Given a positive integer n, the following rules will always create a sequence that ends with 1, called the hailstone sequence: If n is even, divide it by 2 If n is odd, multiply it by 3 and add 1 (i.e. 3n +1) Continue until n is 1 Write a program that reads an integer as input and prints the hailstone sequence starting with the integer entered. Format the output so that ten integers, each separated by a tab character (\t), are printed per line. The output format can be achieved as follows: print(n, end='\t') Ex: If the input is: 25 the output is: 25 76 38 19 58 29 88 44 22 11 34 17 52 26 13 40 20 10 5 16 8 4 2 1 My Code: n = int(input()) print(n, end='\t') i = 1 while n > 1: if not i % 10: print(n, end='\n') if n % 2 == 0: n = n//2 print(n, end='\t') else: n = 3*n+1 print(n, end='\t') i += 1 print() Output: Screenshot attached!
4.15 LAB: Hailstone sequence
Given a positive integer n, the following rules will always create a sequence that ends with 1, called the hailstone sequence:
- If n is even, divide it by 2
- If n is odd, multiply it by 3 and add 1 (i.e. 3n +1)
- Continue until n is 1
Write a program that reads an integer as input and prints the hailstone sequence starting with the integer entered. Format the output so that ten integers, each separated by a tab character (\t), are printed per line.
The output format can be achieved as follows:
print(n, end='\t')
Ex: If the input is:
25
the output is:
25 76 38 19 58 29 88 44 22 11
34 17 52 26 13 40 20 10 5 16
8 4 2 1
My Code:
n = int(input())
print(n, end='\t')
i = 1
while n > 1:
if not i % 10:
print(n, end='\n')
if n % 2 == 0:
n = n//2
print(n, end='\t')
else:
n = 3*n+1
print(n, end='\t')
i += 1
print()
Output:
Screenshot attached!


Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

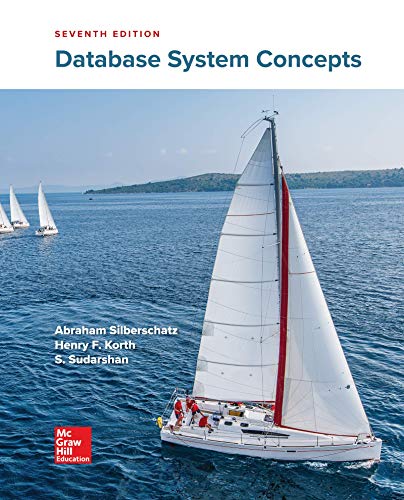
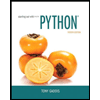
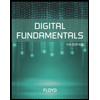
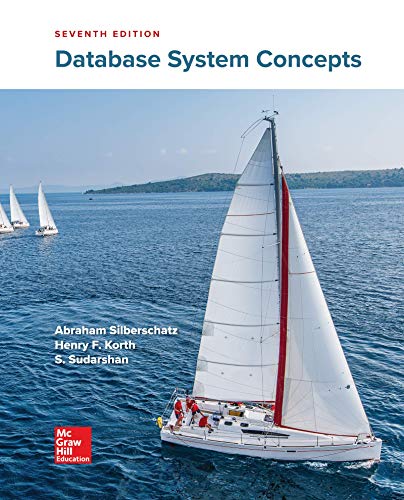
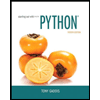
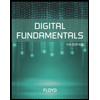
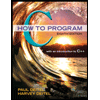
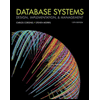
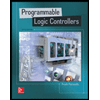