/** * Given two integers, the second larger than the first, this program: * 1) prints the even numbers between the first and second number inclusive * 2) prints the sum of those even numbers * 3) prints the odd numbers between the first and second number inclusive * 4) prints the sum of those odd numbers * * Example: * Enter an integer: 10 * Enter one larger than the first: 20 * Even numbers: 10 12 14 16 18 20 * Sum of even numbers = 90 * Odd numbers: 11 13 15 17 19 * Sum of odd numbers = 75
/** * Given two integers, the second larger than the first, this program: * 1) prints the even numbers between the first and second number inclusive * 2) prints the sum of those even numbers * 3) prints the odd numbers between the first and second number inclusive * 4) prints the sum of those odd numbers * * Example: * Enter an integer: 10 * Enter one larger than the first: 20 * Even numbers: 10 12 14 16 18 20 * Sum of even numbers = 90 * Odd numbers: 11 13 15 17 19 * Sum of odd numbers = 75
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
/**
* Given two integers, the second larger than the first, this program:
* 1) prints the even numbers between the first and second number inclusive
* 2) prints the sum of those even numbers
* 3) prints the odd numbers between the first and second number inclusive
* 4) prints the sum of those odd numbers
*
* Example:
* Enter an integer: 10
* Enter one larger than the first: 20
* Even numbers: 10 12 14 16 18 20
* Sum of even numbers = 90
* Odd numbers: 11 13 15 17 19
* Sum of odd numbers = 75
****This version uses WHILE loops only****
![### Implement the Pseudocode of the Preceding Problem: Reverse a String
Below is the implementation of the pseudocode for reversing a string entered by the user.
---
#### Reverse.java
```java
1 import java.util.Scanner;
2
3 public class Reverse
4 {
5 public static void main(String[] args)
6 {
7 Scanner in = new Scanner(System.in);
8
9 System.out.print("Enter a word: ");
10 String s = in.nextLine();
11 String r = "" ;
12 int i = 0;
13 while (i < s.length())
14 {
15 char c = s.charAt(i);
16 r = c + r;
17 i++;
18 }
19
20 System.out.print(r);
21 }
22 }
```
---
#### Testing Reverse.java
##### Test 1
- **Actual Output:**
```
Enter a word: Timed out after 5 seconds
```
- **Expected Output:**
```
Enter a word: derF
```
- **Result:**
Fail
##### Test 2
- **Actual Output:**
```
Enter a word: Timed out after 5 seconds
```
- **Expected Output:**
```
Enter a word: ippississiM
```
- **Result:**
Fail
---
### Explanation:
1. **Code Explanation:**
- **Lines 1-2:** Import the `Scanner` class for user input.
- **Lines 3-6:** Define the `Reverse` class and the `main` method.
- **Line 7:** Create a `Scanner` object for input.
- **Lines 9-10:** Prompt the user to enter a word and store the input in the string `s`.
- **Line 11:** Initialize an empty string `r`.
- **Line 12:** Initialize an integer `i` to 0 for indexing.
- **Lines 13-18:** Use a while loop to iterate through each character in the string `s`:
- **Line 15:** Get the character at index `i`.
- **Line 16:** Concatenate the character to the beginning of `r`.
- **Line 17:** Increment the index `i`.
- **Line 20:** Print the reversed string](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fd772767f-fd91-4ca5-b737-b5766e3fef0a%2F91b3d0f2-1387-447a-8d19-29f55b8de6bc%2Fkxbnelc.png&w=3840&q=75)
Transcribed Image Text:### Implement the Pseudocode of the Preceding Problem: Reverse a String
Below is the implementation of the pseudocode for reversing a string entered by the user.
---
#### Reverse.java
```java
1 import java.util.Scanner;
2
3 public class Reverse
4 {
5 public static void main(String[] args)
6 {
7 Scanner in = new Scanner(System.in);
8
9 System.out.print("Enter a word: ");
10 String s = in.nextLine();
11 String r = "" ;
12 int i = 0;
13 while (i < s.length())
14 {
15 char c = s.charAt(i);
16 r = c + r;
17 i++;
18 }
19
20 System.out.print(r);
21 }
22 }
```
---
#### Testing Reverse.java
##### Test 1
- **Actual Output:**
```
Enter a word: Timed out after 5 seconds
```
- **Expected Output:**
```
Enter a word: derF
```
- **Result:**
Fail
##### Test 2
- **Actual Output:**
```
Enter a word: Timed out after 5 seconds
```
- **Expected Output:**
```
Enter a word: ippississiM
```
- **Result:**
Fail
---
### Explanation:
1. **Code Explanation:**
- **Lines 1-2:** Import the `Scanner` class for user input.
- **Lines 3-6:** Define the `Reverse` class and the `main` method.
- **Line 7:** Create a `Scanner` object for input.
- **Lines 9-10:** Prompt the user to enter a word and store the input in the string `s`.
- **Line 11:** Initialize an empty string `r`.
- **Line 12:** Initialize an integer `i` to 0 for indexing.
- **Lines 13-18:** Use a while loop to iterate through each character in the string `s`:
- **Line 15:** Get the character at index `i`.
- **Line 16:** Concatenate the character to the beginning of `r`.
- **Line 17:** Increment the index `i`.
- **Line 20:** Print the reversed string
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
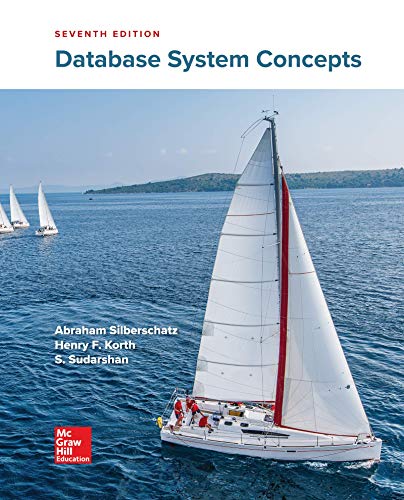
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
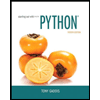
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
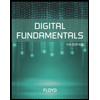
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
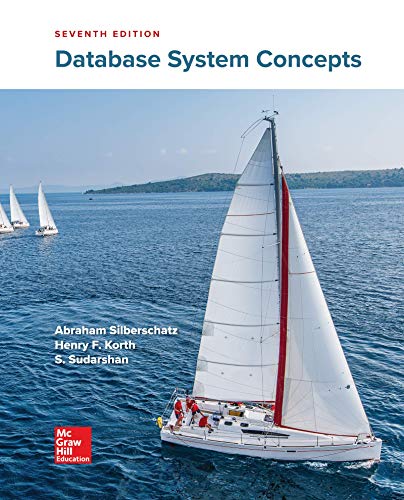
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
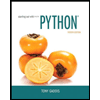
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
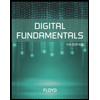
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
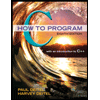
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
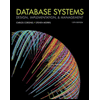
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
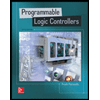
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education