File ParseInts.java contains a program that does the following: Prompts for and reads in a line of input Uses a second Scanner to take the input line one token at a time and parses an integer from each token as it is extracted. Sums the integers. Prints the sum. Save ParseInts to your directory and compile and run it. If you give it the input 10 20 30 40 it should print The sum of the integers on the line is 100. Try some other inputs as well. Now try a line that contains both integers and other values, e.g., We have 2 dogs and 1 cat.
2. ParseInts
File ParseInts.java contains a program that does the following:
Prompts for and reads in a line of input
Uses a second Scanner to take the input line one token at a time and parses an integer from each token as it is extracted.
Sums the integers.
Prints the sum.
Save ParseInts to your directory and compile and run it. If you give it the input
10 20 30 40
it should print
The sum of the integers on the line is 100.
Try some other inputs as well. Now try a line that contains both integers and other values, e.g.,
We have 2 dogs and 1 cat.
You should get a NumberFormatException when it tries to call Integer.parseInt on “We”, which is not an integer. One way around this is to put the loop that reads inside a try and catch the NumberFormatException but not do anything with it. This way if it’s not an integer it doesn’t cause an error; it goes to the exception handler, which does nothing. Do this as follows:
Modify the program to add a try statement that encompasses the entire while loop. The try and opening { should go before the while, and the catch after the loop body. Catch a NumberFormatException and have an empty body for the catch.
Compile and run the program and enter a line with mixed integers and other values. You should find that it stops summing at the first non-integer, so the line above will produce a sum of 0, and the line “1 fish 2 fish” will produce a sum of 1. This is because the entire loop is inside the try, so when an exception is thrown the loop is terminated. To make it continue, move the try and catch inside the loop. Now when an exception is thrown, the next statement is the next iteration of the loop, so the entire line is processed. The dogs-and-cats input should now give a sum of 3, as should the fish input.
// ****************************************************************
// ParseInts.java
//
// Reads a line of text and prints the integers in the line.
//
// **************************************************************** import java.util.Scanner;
public class ParseInts
{
public static void main(String[] args)
{
int val, sum=0;
Scanner scan = new Scanner(System.in); String line;
System.out.println("Enter a line of text");
Scanner scanLine = new Scanner(scan.nextLine());
while (scanLine.hasNext())
{
val = Integer.parseInt(scanLine.next());
sum += val;
}
System.out.println("The sum of the integers on this line is " + sum);
}

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 2 images

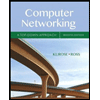
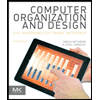
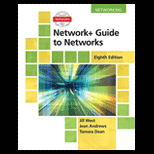
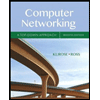
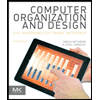
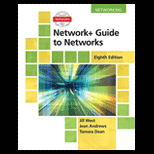
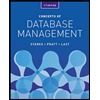
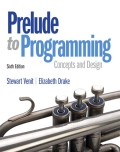
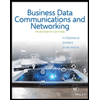