from dateutil import parser import datetime from datetime import datetime usernames = [] passwords = [] def reg_user(): """Return for registering new user""" withopen('user.txt', 'a') asfile1: iflogin_username == "admin": new_username = input("Enter a username: ") whilenew_usernameinusernames: print("This username already exists.") new_username = input("Enter a username: ") new_password = input("Enter a password: ") confirm_password = input("Re-enter the password: ") whileconfirm_password != new_password: print("Passwords do not match.") new_password = input("Enter your password: ") confirm_password = input("Re-enter your password: ") else: print("Welcome ", new_username) whilenew_passwordinpasswords: print("This password already exists.") new_password = input("Enter a password: ") confirm_password = input("Re-enter the password: ") else: print("User not authorised to register a new user.") return file1.writelines(f"\n{new_username}, {new_password}") def add_task(): """Return for adding task""" withopen('tasks.txt', 'a+') asfile2: assigned_user = input("Who is this task assigned to: ") task_title = input("Title of task: ") task_description = input("Description of the task: ") task_due = input("When is this task due (DD MMM YYYY): ") the_date_today = datetime.today() current_date = the_date_today.strftime("%d %B %Y") completed_question = "No" file2.write(f"\n{assigned_user}, {task_title}, {task_description}, {task_due}, {current_date}, {completed_question}") return() def view_all(): """Return for viewing all tasks""" withopen('tasks.txt', 'r') asfile3: withopen('tasks.txt', 'r') asfile4: forlineinfile3: line = line.strip() line = line.split(", ") print("--------------------------------------------------------------") print("Task:\t\t\t" + line[1]) print("Assigned to:\t\t" + line[0]) print("Date assigned:\t\t" + line[3]) print("Due date:\t\t" + line[4]) print("Task compelete?\t\t" + line[5]) print("Task description:\n" + line[2] + "\n") print("-------------------------------------------------------------") return() def view_mine(): """Return for viewing task for the user loged in""" withopen('tasks.txt','r+') asfile5: view_tasks = file5.read().strip().split("\n") forindex, lineinenumerate(view_tasks): task_lines = line.strip("\n").split(", ") iftask_lines[0] == login_username: print(f"Task Number:\t{index}\nAssigned to:\t{task_lines[0]}\nTitle:\t\t{task_lines[1]}\nDescription:\t{task_lines[2]}\nDate assigned:\t{task_lines[3]}\nDue date:\t{task_lines[4]}\nTask completed?\t{task_lines[5]}\n") else: print("You are not authorised to see the task list.") fori, linesinenumerate(view_tasks): new_line = lines.strip("\n").split(", ") ifnew_line[0] == login_username: the_choice = input("Would you like to mark your task as complete = 'c' or edit your tasks = 'e', or return back to main menu = '-1': ").lower() ifthe_choice == "-1": exit() elifthe_choice == "c": task_number = (input("Enter the number of which task number you would like to mark as complete: ").strip().split("\n")) if i == task_number: if new_line[5] == "No": new_line[5] = "Yes" print(new_line) with open('user.txt', 'r') as file1: forlinesinfile1: temp = lines.strip() temp = temp.split(", ") usernames.append(temp[0]) passwords.append(temp[1]) login_username = input("Enter your username: ") while login_username not in usernames: print("This username does not exist.") login_username = input("Enter your username: ") login_password = input("Enter your password: ") while login_password not in passwords: print("This password is not valid.") login_password = input("Enter your password: ") while True: iflogin_username == "admin": menu = input('''Select one of the following Options below: r - Registering a user a - Adding a task va - View all tasks vm - view my task gr - generate reports ds - display statistics e - Exit : ''').lower() else: menu = input('''Select one of the following Options below: a - Adding a task va - View all tasks vm - view my task e - Exit : ''').lower() ifmenu == 'r': reg_user() elifmenu == 'a': add_task() elifmenu == 'va': view_all() elifmenu == 'vm': view_mine() tasks.txt: admin, Register Users with taskManager.py, Use taskManager.py to add the usernames and passwords for all team members that will be using this program., 10 Oct 2019, 20 Oct 2019, No admin, Assign initial tasks, Use taskManager.py to assign each team member with appropriate tasks, 10 Oct 2019, 25 Oct 2019, No user.txt: admin, adm1n Question: For def view_mine() I need to the user to be able to select which task they are going to choose to edit or mark at complete. I am using index, but it will only let the tasks be edited the tasks in the index order. how do i make it that the user can edit any task.
from dateutil import parser
import datetime
from datetime import datetime
usernames = []
passwords = []
def reg_user():
"""Return for registering new user"""
withopen('user.txt', 'a') asfile1:
iflogin_username == "admin":
new_username = input("Enter a username: ")
whilenew_usernameinusernames:
print("This username already exists.")
new_username = input("Enter a username: ")
new_password = input("Enter a password: ")
confirm_password = input("Re-enter the password: ")
whileconfirm_password != new_password:
print("Passwords do not match.")
new_password = input("Enter your password: ")
confirm_password = input("Re-enter your password: ")
else:
print("Welcome ", new_username)
whilenew_passwordinpasswords:
print("This password already exists.")
new_password = input("Enter a password: ")
confirm_password = input("Re-enter the password: ")
else:
print("User not authorised to register a new user.")
return
file1.writelines(f"\n{new_username}, {new_password}")
def add_task():
"""Return for adding task"""
withopen('tasks.txt', 'a+') asfile2:
assigned_user = input("Who is this task assigned to: ")
task_title = input("Title of task: ")
task_description = input("Description of the task: ")
task_due = input("When is this task due (DD MMM YYYY): ")
the_date_today = datetime.today()
current_date = the_date_today.strftime("%d %B %Y")
completed_question = "No"
file2.write(f"\n{assigned_user}, {task_title}, {task_description}, {task_due}, {current_date}, {completed_question}")
return()
def view_all():
"""Return for viewing all tasks"""
withopen('tasks.txt', 'r') asfile3:
withopen('tasks.txt', 'r') asfile4:
forlineinfile3:
line = line.strip()
line = line.split(", ")
print("--------------------------------------------------------------")
print("Task:\t\t\t" + line[1])
print("Assigned to:\t\t" + line[0])
print("Date assigned:\t\t" + line[3])
print("Due date:\t\t" + line[4])
print("Task compelete?\t\t" + line[5])
print("Task description:\n" + line[2] + "\n")
print("-------------------------------------------------------------")
return()
def view_mine():
"""Return for viewing task for the user loged in"""
withopen('tasks.txt','r+') asfile5:
view_tasks = file5.read().strip().split("\n")
forindex, lineinenumerate(view_tasks):
task_lines = line.strip("\n").split(", ")
iftask_lines[0] == login_username:
print(f"Task Number:\t{index}\nAssigned to:\t{task_lines[0]}\nTitle:\t\t{task_lines[1]}\nDescription:\t{task_lines[2]}\nDate assigned:\t{task_lines[3]}\nDue date:\t{task_lines[4]}\nTask completed?\t{task_lines[5]}\n")
else:
print("You are not authorised to see the task list.")
fori, linesinenumerate(view_tasks):
new_line = lines.strip("\n").split(", ")
ifnew_line[0] == login_username:
the_choice = input("Would you like to mark your task as complete = 'c' or edit your tasks = 'e', or return back to main menu = '-1': ").lower()
ifthe_choice == "-1":
exit()
elifthe_choice == "c":
task_number = (input("Enter the number of which task number you would like to mark as complete: ").strip().split("\n"))
if i == task_number:
if new_line[5] == "No":
new_line[5] = "Yes"
print(new_line)
with open('user.txt', 'r') as file1:
forlinesinfile1:
temp = lines.strip()
temp = temp.split(", ")
usernames.append(temp[0])
passwords.append(temp[1])
login_username = input("Enter your username: ")
while login_username not in usernames:
print("This username does not exist.")
login_username = input("Enter your username: ")
login_password = input("Enter your password: ")
while login_password not in passwords:
print("This password is not valid.")
login_password = input("Enter your password: ")
while True:
iflogin_username == "admin":
menu = input('''Select one of the following Options below:
r - Registering a user
a - Adding a task
va - View all tasks
vm - view my task
gr - generate reports
ds - display statistics
e - Exit
: ''').lower()
else:
menu = input('''Select one of the following Options below:
a - Adding a task
va - View all tasks
vm - view my task
e - Exit
: ''').lower()
ifmenu == 'r':
reg_user()
elifmenu == 'a':
add_task()
elifmenu == 'va':
view_all()
elifmenu == 'vm':
view_mine()
tasks.txt:
admin, Register Users with taskManager.py, Use taskManager.py to add the usernames and passwords for all team members that will be using this program., 10 Oct 2019, 20 Oct 2019, No
admin, Assign initial tasks, Use taskManager.py to assign each team member with appropriate tasks, 10 Oct 2019, 25 Oct 2019, No
user.txt:
admin, adm1n
Question:
For def view_mine()
I need to the user to be able to select which task they are going to choose to edit or mark at complete.
I am using index, but it will only let the tasks be edited the tasks in the index order.
how do i make it that the user can edit any task.


Step by step
Solved in 3 steps

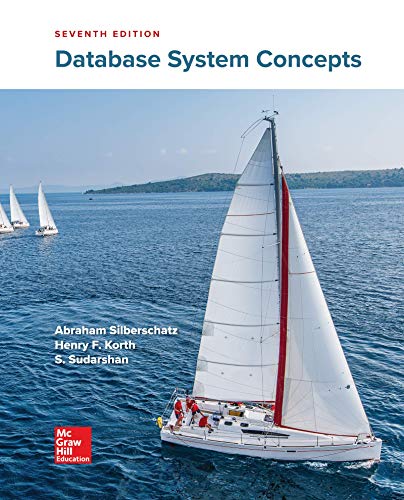
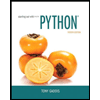
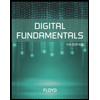
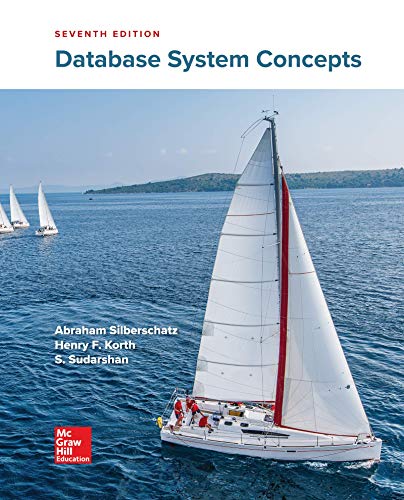
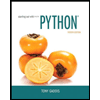
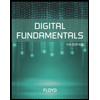
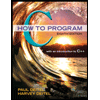
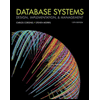
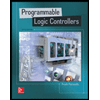