Given: The formula to calculate the weight of an object when the mass will be known, WEIGHT = MASS * 9.8 Purpose of the solution: To verify if a given object is too heavy or light on the basis of the computed weight value. Task: Design a Flowchart that asks the user to enter an object’s mass, and then calculates its weight. If the object weighs more than 1,000 Newtons, display a message indicating that it is too heavy. If the object weighs less than 10 Newtons, display a message indicating that it is too light.. Source Code: import java.util.Scanner; public class Main { /*This application requires the amount of mass of an object, calculates the weight, using the formula, Weight = Mass × 9.8, and verify if the object is too heavy or light.*/ // return the amount of weight public static double computeWeight(double mass) { double consVal = 9.8; return mass*consVal; } public static void main(String[] args) { //ask user input System.out.println("Enter the mass of the object:"); Scanner scan = new Scanner(System.in); double massObj = scan.nextDouble(); // call method computing weight double weight = computeWeight(massObj); //verify if too heavy or light if(weight>1000) { System.out.println("The object is too heavy"); } else if (weight<10) { System.out.println("The object is too light"); } else { System.out.println("The weight of the object: "+weight+"N"); } } }
Given: The formula to calculate the weight of an object when the mass will be known, WEIGHT = MASS * 9.8
Purpose of the solution: To verify if a given object is too heavy or light on the basis of the computed weight value.
Task: Design a Flowchart that asks the user to enter an object’s mass, and then calculates its weight. If the object weighs more than 1,000 Newtons, display a message indicating that it is too heavy. If the object weighs less than 10 Newtons, display a message indicating that it is too light..
Source Code:
import java.util.Scanner;
public class Main
{
/*This application requires the amount of mass of an object,
calculates the weight, using the formula, Weight = Mass × 9.8,
and verify if the object is too heavy or light.*/
// return the amount of weight
public static double computeWeight(double mass)
{
double consVal = 9.8;
return mass*consVal;
}
public static void main(String[] args) {
//ask user input
System.out.println("Enter the mass of the object:");
Scanner scan = new Scanner(System.in);
double massObj = scan.nextDouble();
// call method computing weight
double weight = computeWeight(massObj);
//verify if too heavy or light
if(weight>1000)
{
System.out.println("The object is too heavy");
}
else if (weight<10)
{
System.out.println("The object is too light");
}
else
{
System.out.println("The weight of the object: "+weight+"N");
}
}
}

Step by step
Solved in 2 steps with 1 images

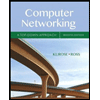
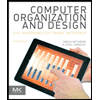
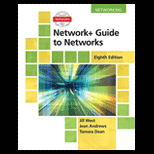
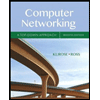
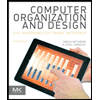
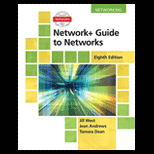
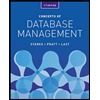
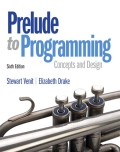
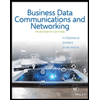