Exercise GradesStatistics (Method): Write a program called GradesStatistics, which reads in n grades (of int between 0 and 100, inclusive) and displays the average, minimum, maximum, median and standard deviation. Display the floating-point values upto 2 decimal places. Your output shall look like: Enter the number of students: 4 Enter the grade for student 1: 50 Enter the grade for student 2: 51 Enter the grade for student 3: 56 Enter the grade for student 4: 53 {50,51,56,53} The average is: 52.50 The median is: 52.00 The minimum is: 50 The maximum is: 56 The standard deviation is: 2.29 The formula for calculating standard deviation is: Hints: public class GradesStatistics { public static int[] grades; // Declare an int[], to be allocated later. // This array is accessible by all the methods. public static void main(String[] args) { readGrades(); // Read and save the inputs in int[] grades printArray(grades); System.out.println("The average is " + average(grades)); System.out.println("The median is " + median(grades)); System.out.println("The minimum is " + min(grades)); System.out.println("The maximum is " + max(grades)); System.out.println("The standard deviation is " + stdDev(grades)); } // Prompt user for the number of students and allocate the global "grades" array. // Then, prompt user for grade, check for valid grade, and store in "grades". public static void readGrades() { ....... } // Print the given int array in the form of {x1, x2, x3,..., xn}. public static void printArray(int[] array) { ....... } // Return the average value of the given int[] public static double average(int[] array) { ...... } // Return the median value of the given int[] // Median is the center element for odd-number array, // or average of the two center elements for even-number array. // Use Arrays.sort(anArray) to sort anArray in place. public static double median(int[] array) { ...... } // Return the maximum value of the given int[] public static int max(int[] array) { int max = array[0]; // Assume that max is the first element // From second element, if the element is more than max, set the max to this element. ...... } // Return the minimum value of the given int[] public static int min(int[] array) { ....... } // Return the standard deviation of the given int[] public static double stdDev(int[] array) { ....... } } Take note that besides readGrade() that relies on global variable grades, all the methods are self-contained general utilities that operate on any given array.
- Exercise GradesStatistics (Method): Write a
program called GradesStatistics, which reads in n grades (of int between 0 and 100, inclusive) and displays the average, minimum, maximum, median and standard deviation. Display the floating-point values upto 2 decimal places. Your output shall look like:
Enter the number of students: 4
Enter the grade for student 1: 50
Enter the grade for student 2: 51
Enter the grade for student 3: 56
Enter the grade for student 4: 53
{50,51,56,53}
The average is: 52.50
The median is: 52.00
The minimum is: 50
The maximum is: 56
The standard deviation is: 2.29
The formula for calculating standard deviation is:
Hints:
public class GradesStatistics {
public static int[] grades; // Declare an int[], to be allocated later.
// This array is accessible by all the methods.
public static void main(String[] args) {
readGrades(); // Read and save the inputs in int[] grades
printArray(grades);
System.out.println("The average is " + average(grades));
System.out.println("The median is " + median(grades));
System.out.println("The minimum is " + min(grades));
System.out.println("The maximum is " + max(grades));
System.out.println("The standard deviation is " + stdDev(grades));
}
// Prompt user for the number of students and allocate the global "grades" array.
// Then, prompt user for grade, check for valid grade, and store in "grades".
public static void readGrades() { ....... }
// Print the given int array in the form of {x1, x2, x3,..., xn}.
public static void printArray(int[] array) { ....... }
// Return the average value of the given int[]
public static double average(int[] array) { ...... }
// Return the median value of the given int[]
// Median is the center element for odd-number array,
// or average of the two center elements for even-number array.
// Use Arrays.sort(anArray) to sort anArray in place.
public static double median(int[] array) { ...... }
// Return the maximum value of the given int[]
public static int max(int[] array) {
int max = array[0]; // Assume that max is the first element
// From second element, if the element is more than max, set the max to this element.
......
}
// Return the minimum value of the given int[]
public static int min(int[] array) { ....... }
// Return the standard deviation of the given int[]
public static double stdDev(int[] array) { ....... }
}
Take note that besides readGrade() that relies on global variable grades, all the methods are self-contained general utilities that operate on any given array.
- Exercise GradesHistogram (Method): Write a program called GradesHistogram, which reads in n grades (as in the previous exercise), and displays the horizontal and vertical histograms. For example:
0 - 9: ***
10 - 19: ***
20 - 29:
30 - 39:
40 - 49: *
50 - 59: *****
60 - 69:
70 - 79:
80 - 89: *
90 -100: **
*
*
* * *
* * * *
* * * * * *
0-9 10-19 20-29 30-39 40-49 50-59 60-69 70-79 80-89 90-100
Hints:
- Declare a 10-element int arrays called bins, to maintain the counts for [0,9], [10,19], ..., [90,100]. Take note that the bins's index is mark/10, except mark of 100.
- Write the codes to print the star first. Test it. Then print the labels.
- To print the horizontal histogram, use a nested loop:
- for (int binNum = 0; binNum < bins.length; binNum++) { // each row for one bin
- // Print label for each row
- .......
- for (int starNum = 0; starNum < bins[binNum]; starNum++) { // each column is one star
- .......
- }
- ...... // print newline
}
- To print the vertical histogram, you need to find the maximum bin count (called maxBinCount) and use a nested loop:
- for (int level = maxBinCount; level >= 1; level--) { // each row for one count level
- for (int binNum = 0; binNum < bins.length; binNum++) { // each column for one bin
- // if (bin's count >= level) print *
- // else print blank.
- }
- ...... // print newline
}
- Exercise (Array and Method): Write a program called PrintChart that prompts the user to input n non-negative integers and draws the corresponding horizontal bar chart. Your program shall use an int array of length n; and comprise methods readInput() and printChart(). A sample session is as follows:
Enter number of bars: 4
Enter bar 1 value: 4
Enter bar 2 value: 3
Enter bar 3 value: 5
Enter bar 4 value: 7
**** (4)
*** (3)
***** (5)
******* (7)

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

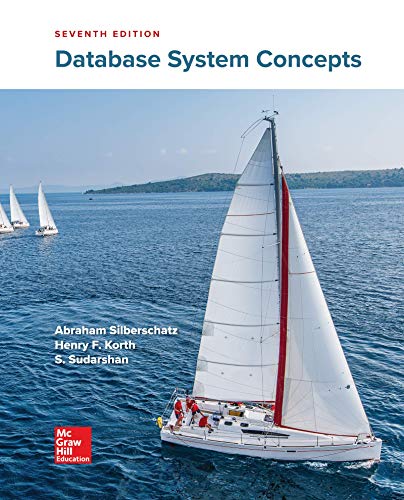
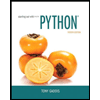
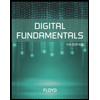
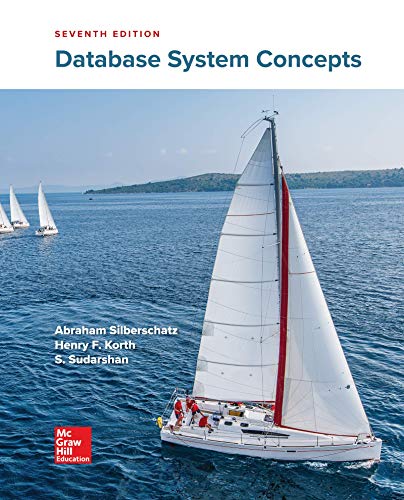
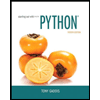
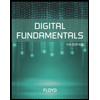
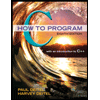
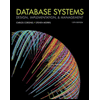
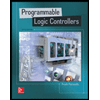