For C++: Convert the following C++ code into psuedocode: #include using namespace std; #define ARRAY_SIZE 8 void Display(int[]); void BubbleSort(int[]); void SelectionSort(int[]); int main() { int arra1[] = { 45, 58, 12, 35, 45, 87, 15, 25 }; int arra2[] = { 45, 58, 12, 35, 45, 87, 15, 25 }; cout << "First Array: \n"; Display(arra1); cout << endl; cout << "Bubble Sort: " << endl; BubbleSort(arra1); //displays second array cout << "\nSecond Array: \n"; Display(arra2); cout << endl; cout << "Selection Sort: " << endl; SelectionSort(arra2); cout << endl; return 0; } void Display(int arra[]) { for (int i = 0; i < ARRAY_SIZE; i++) cout << arra[i] << " "; cout << endl; } void BubbleSort(int arra[]) { for (int i = ARRAY_SIZE - 2; i >= 0; i--) { for (int j = 0; j <= i; j++) { if (arra[j] > arra[j + 1]) { int tmp = arra[j]; arra[j] = arra[j + 1]; arra[j + 1] = tmp; Display(arra); } } } } void SelectionSort(int arra[]) { for (int i = 0; i < ARRAY_SIZE - 1; i++) { for (int j = i + 1; j < ARRAY_SIZE; j++) if (arra[i] > arra[j]) { int tmp = arra[i]; arra[i] = arra[j]; arra[j] = tmp; Display(arra); } } }
For C++:
Convert the following C++ code into psuedocode:
#include <iostream>
using namespace std;
#define ARRAY_SIZE 8
void Display(int[]);
void BubbleSort(int[]);
void SelectionSort(int[]);
int main()
{
int arra1[] = {
45,
58,
12,
35,
45,
87,
15,
25
};
int arra2[] = {
45,
58,
12,
35,
45,
87,
15,
25
};
cout << "First Array: \n";
Display(arra1);
cout << endl;
cout << "Bubble Sort: " << endl;
BubbleSort(arra1);
//displays second array
cout << "\nSecond Array: \n";
Display(arra2);
cout << endl;
cout << "Selection Sort: " << endl;
SelectionSort(arra2);
cout << endl;
return 0;
}
void Display(int arra[]) {
for (int i = 0; i < ARRAY_SIZE; i++)
cout << arra[i] << " ";
cout << endl;
}
void BubbleSort(int arra[]) {
for (int i = ARRAY_SIZE - 2; i >= 0; i--)
{
for (int j = 0; j <= i; j++)
{
if (arra[j] > arra[j + 1])
{
int tmp = arra[j];
arra[j] = arra[j + 1];
arra[j + 1] = tmp;
Display(arra);
}
}
}
}
void SelectionSort(int arra[]) {
for (int i = 0; i < ARRAY_SIZE - 1; i++)
{
for (int j = i + 1; j < ARRAY_SIZE; j++)
if (arra[i] > arra[j])
{
int tmp = arra[i];
arra[i] = arra[j];
arra[j] = tmp;
Display(arra);
}
}
}

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

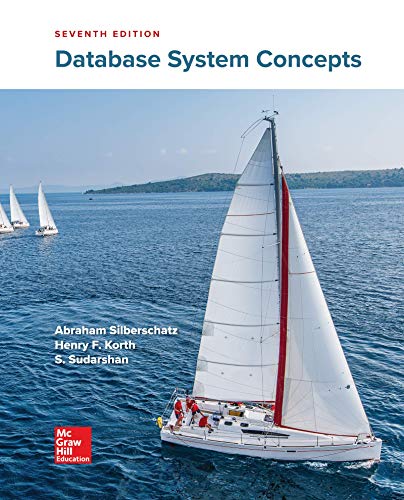
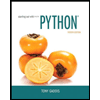
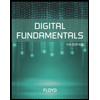
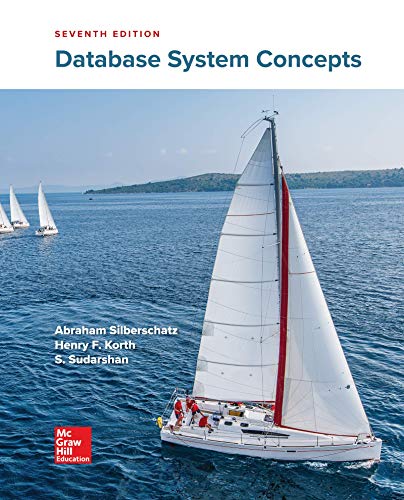
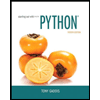
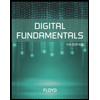
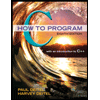
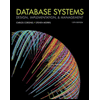
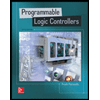