Extend the below program such that when reading the 3 values per record from the file, the values get stored in arrays. We need an array for each data type read per record ( loop counter to store corresponding values at the same index in the 3 different arrays). We need a two dimensional char array. when copying a string to another, we need to use the strncpy function. Once all the data from the input file is read and stored this data into the 3 arrays, open a file for writing ( output.csv) and print the array data to this file (use fprintf). The format written to the file should be one line per record, with each field separated by a comma (‘,’). Close both files. #include char c; int main (int argc, char **argv) { FILE* f; if (argc != 2) { printf("No filename in the argument"); return 1; } f = fopen(argv[1], "r"); if (f == NULL) { printf("The file cannot be opened successfully: %s\n",argv[1]); return 1; } printf("The file has been opened successfully\n\n"); int lines = 0; while( c != EOF) { c = fgetc(f); if(c == '\n') { lines++; } if(c ==EOF) { lines++; } else { printf("%c",c); } } printf("\n\nFile has Total %d Lines.",lines) ; fclose(f); return 0; }
Extend the below program such that when reading the 3 values per record from the file, the values get stored in arrays. We need an array for each data type read per record ( loop counter to store corresponding values at the same index in the 3 different arrays). We need a two dimensional char array. when copying a string to another, we need to use the strncpy function.
Once all the data from the input file is read and stored this data into the 3 arrays, open a file for writing ( output.csv) and print the array data to this file (use fprintf). The format written to the file should be one line per record, with each field separated by a comma (‘,’). Close both files.
#include<stdio.h>
char c;
int main (int argc, char **argv)
{
FILE* f;
if (argc != 2)
{
printf("No filename in the argument");
return 1;
}
f = fopen(argv[1], "r");
if (f == NULL) {
printf("The file cannot be opened successfully: %s\n",argv[1]);
return 1;
}
printf("The file has been opened successfully\n\n");
int lines = 0;
while( c != EOF)
{
c = fgetc(f);
if(c == '\n')
{
lines++;
}
if(c ==EOF)
{
lines++;
}
else
{
printf("%c",c);
}
}
printf("\n\nFile has Total %d Lines.",lines) ;
fclose(f);
return 0;
}

Step by step
Solved in 3 steps with 3 images

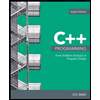
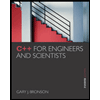
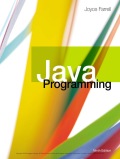
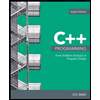
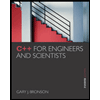
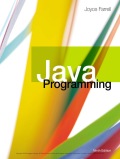
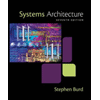
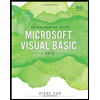