Explain through each question about Classes and Objects in Java. What is encapsulations and why is it useful? What is an instance method and how does it differ from the static methods we learned previously? What is a mutator method? What is an accessor method? What is a constructor in a Java class? What is an implicit parameter?
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
Explain through each question about Classes and Objects in Java.
- What is encapsulations and why is it useful?
- What is an instance method and how does it differ from the static methods we learned previously?
- What is a mutator method? What is an accessor method?
- What is a constructor in a Java class?
- What is an implicit parameter?
Please provide an entire coding example to illustrate the answer.

Encapsulation- Encapsulation is a process of wrapping up of data and code into a single unit. An encapsulated class in java can be created by making all the data members in the class private. And then, getter and setter methods can be used to retrieve and update data.
Why Encapsulation is useful?
- The whole idea behind Encapsulation is to hide the details from the users. If the data member is private, it means it can only be accessed in the same class and no other classes can access that private data member.
- Encapsulation provides control over the data. For example, if we want to set a value of marks greater than 80 which would only show students who obtained marks greater than 80, we can write the logic in the setter method.
- The encapsulated class is easy to test. So, it can be used for unit testing.
Code Of Encapsulation in Java-
File: Teacher.java
public class Teacher
{
private String name;
public void setName(String name)
{
this.name=name;
}
public String getName()
{
return name;
}
}
File: Main.java
class Main
{
public static void main(String []args)
{
Teacher t=new Teacher();
t.setName("Mr. Smith");
System.out.println(t.getName());
}
}
Trending now
This is a popular solution!
Step by step
Solved in 5 steps with 3 images

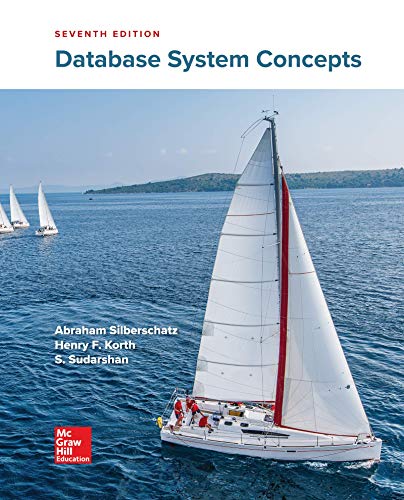
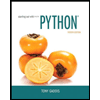
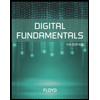
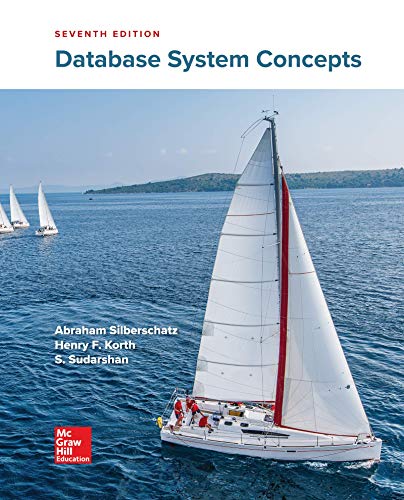
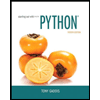
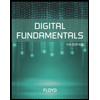
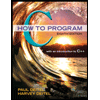
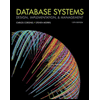
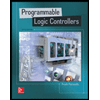