Explain the working of this code #include #include using namespace std; class Pancake { private: int top; int arr[10]; public: Pancake() { top = -1; for (int i = 0; i < 5; i++) { arr[i] = 0; } } bool isEmpty() { if (top == -1) return true; else return false; } bool isFull() { if (top == 4) return true; else return false; } void push(int val) { if (isFull()) { cout << "pancakes overflow" << endl; } else { top++; // 1 arr[top] = val; } } int pop() { if (isEmpty()) { cout << "pancakes underflow" << endl; return 0; } else { int popValue = arr[top]; arr[top] = 0; top--; return popValue; } } int count() { return (top + 1); } int peek(int pos) { if (isEmpty()) { cout << "pancake underflow" << endl; return 0; } else { return arr[pos]; } } void display() { cout << "All values in the pancake are " << endl; for (int i = 4; i >= 0; i--) { cout << arr[i] << endl; } } }; int main() { Pancake s1; int option, postion, value; do { cout << "What operation do you want to perform? Select Option number. Enter 0 to exit." << endl; cout << "1. Push()" << endl; cout << "2. Pop()" << endl; cout << "3. isEmpty()" << endl; cout << "4. isFull()" << endl; cout << "5. peek()" << endl; cout << "6. count()" << endl; cout << "7. display()" << endl; cout << "8. Clear Screen" << endl << endl; cin >> option; switch (option) { case 0: break; case 1: cout << "Enter an item to push in the Pancake" << endl; cin >> value; s1.push(value); break; case 2: cout << "Pop Function Called - Poped Value: " << s1.pop() << endl; break; case 3: if (s1.isEmpty()) cout << "Pancake is Empty" << endl; else cout << "Pancake is not Empty" << endl; break; case 4: if (s1.isFull()) cout << "Pancake is Full" << endl; else cout << "Pancake is not Full" << endl; break; case 5: cout << "Enter position of item you want to peek: " << endl; cin >> postion; cout << "Peek Function Called - Value at position " << postion << " is " << s1.peek(postion) << endl; break; case 6: cout << "Count Function Called - Number of Items in the Pancake are: " << s1.count() << endl; break; case 7: cout << "Display Function Called - " << endl; s1.display(); break; case 8: system("cls"); break; default: cout << "Enter Proper Option number " << endl; } } while (option != 0); return 0; }
Explain the working of this code
#include<iostream>
#include<string>
using namespace std;
class Pancake {
private:
int top;
int arr[10];
public:
Pancake() {
top = -1;
for (int i = 0; i < 5; i++) {
arr[i] = 0;
}
}
bool isEmpty() {
if (top == -1)
return true;
else
return false;
}
bool isFull() {
if (top == 4)
return true;
else
return false;
}
void push(int val) {
if (isFull()) {
cout << "pancakes overflow" << endl;
} else {
top++; // 1
arr[top] = val;
}
}
int pop() {
if (isEmpty()) {
cout << "pancakes underflow" << endl;
return 0;
} else {
int popValue = arr[top];
arr[top] = 0;
top--;
return popValue;
}
}
int count() {
return (top + 1);
}
int peek(int pos) {
if (isEmpty()) {
cout << "pancake underflow" << endl;
return 0;
} else {
return arr[pos];
}
}
void display() {
cout << "All values in the pancake are " << endl;
for (int i = 4; i >= 0; i--) {
cout << arr[i] << endl;
}
}
};
int main() {
Pancake s1;
int option, postion, value;
do {
cout << "What operation do you want to perform? Select Option number. Enter 0 to exit." << endl;
cout << "1. Push()" << endl;
cout << "2. Pop()" << endl;
cout << "3. isEmpty()" << endl;
cout << "4. isFull()" << endl;
cout << "5. peek()" << endl;
cout << "6. count()" << endl;
cout << "7. display()" << endl;
cout << "8. Clear Screen" << endl << endl;
cin >> option;
switch (option) {
case 0:
break;
case 1:
cout << "Enter an item to push in the Pancake" << endl;
cin >> value;
s1.push(value);
break;
case 2:
cout << "Pop Function Called - Poped Value: " << s1.pop() << endl;
break;
case 3:
if (s1.isEmpty())
cout << "Pancake is Empty" << endl;
else
cout << "Pancake is not Empty" << endl;
break;
case 4:
if (s1.isFull())
cout << "Pancake is Full" << endl;
else
cout << "Pancake is not Full" << endl;
break;
case 5:
cout << "Enter position of item you want to peek: " << endl;
cin >> postion;
cout << "Peek Function Called - Value at position " << postion << " is " << s1.peek(postion) << endl;
break;
case 6:
cout << "Count Function Called - Number of Items in the Pancake are: " << s1.count() << endl;
break;
case 7:
cout << "Display Function Called - " << endl;
s1.display();
break;
case 8:
system("cls");
break;
default:
cout << "Enter Proper Option number " << endl;
}
} while (option != 0);
return 0;
}

Step by step
Solved in 5 steps

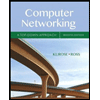
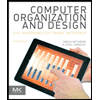
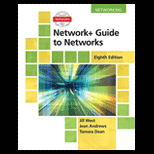
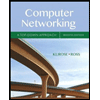
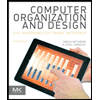
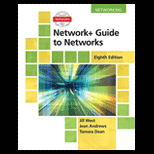
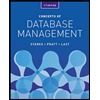
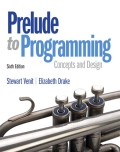
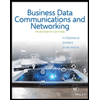