For this question, you will be required to use the binary search to find the root of some function f(x)f(x) on the domain x∈[a,b]x∈[a,b] by continuously bisecting the domain. In our case, the root of the function can be defined as the x-values where the function will return 0, i.e. f(x)=0f(x)=0 For example, for the function: f(x)=sin2(x)x2−2f(x)=sin2(x)x2−2 on the domain [0,2][0,2], the root can be found at x≈1.43x≈1.43 Constraints Stopping criteria: ∣∣f(root)∣∣<0.0001|f(root)|<0.0001 or you reach a maximum of 1000 iterations. Round your answer to two decimal places. Function specifications Argument(s): f (function) →→ mathematical expression in the form of a lambda function. domain (tuple) →→ the domain of the function given a set of two integers. MAX (int) →→ the maximum number of iterations that will be performed by the function. Return: root (float) →→ return the root (rounded to two decimals) of the given function. START FUNCTION def binary_search(f,domain, MAX = 1000): f = lambda x:(np.sin(x)**2)*(x**2)-2 domain = (0,2) x=binary_search(f,domain) x test binary_search(lambda x:(np.sin(x)**2)*(x**2)-2,(0,2))==1.43 Expert Solution arrow_forward Step 1 : Code (Explained with comment) import numpy as np def binary_search(f,domain, MAX = 1000): start ,end = domain # get the start and end point while start < end and MAX: # get the mid mid = (start + end)/2 # if we reached to root if abs(f(mid)) < 0.0001: # return rounded return round(mid,2) if f(mid) < 0: # if we are left of root start = mid # set start to mid else: # otherwise go to left end = mid MAX -= 1 # decrement max by 1 return round(mid,2)# return rounded f = lambda x:(np.sin(x)**2)*(x**2)-2 domain = (0,2) x=binary_search(f,domain) # test print(binary_search(lambda x:(np.sin(x)**2)*(x**2)-2,(0,2)) == 1.43)
For this question, you will be required to use the binary search to find the root of some function f(x)f(x) on the domain x∈[a,b]x∈[a,b] by continuously bisecting the domain. In our case, the root of the function can be defined as the x-values where the function will return 0, i.e. f(x)=0f(x)=0
For example, for the function: f(x)=sin2(x)x2−2f(x)=sin2(x)x2−2 on the domain [0,2][0,2], the root can be found at x≈1.43x≈1.43
Constraints
- Stopping criteria: ∣∣f(root)∣∣<0.0001|f(root)|<0.0001 or you reach a maximum of 1000 iterations.
- Round your answer to two decimal places.
Function specifications
Argument(s):
- f (function) →→ mathematical expression in the form of a lambda function.
- domain (tuple) →→ the domain of the function given a set of two integers.
- MAX (int) →→ the maximum number of iterations that will be performed by the function.
Return:
- root (float) →→ return the root (rounded to two decimals) of the given function.
START FUNCTION
def binary_search(f,domain, MAX = 1000):
f = lambda x:(np.sin(x)**2)*(x**2)-2
domain = (0,2)
x=binary_search(f,domain)
x
test
binary_search(lambda x:(np.sin(x)**2)*(x**2)-2,(0,2))==1.43
import numpy as np
def binary_search(f,domain, MAX = 1000):
start ,end = domain # get the start and end point
while start < end and MAX:
# get the mid
mid = (start + end)/2
# if we reached to root
if abs(f(mid)) < 0.0001:
# return rounded
return round(mid,2)
if f(mid) < 0: # if we are left of root
start = mid # set start to mid
else: # otherwise go to left
end = mid
MAX -= 1 # decrement max by 1
return round(mid,2)# return rounded
f = lambda x:(np.sin(x)**2)*(x**2)-2
domain = (0,2)
x=binary_search(f,domain)
# test
print(binary_search(lambda x:(np.sin(x)**2)*(x**2)-2,(0,2)) == 1.43)

Trending now
This is a popular solution!
Step by step
Solved in 3 steps

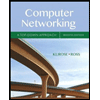
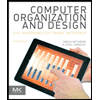
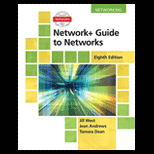
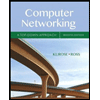
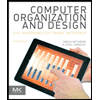
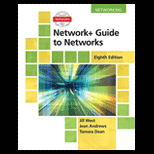
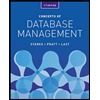
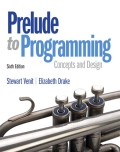
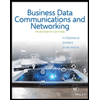