For the following problems, create one main program that will a) create 4 sets of array of characters (one to be used for each function call) b) populate each array with the same symbols, lowercase and uppercase letters and numbers. c) print the elements of the array d) call each of the functions using it's own array e) print the expected output (output varies depending on the function) Functionality of the functions 1. Convert lowercase letters to uppercase letters and print the updated array 2. print the length of the array 3. print the resulting array containing only numbers 4. print the resulting array containing only lowercase letters
using chapters 1 to 7 from the book c how to


A required program is as follows,
#include <stdio.h>
#include <string.h>
//Define a function that converts lowercase to uppercase letters
char *lowerToUpper_string(char s[]) {
int c = 0;
while (s[c] != '\0') {
//Check if array contains lower letter
if (s[c] >= 'a' && s[c] <= 'z') {
//Convert to upper
s[c] = s[c] - 32;
}
c++;
}
return s;
}
//Define a function that computes the length of the array
int computeLength(char s[])
{
int c=0;
while (s[c] != '\0') {
//Count characters in the array
c++;
}
return c;
}
//Define a function that returns array containing only numbers
char *onlynumbers(char s[])
{
int ascii;
int c=0,i=0;
while(s[c]!='\0')
{
ascii=(int)s[c];
//Check if array contains a number
if(ascii>=48&&ascii<=57)
{
s[i]=s[c];
i++;
}
c++;
}
s[i]='\0';
return s;
}
//Define a function that returns array containing only lowercase letters
char *onlyLower(char s[]) {
for (int i = 0, j; s[i] != '\0'; ++i) {
while (!(s[i] >= 'a' && s[i] <= 'z') && !(s[i] == '\0')) {
for (j = i; s[j] != '\0'; ++j) {
s[j] = s[j + 1];
}
s[j] = '\0';
}
}
return s;
}
int main()
{
// a)Create 4 sets of array of characters
// b)Populate each array with same contents
char array1[]="DavidJack89";
char array2[]="DavidJack89";
char array3[]="DavidJack89";
char array4[]="DavidJack89";
char *result1;
int result2;
char *result3;
char *result4;
//c)Print the elements of the array
printf("%s\n",array1);
printf("%s\n",array2);
printf("%s\n",array3);
printf("%s\n",array4);
/*Call the function to convert the lowercase letters to
uppercase letters and print the updated array*/
result1=lowerToUpper_string(array1);
printf("Array containing uppercase letters: %s\n\n",result1);
// 2)Call a function to print length of array
result2=computeLength(array2);
printf("Length of \"%s\" is: %d\n\n",array2,result2);
// 3)Call a function to print only numbers
result3=onlynumbers(array3);
printf("Array containing only numbers: %s\n\n",result3);
// 4)Call a function to print array containing only lowercase letters
result4=onlyLower(array4);
printf("Array containing only lowercase letters: %s",result4);
return 0;
}
Step by step
Solved in 2 steps with 1 images

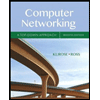
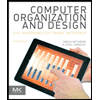
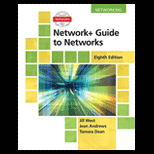
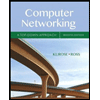
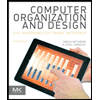
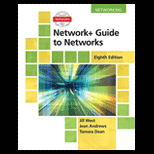
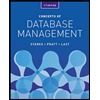
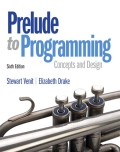
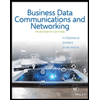