message, "is doing satisfactory work");
} else if (letter_grade[0] == 'D') {
sprintf(message, "is doing below average work");
} else {
sprintf(message, "is failing the course");
}
}
Compile the 3 codes to a makefile to follow the example!
Thank you, much appreicated



Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 1 images

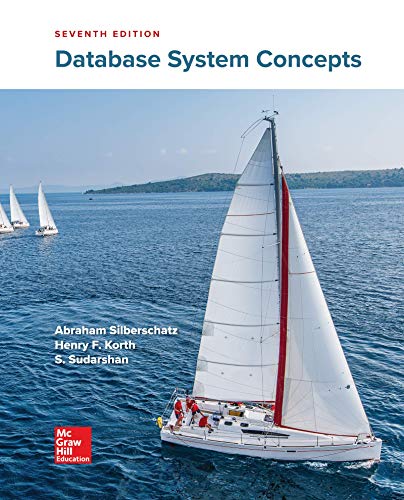
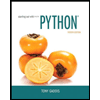
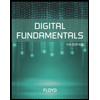
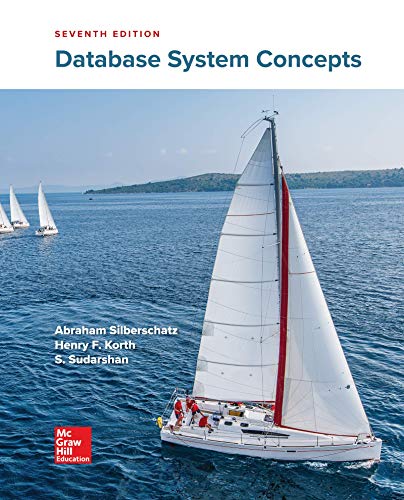
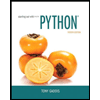
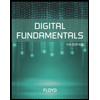
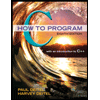
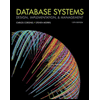
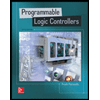