Explain in detail what the following code is doing: import sys """ This functions build the title-actors dictionary. The keys are movie titles, and the values are the cast lists of each movie.""" def make_title_dict(): titleActorDict = {} # create an empty dict (movie title -> key, list of actor(s) ) with open("imdb.txt", "r") as fHandle: # open file for reading lines = fHandle.readlines() # list of lines from the input file for index in range (0, len(lines), 2): title = lines[index].strip().lower() # movie name -> key actor = lines[index+1].strip().lower() # actor name -> value if title in titleActorDict: # already in Dict titleActorDict[title].append(actor) else : # its not in the Dict yet titleActorDict[title] = [actor] # list of actors, add that one actor to begin with return titleActorDict """ Define the following functions to return the requested result """ def getNumFilms(titleActorDict): res = list(titleActorDict.keys()) #listing out the data return len(res) #returning length of list for number of films def getLongestCastList(titleActorDict): keys = list(titleActorDict.keys()) #listing out the data maxCastNum = 0 #setting the maxCastNum for key in keys: #creating a loop to iterate over the list if(maxCastNum < len(titleActorDict[key])): maxCastNum = len(titleActorDict[key]) return maxCastNum #returning the longest cast list def getShortestCastList(titleActorDict): keys = list(titleActorDict.keys()) minCastNum = 9999999 #setting the minCastNum for key in keys: if (minCastNum > len(titleActorDict[key])): minCastNum = len(titleActorDict[key]) #doing something similar when getting the Longest cast list return minCastNum def lookupActor(titleActorDict, movie, actor): if(actor in titleActorDict[movie]): #checks if actor is within list return True else: return False def getCommonActors(titleActorDict, movie1, movie2): resList = [] actor_list1 = titleActorDict[movie1] actor_list2 = titleActorDict[movie2] for actor in actor_list1: if(actor in actor_list2): resList.append(actor) return resList def getCommonActors_v2(titleActorDict, movie1, movie2, movie3): if movie1 in titleActorDict and movie2 in titleActorDict and movie3 in titleActorDict: actor_list1 = titleActorDict[movie1] actor_list2 = titleActorDict[movie2] actor_list3 = titleActorDict[movie3] common_actors = list(set(actor_list1) & set(actor_list2) & set(actor_list3)) return common_actors return [] def getActorMovieList(titleActorDict, actor): movies = [movie for movie, cast_list in titleActorDict.items() if actor in cast_list] return movies titleActorDict = make_title_dict()
Explain in detail what the following code is doing:
import sys
""" This functions build the title-actors dictionary.
The keys are movie titles, and the values are the cast lists of each movie."""
def make_title_dict():
titleActorDict = {} # create an empty dict (movie title -> key, list of actor(s) )
with open("imdb.txt", "r") as fHandle: # open file for reading
lines = fHandle.readlines() # list of lines from the input file
for index in range (0, len(lines), 2):
title = lines[index].strip().lower() # movie name -> key
actor = lines[index+1].strip().lower() # actor name -> value
if title in titleActorDict: # already in Dict
titleActorDict[title].append(actor)
else : # its not in the Dict yet
titleActorDict[title] = [actor] # list of actors, add that one actor to begin with
return titleActorDict
""" Define the following functions to return the requested result """
def getNumFilms(titleActorDict):
res = list(titleActorDict.keys()) #listing out the data
return len(res) #returning length of list for number of films
def getLongestCastList(titleActorDict):
keys = list(titleActorDict.keys()) #listing out the data
maxCastNum = 0 #setting the maxCastNum
for key in keys: #creating a loop to iterate over the list
if(maxCastNum < len(titleActorDict[key])):
maxCastNum = len(titleActorDict[key])
return maxCastNum #returning the longest cast list
def getShortestCastList(titleActorDict):
keys = list(titleActorDict.keys())
minCastNum = 9999999 #setting the minCastNum
for key in keys:
if (minCastNum > len(titleActorDict[key])):
minCastNum = len(titleActorDict[key]) #doing something similar when getting the Longest cast list
return minCastNum
def lookupActor(titleActorDict, movie, actor):
if(actor in titleActorDict[movie]): #checks if actor is within list
return True
else:
return False
def getCommonActors(titleActorDict, movie1, movie2):
resList = []
actor_list1 = titleActorDict[movie1]
actor_list2 = titleActorDict[movie2]
for actor in actor_list1:
if(actor in actor_list2):
resList.append(actor)
return resList
def getCommonActors_v2(titleActorDict, movie1, movie2, movie3):
if movie1 in titleActorDict and movie2 in titleActorDict and movie3 in titleActorDict:
actor_list1 = titleActorDict[movie1]
actor_list2 = titleActorDict[movie2]
actor_list3 = titleActorDict[movie3]
common_actors = list(set(actor_list1) & set(actor_list2) & set(actor_list3))
return common_actors
return []
def getActorMovieList(titleActorDict, actor):
movies = [movie for movie, cast_list in titleActorDict.items() if actor in cast_list]
return movies
titleActorDict = make_title_dict()

Trending now
This is a popular solution!
Step by step
Solved in 3 steps

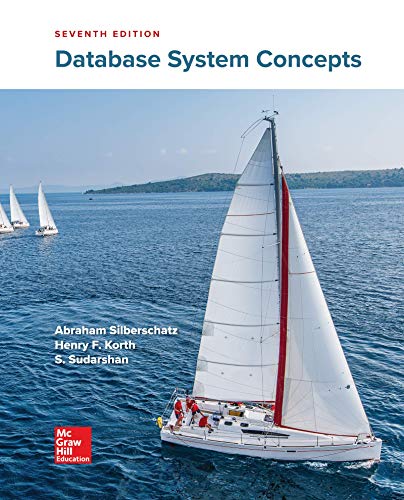
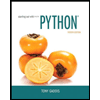
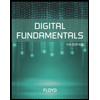
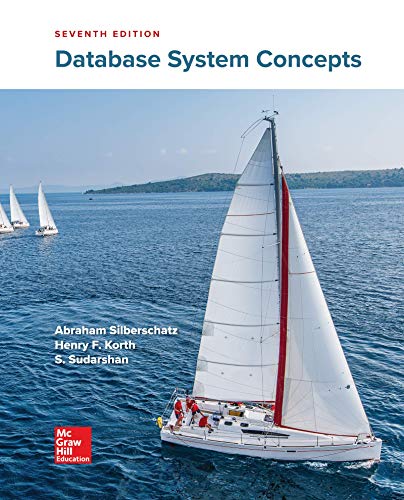
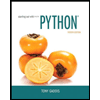
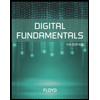
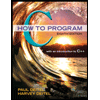
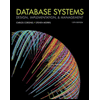
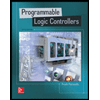