Find the value of x , y after the statements are executed: Both qestions are in the picture files
Find the value of x , y after the statements are executed: Both qestions are in the picture files
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
Question (3) 30: Find the value of x , y after the statements are executed:
Both qestions are in the picture files

Transcribed Image Text:```plaintext
x = 3;
if (x == 3)
if (2 != 2)
y = ++x;
else
y = x++;
y++;
```
### Explanation:
This code is a simple C-like programming structure with an `if-else` conditional statement and pre/post-increment operators.
- **x = 3;**: Initializes the variable `x` with the value `3`.
- **if (x == 3)**: Checks if `x` is equal to `3`. Since `x` is `3`, the condition is true, and the subsequent block is entered.
- **if (2 != 2) y = ++x;**: This checks the condition `2 != 2`, which is false. This means the code block `y = ++x;` will not execute.
- **else y = x++;**: Because the previous `if` condition is false, this `else` block executes, setting `y` to `x` (`3`) and then incrementing `x` after the assignment (`x` becomes `4`).
- **y++;**: Increments `y` by `1` after the assignment from the `else` block, making `y` equal to `4`.
This program demonstrates conditional logic and the difference between pre-increment (`++x`) and post-increment (`x++`) operators.

Transcribed Image Text:```java
y=1; x=0; boolean test=true;
if (x==0 || !test)
{
if (2==2) y=++x;
else y=++x;
y=y+2+ --x;
}
else x=2;
```
### Explanation:
This snippet is a small Java program demonstrating the use of basic control structures:
1. **Variable Initialization**:
- `y` and `x` are integer variables initialized to 1 and 0, respectively.
- `test` is a boolean variable initialized to `true`.
2. **If Statement**:
- The outer `if` condition checks if `x` equals 0 or if `test` is false. Since `x` is 0, the code inside the `if` block executes.
3. **Nested If-Else Statement**:
- Inside the first block, there is a nested `if-else` statement.
- The condition `2==2` is always true, so the `if` block executes, setting `y` to the value of `++x` (increments `x` by 1, then assigns it to `y`). Thus, `y` becomes 1 and `x` becomes 1.
4. **Arithmetic Operation**:
- `y=y+2+ --x;` decreases `x` by 1 before the addition (making `x` return to 0), then adds 2 to `y`, resulting in `y` equaling 3.
5. **Else Clause**:
- The `else` part of the outer `if` is not executed since the `if` condition was true.
This simple program illustrates conditional execution and the effects of increment and decrement operations within expressions.
Expert Solution

Step 1
Answer:
Step by step
Solved in 3 steps

Recommended textbooks for you
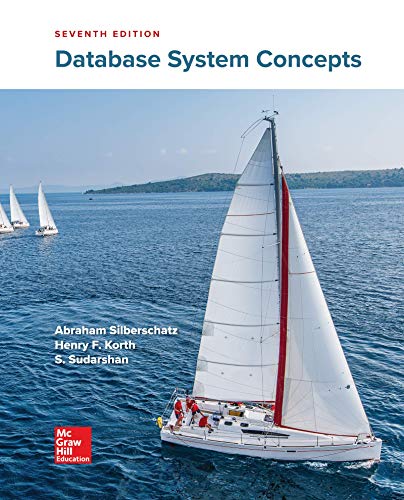
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
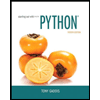
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
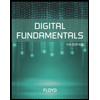
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
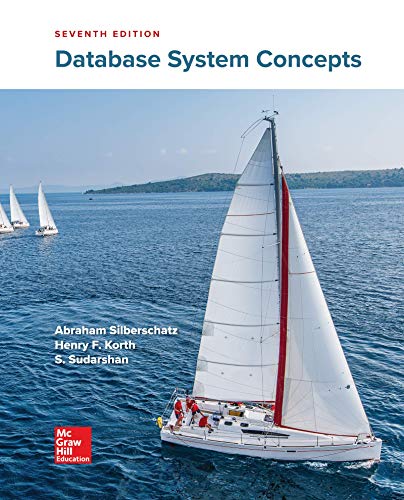
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
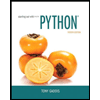
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
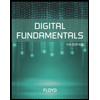
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
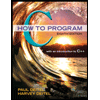
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
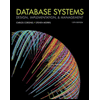
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
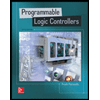
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education